Discuss Scratch
- Discussion Forums
- » Advanced Topics
- » js or python
- imfh
-
1000+ posts
js or python
Some more weirdness with string references. Notice how the id changes whenever you modify it. This shows that a new string is being made behind the scenes, even if you use the += operator.
>>> a = "hi" >>> id(a) 139918267500592 >>> a += " there" >>> id(a) 139918269043632 >>> b = "hello" >>> id(b) 139918269051248 >>> id("hello") 139918269051248
Scratch to Pygame converter: https://scratch.mit.edu/discuss/topic/600562/
- davidtheplatform
-
500+ posts
js or python
This also applies to small integers, anything less than 256 IIRC.FYI,My mistake (I'm not deeply familiar with Python), although given all python vars are references then why does this happen? ~Snip~This means that strings are immutable while lists and dictionaries are mutable, but they're both passed in as refs. A string is a reference, but mostly acts as a primitive?>>> x = "hi" ... y = "hi" >>> id(x) 4414027184 >>> id(y) 4414027184 >>> def z(a): ... a += "hhhh" ... z(x) ... x 'hi'does not mutate the variable, it just reassigns the value of a. Oh by the way, JavaScript also acts the same waya += "hhhh" # shorthand for a = a + "hhhh"And Java while we're at itlet a = "a" function addhhhh(b) { b += "hhhh" } addhhhh(a) console.log(a) // aclass Main { public static void addhhhh(String b) { b += "hhhh"; } public static void main(String[] args) { String a = "a"; addhhhh(a); System.out.println(a); // a } }That's most likely for optimization purposes - when would you need to compare memory locations for two equivalent strings anyways? Also creating two separate strings assigns them the same reference if they are equivalent meaning that == and ‘is’ comparisons return the same, meaning that for almost every purpose a string acts as a js-like primitive, but isn't.
Generation 4: the first time you see this copy and paste it on top of your sig in the scratch forums and increase generation by 1. Social experiment.
- AHypnoman
-
1000+ posts
js or python
That's what I said. does not mutate the variable
The difference is that in JS primitives are all immutable, and, unless specified when creating one (excluding Web APIs, as they aren't a core part of JS), objects are mutable. Oh by the way, JavaScript also acts the same way...
Java and JS are entirely distinct. One is used on nearly every website and the other can be found at archaeological sites. And Java while we're at itclass Main { public static void addhhhh(String b) { b += "hhhh"; } public static void main(String[] args) { String a = "a"; addhhhh(a); System.out.println(a); // a } }
The reason for such behaviour is irrelevant (although I doubt this is for speed - doing this means that Python will need to utilise more memory to store a new reference which is almost certainly a slow process). There are a few reasons you'd want to compare memory locations (such as manual memory management if you have limited resources), although my main problem with it is the inconsistency in behaviour - not lack of capability.That's most likely for optimization purposes - when would you need to compare memory locations for two equivalent strings anyways? Also creating two separate strings assigns them the same reference if they are equivalent meaning that == and ‘is’ comparisons return the same, meaning that for almost every purpose a string acts as a js-like primitive, but isn't.
~ AHypnoman (Use Shift+Down Arrow to see the rest of my signature)
I am an evil kumquat, and your siggy looks tasty…
Hello there, I'm AHypnoman, If you write my name remember to capitalize the “H”. “When you don't create things, you become defined by your tastes rather than ability. your tastes only narrow & exclude people. so create.” I write though as tho.
According to the Chrome Console, my viewbox attribute is “Garbage”. Very nice, Chrome.
#TrendyHashtag
Gib internneeet
Play Retorquebit!
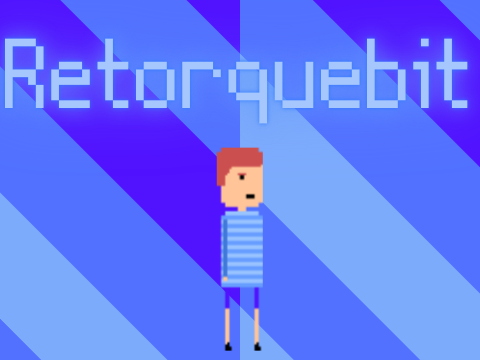
- ajskateboarder
-
1000+ posts
js or python
I'm including a Java sample here because both Java and Python make strings an immutable type, not because JavaScript and Java are supposedly similar (with the exception of standard libraries like Math looking practically the same)Java and JS are entirely distinct. One is used on nearly every website and the other can be found at archaeological sites. Oh by the way, JavaScript also acts the same way...
