Discuss Scratch
- Discussion Forums
- » Developing Scratch Extensions
- » HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
- IronBill05
-
89 posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
Hello! I have been developing an extension for TurboWarp called HTML Blocks!
It is used for creating HTML pages (obviously!) and can also run JavaScript code
It also works on other Scratch mods like LibreKitten
——————————————————————————————————
Version 1.0.4
The latest code is here:
It is used for creating HTML pages (obviously!) and can also run JavaScript code
It also works on other Scratch mods like LibreKitten
——————————————————————————————————
Version 1.0.4
The latest code is here:
/*
Name: HTML Blocks
ID: htmlblocks
Description: Blocks for coding in HTML. It can also run JavaScript code!
By: IronBill05 <https://scratch.mit.edu/users/IronBill05/>
*/
(function(Scratch) {
'use strict';
if (!Scratch.extensions.unsandboxed) {
throw new Error('HTMLblocks must run unsandboxed');
}
class HTMLblocks {
getInfo() {
return {
id: 'HTMLblocks',
name: 'HTML Blocks',
color1: '#ff7777',
color2: '#ee6666',
color3: '#dd5555',
blocks: [
{
opcode: 'HTMLtag',
blockType: Scratch.BlockType.REPORTER,
text: 'HTML tag [ONE]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'h1'
},
}
},
{
opcode: 'HTMLtagWithAttr',
blockType: Scratch.BlockType.REPORTER,
text: 'HTML tag [ONE] with attributes [TWO]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'div'
},
TWO: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'id="div1"'
},
}
},
{
opcode: 'HTMLtagWithContents',
blockType: Scratch.BlockType.REPORTER,
text: 'HTML tag [ONE] contents [TWO]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'div'
},
TWO: {
type: Scratch.ArgumentType.STRING,
defaultValue: '<p> Hello! </p>'
},
}
},
{
opcode: 'HTMLtagContentsAndAttributes',
blockType: Scratch.BlockType.REPORTER,
text: 'HTML tag [ONE] attributes [TWO] contents [THREE]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'div'
},
TWO: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'id="header"'
},
THREE: {
type: Scratch.ArgumentType.STRING,
defaultValue: '<h1> Hello! </h1>'
},
}
},
{
opcode: 'encodeToDataURL',
blockType: Scratch.BlockType.REPORTER,
text: 'encode HTML [ONE] to URL',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: '<div> Hello! </div>'
},
}
},
{
opcode: 'openHTMLcode',
blockType: Scratch.BlockType.COMMAND,
text: 'open HTML [ONE]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: '<div> Hello! </div>'
},
}
},
{
opcode: 'RunJS',
blockType: Scratch.BlockType.COMMAND,
text: 'Run JS code [ONE]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'console.log("Hello World!")'
},
}
},
{
opcode: 'RunJSwithReturn',
blockType: Scratch.BlockType.REPORTER,
text: 'Evaluate JS code [ONE]',
arguments: {
ONE: {
type: Scratch.ArgumentType.STRING,
defaultValue: 'return("Hello World!")'
},
}
},
]
};
}
HTMLtag(args) {
return(`<${args.ONE}> </${args.ONE}>`);
}
HTMLtagWithAttr(args) {
return(`<${args.ONE} ${args.TWO}> </${args.ONE}>`);
}
HTMLtagWithContents(args) {
return(`<${args.ONE}> ${args.TWO} </${args.ONE}>`);
}
HTMLtagContentsAndAttributes(args) {
return(`<${args.ONE} ${args.TWO}