The difference is that in JS primitives are all immutable, and, unless specified when creating one (excluding Web APIs, as they aren't a core part of JS), objects are mutable.Yes. This is also true for Python as well. Any hashable built-in object like a str, int, float are immutable and can't be modified through a function parameter, while mutable containers (which can't be hashed) like dict and list can be
The reason for such behaviour is irrelevant (although I doubt this is for speed - doing this means that Python will need to utilise more memory to store a new reference which is almost certainly a slow process).I was moreso thinking of optimization as in avoiding excess memory allocation, not speed (even then this is a strange decision, considering the amount of memory in the everyday system). Anyways
There are a few reasons you'd want to compare memory locations (such as manual memory management if you have limited resources), although my main problem with it is the inconsistency in behaviour - not lack of capability.Notice I said comparing the memory locations of constant equivalent strings. What is this inconsistent with, really? Also, meticulous memory management can be much better done through C and Cython. Python isn't quite good with controlled memory management
Last edited by ajskateboarder (April 4, 2024 16:49:22)
- AHypnoman
-
1000+ posts
js or python
My point was that in JS default mutability/immutability correlates to being an object or primitive, whilst in Python there is no such correlation. Yes. This is also true for Python as well. Any hashable built-in object like a str, int, float are immutable and can't be modified through a function parameter, while mutable containers (which can't be hashed) like dict and list can be
constant equivalent strings. What is this inconsistent with, really?(You did not specify that they were constants in your original post). The inconsistency I refer to is the differing mutability between types of object - strings and lists, for example, are both objects. List are mutable, while strings are immutable. Primitives are inherently immutable, so it makes sense that they are immutable in JS. Reference variables on the other hand are inherently mutable, so it makes sense that they are by default in a language (They are plenty of reasons a program may want to change their mutability, but defaults should be mutable since they are internally). In Python, everything is an object+reference and some objects are immutable. There is no reason to have this behaviour, especially since it introduces inconistencies. Notice I said comparing the memory locations of
This behaviour isn't uncommon in programming languages, although that certainly doesn't make it any better.
Whether Python is best suited to it or not, it must be a consideration. Most AI software is written in Python now and memory management is incredibly important to that (granted, anyone writing AI in Python chose the wrong language for the job: languages like C and Rust are far faster and better for memory management so are better suited for AI). Also, meticulous memory management can be much better done through C and Cython. Python isn't quite good with controlled memory management
I don't mean to say JS is a perfect language, or that Python is a particularly bad one. I'm just providing reasoning for my preference.
(JS has weird inconsistencies too, e.g. parseFloat is a top-level function while .toString is on prototype?? IK there's String, but that can be weird).
Last edited by AHypnoman (April 4, 2024 17:58:02)
~ AHypnoman (Use Shift+Down Arrow to see the rest of my signature)
I am an evil kumquat, and your siggy looks tasty…
Hello there, I'm AHypnoman, If you write my name remember to capitalize the “H”. “When you don't create things, you become defined by your tastes rather than ability. your tastes only narrow & exclude people. so create.” I write though as tho.
According to the Chrome Console, my viewbox attribute is “Garbage”. Very nice, Chrome.
#TrendyHashtag
Gib internneeet
Play Retorquebit!
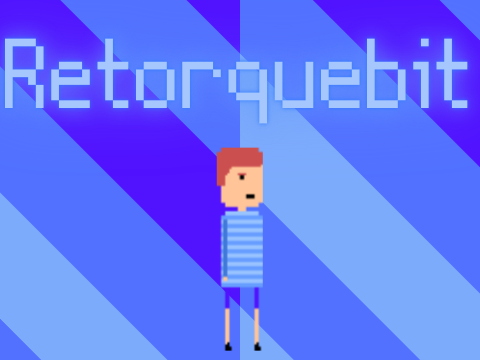
- ajskateboarder
-
1000+ posts
js or python
Great point, it might be consistent with other languages, but it's not consistent itself. It's just like Java exceptions, they are popular in other languages but somehow act as some escape hatch for control-flow (Result enums > go if err != nil > exceptions). Seems like all the bad programming concepts stem from Java The inconsistency I refer to is the differing mutability between types of object - strings and lists, for example, are both objects. List are mutable, while strings are immutable. Primitives are inherently immutable, so it makes sense that they are immutable in JS. Reference variables on the other hand are inherently mutable, so it makes sense that they are by default in a language (They are plenty of reasons a program may want to change their mutability, but defaults should be mutable since they are internally). In Python, everything is an object+reference and some objects are immutable. There is no reason to have this behaviour, especially since it introduces inconistencies.
This behaviour isn't uncommon in programming languages, although that certainly doesn't make it any better.
I don't mean to say JS is a perfect language, or that Python is a particularly bad one. I'm just providing reasoning for my preference.Of course

A lot of ML libraries like TensorFlow or PyTorch (as well as numpy and scipy for statistical calculations) are primarily written with C++ and Cython, so a lot of overhead just disappears. C and Rust aren't exactly the best languages to teach data scientists/engineers due to:Whether Python is best suited to it or not, it must be a consideration. Most AI software is written in Python now and memory management is incredibly important to that (granted, anyone writing AI in Python chose the wrong language for the job: languages like C and Rust are far faster and better for memory management so are better suited for AI). Also, meticulous memory management can be much better done through C and Cython. Python isn't quite good with controlled memory management
- how specialized their role is anyways
- how much of the ML ecosystem already uses Python
- Python being good for rapid development, which is primarily what data science involves (source: see the amount of jupyter notebooks that people make)
That being said, Rust does have a TensorFlow binding
JavaScript also has a port of TensorFlow, but configuring web workers to load 500mb chunks of model checkpoints into the browser is a headache, and the Node API is just… weird
API design is just kind of strange with Node. It's super fragmented, and only the most updated of libraries will work, even though Node has been supposedly stable for years now. There's also like 4 competing package managers (which mostly will not cooperate with each other) and a couple of alternative runtimes (most of which thankfully aren't being pervasively adopted)
Last edited by ajskateboarder (April 4, 2024 19:45:24)
- AHypnoman
-
1000+ posts
js or python
Yes - that's something I did't consider. Given the existent support for AI that Python has, it's probably best for AI at this point. I was referencing the speed benefits and requirements rather than infrastructure and syntactic suitability (which admittedly I overlooked entirely). A lot of ML libraries like TensorFlow or PyTorch (as well as numpy and scipy for statistical calculations) are primarily written with C++ and Cython, so a lot of overhead just disappears. C and Rust aren't exactly the best languages to teach data scientists/engineers due to:
- how specialized their role is anyways
- how much of the ML ecosystem already uses Python
- Python being good for rapid development, which is primarily what data science involves (source: see the amount of jupyter notebooks that people make)
That being said, Rust does have a TensorFlow binding
JavaScript also has a port of TensorFlow, but configuring web workers to load 500mb chunks of model checkpoints into the browser is a headache, and the Node API is just… weird
Getting Node working can be a pain, although I rarely encounter problems with packages breaking - aside from a small few I've never really had a problem installing older and unmaintained packages. There are a handful of package managers although NPM is by far the most used and has far more content than PNPM, Yarn, etc. Python does have some alternatives to PIP like Anaconda, although AFAIK they aren't nearly big enough to be a real competitor. API design is just kind of strange with Node. It's super fragmented, and only the most updated of libraries will work, even though Node has been supposedly stable for years now. There's also like 4 competing package managers (which mostly will not cooperate with each other) and a couple of alternative runtimes (most of which thankfully aren't being pervasively adopted)
As for alternative runtimes - I agree entirely. It would be far better to have one universally agreed-upon runtime. Some runtimes do have huge advantages over others, granted (e.g. Bun using internal multithreading to run far faster than Node and having a centrally distributed package manager), but decentralisation means that despite many programmes being written in the same language they are entirely incompatible with one another.
Last edited by AHypnoman (April 4, 2024 21:21:45)
~ AHypnoman (Use Shift+Down Arrow to see the rest of my signature)
I am an evil kumquat, and your siggy looks tasty…
Hello there, I'm AHypnoman, If you write my name remember to capitalize the “H”. “When you don't create things, you become defined by your tastes rather than ability. your tastes only narrow & exclude people. so create.” I write though as tho.
According to the Chrome Console, my viewbox attribute is “Garbage”. Very nice, Chrome.
#TrendyHashtag
Gib internneeet
Play Retorquebit!
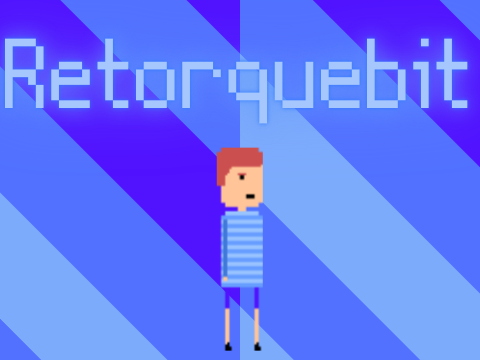
- BigNate469
-
1000+ posts
js or python
You do have to keep in mind that newer processors have cores specifically designed to run (or, at least speed up) AI, so whatever language works best for those processors can greatly improve performance on them, even if Python has more support in general.Yes - that's something I did't consider. Given the existent support for AI that Python has, it's probably best for AI at this point. I was referencing the speed benefits and requirements rather than infrastructure and syntactic suitability (which admittedly I overlooked entirely). A lot of ML libraries like TensorFlow or PyTorch (as well as numpy and scipy for statistical calculations) are primarily written with C++ and Cython, so a lot of overhead just disappears. C and Rust aren't exactly the best languages to teach data scientists/engineers due to:
- how specialized their role is anyways
- how much of the ML ecosystem already uses Python
- Python being good for rapid development, which is primarily what data science involves (source: see the amount of jupyter notebooks that people make)
That being said, Rust does have a TensorFlow binding
JavaScript also has a port of TensorFlow, but configuring web workers to load 500mb chunks of model checkpoints into the browser is a headache, and the Node API is just… weird
…
Highlight any part of this signature and press ctrl+shift+down arrow to see the rest of it.
For information on a signiture, including how to make one, visit here.
Please read the list of Officially Rejected Suggestions before posting a suggestion for Scratch!
View all of the topics you've posted in:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=topics
View all of your posts:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=posts
Lesser-known Scratch URLs:
https://scratch.mit.edu/projects/PROJECT ID HERE/remixtree (replace “PROJECT ID HERE” with project id number. Shows all the remixes of the project, and the remixes of those projects, and the remixes of those projects, and so on, as a chart. Link currently redirects to one of my projects). There was a button on the project page linking to this in 2.0, but it was removed.
View a larger list at: https://scratch.mit.edu/discuss/topic/542480/
Why @Paddle2See's responses are so often identical: https://scratch.mit.edu/discuss/topic/762351/
1000th post
The devs really need to update the scratch-gui repo- I'm getting at least 19 “npm warn deprecated” warnings whenever I try to install its dependencies, and yes, I'm using the latest version of node.js and npm. Then again, the dev page on this website and the wiki in the repo still refer to Scratch 3.0 in beta, and link the current website as an example of 2.0.
- ajskateboarder
-
1000+ posts
js or python
A little pet peeve that is sort of confusing me:
But this does work
Here's basically the code that is causing the same confusion
I'm guessing it won't since I assign the handler to the element before placing it in the DOM, but yeah ¯\_(ツ)_/¯
function a(callback) { callback(); return 12 } let b = a(() => (b = 13)); console.log(b); // index.js:5 // let a = thing(() => {a = 11}) // ^ // ReferenceError: Cannot access 'a' before initialization
function a(callback) { setTimeout(callback, 0.001); // timeout ms is arbitrary, setting anything will make it work return 12 } let b = a(() => (b = 13)); console.log(b); // 12 // this means the variable set happened in the callback
/** @type {HTMLButtonElement} /* let button = Button({ // will this error or not?? onmouseover: () => { button.style.scale = "0.9"; }, });
- ajskateboarder
-
1000+ posts
js or python
// destructuring without an array? var thing = (1, 2, 44, "a"); console.log(thing) // "a" var thing, _, __, ___ = (1, 2, 44, "a"); // expects to print 1 console.log(thing); // undefined var (thing, _, __, ___) = (1, 2, 44, "a"); // ReferenceError: let is not defined
- mybearworld
-
1000+ posts
js or python
I don't really understand your question, so I'll just explain what's going on in these lines of code
// JS has the comma operator. This takes in two values and discards the first one, only returning // the second one. So: console.log((1, 2) === 2) // true // Here, (1, 2, 44, "a") gets evaluated to "a", because the comma operators discard everything // to the left. thing gets set to that value. var thing = (1, 2, 44, "a"); console.log(thing) // "a" // JS allows multiple variable declarations in a single statement. If you run this line through a // formatter, you'll get: // var thing, // _, // __, // ___ = (1, 2, 44, "a"); // So, this declares "thing", "_" and "__" without assigning them a value (so they default to // undefined), and ___ as "a". var thing, _, __, ___ = (1, 2, 44, "a"); console.log(thing); // undefined // The parentheses would make the code look incorrect here, so they're not allowed. var (thing, _, __, ___) = (1, 2, 44, "a"); // Uncaught SyntaxError: Unexpected token '('
Signatures are the only place where assets links still work.
- julmik6478
-
500+ posts
js or python
Python is lot easier. Look at this python code:
print(“Hello World”)And now look at same, but in javascript:
console.log('Hello World');Ok, this one is easy in javascript too, but more advanced things are easier in python and in python you don't need to add “;” at the end.

✊ Support the suggestion HERE by adding this button to your signature ✊
My acounts in some websites:
youtube
planet minecraft
mcreator

- robotkid828
-
100+ posts
js or python
python and js each have their own niche, but the very fact that they allow you to do this confuses me:
(though in my very unbiased opinion python will always be superior)
//javascript ([]+![])[![]+![]] 'f'
#python (at least this requires effort to do) ((f"{[]in[not[]]}"[[]or not[]])+(f"{[]in[not[]]}"))[[]or not[]] 'F' #OR f"{[]in[]}"[[]in[[[]]]] 'F'
Last edited by robotkid828 (May 20, 2024 15:41:18)
the robotkid828 suddenly appears!
random programmer/game developer here, probably wont be on scratch much other than the forums.
- BigNate469
-
1000+ posts
js or python
Technically speaking, in Python is lot easier. Look at this python code:newer versions of (See post #598) JS you don't need to add the semicolon either, just a line break.print(“Hello World”)And now look at same, but in javascript:console.log('Hello World');Ok, this one is easy in javascript too, but more advanced things are easier in python and in python you don't need to add “;” at the end.
console.log('Hello World')
console.log('Hello World');
Tip: use the [code] and [/code] tags to insert code into the forums. To specify automatic coloring based on programing language, use [code=insertlanguagenamehere][/code]
The language name must be in all lowercase.
Last edited by BigNate469 (May 21, 2024 20:10:24)
Highlight any part of this signature and press ctrl+shift+down arrow to see the rest of it.
For information on a signiture, including how to make one, visit here.
Please read the list of Officially Rejected Suggestions before posting a suggestion for Scratch!
View all of the topics you've posted in:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=topics
View all of your posts:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=posts
Lesser-known Scratch URLs:
https://scratch.mit.edu/projects/PROJECT ID HERE/remixtree (replace “PROJECT ID HERE” with project id number. Shows all the remixes of the project, and the remixes of those projects, and the remixes of those projects, and so on, as a chart. Link currently redirects to one of my projects). There was a button on the project page linking to this in 2.0, but it was removed.
View a larger list at: https://scratch.mit.edu/discuss/topic/542480/
Why @Paddle2See's responses are so often identical: https://scratch.mit.edu/discuss/topic/762351/
1000th post
The devs really need to update the scratch-gui repo- I'm getting at least 19 “npm warn deprecated” warnings whenever I try to install its dependencies, and yes, I'm using the latest version of node.js and npm. Then again, the dev page on this website and the wiki in the repo still refer to Scratch 3.0 in beta, and link the current website as an example of 2.0.
- mybearworld
-
1000+ posts
js or python
(#594)Is that newer? I thought ASI has existed since pretty early on.
Technically speaking, in newer versions of JS you don't need to add the semicolon either, just a line break.
Signatures are the only place where assets links still work.
- BigNate469
-
1000+ posts
js or python
I thought it was newer (I consider “newer” versions of JS as ES5 and up, since a small fraction of people still use Internet Explorer), but I might be wrong. MDN isn't giving me an answer, nor is the internet (or even the standard itself), so you could be correct.(#594)Is that newer? I thought ASI has existed since pretty early on.
Technically speaking, in newer versions of JS you don't need to add the semicolon either, just a line break.
Regardless, JS seems to still work better with the semicolons than without.
Highlight any part of this signature and press ctrl+shift+down arrow to see the rest of it.
For information on a signiture, including how to make one, visit here.
Please read the list of Officially Rejected Suggestions before posting a suggestion for Scratch!
View all of the topics you've posted in:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=topics
View all of your posts:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=posts
Lesser-known Scratch URLs:
https://scratch.mit.edu/projects/PROJECT ID HERE/remixtree (replace “PROJECT ID HERE” with project id number. Shows all the remixes of the project, and the remixes of those projects, and the remixes of those projects, and so on, as a chart. Link currently redirects to one of my projects). There was a button on the project page linking to this in 2.0, but it was removed.
View a larger list at: https://scratch.mit.edu/discuss/topic/542480/
Why @Paddle2See's responses are so often identical: https://scratch.mit.edu/discuss/topic/762351/
1000th post
The devs really need to update the scratch-gui repo- I'm getting at least 19 “npm warn deprecated” warnings whenever I try to install its dependencies, and yes, I'm using the latest version of node.js and npm. Then again, the dev page on this website and the wiki in the repo still refer to Scratch 3.0 in beta, and link the current website as an example of 2.0.
- alwayspaytaxes
-
100+ posts
js or python
In terms of style guides and formatting, Python undoubtedly wins. Using Black + Pylint helps format your code for basically all aspects, even how much line spacing should go between functions! ESLint is a nightmare to setup, its VSCode plugin barely works with TypeScript, and while Prettier is nice, it's just not opinionated enough for me.
Last edited by alwayspaytaxes (May 20, 2024 20:35:48)
-\{:)_/-
play sound [bad to the bone riff.wav v]
- Maximouse
-
1000+ posts
js or python
The first version of the ECMAScript standard from 1997 already mentions automatic semicolon insertion:I thought it was newer (I consider “newer” versions of JS as ES5 and up, since a small fraction of people still use Internet Explorer), but I might be wrong. MDN isn't giving me an answer, nor is the internet (or even the standard itself), so you could be correct. Is that newer? I thought ASI has existed since pretty early on.

- mybearworld
-
1000+ posts
js or python
(#597)Can you give an example of Prettier not being opinionated enough? I haven't had that experience.
In terms of style guides and formatting, Python undoubtedly wins. Using Black + Pylint helps format your code for basically all aspects, even how much line spacing should go between functions! ESLint is a nightmare to setup, its VSCode plugin barely works with TypeScript, and while Prettier is nice, it's just not opinionated enough for me.
Signatures are the only place where assets links still work.
- alwayspaytaxes
-
100+ posts
js or python
It doesn't space out functions with a certain amount of lines like Black, and import sorting is essentially non-existent(#597)Can you give an example of Prettier not being opinionated enough? I haven't had that experience.
In terms of style guides and formatting, Python undoubtedly wins. Using Black + Pylint helps format your code for basically all aspects, even how much line spacing should go between functions! ESLint is a nightmare to setup, its VSCode plugin barely works with TypeScript, and while Prettier is nice, it's just not opinionated enough for me.
Last edited by alwayspaytaxes (May 21, 2024 11:42:50)
-\{:)_/-
play sound [bad to the bone riff.wav v]
- Discussion Forums
- » Advanced Topics
-
» js or python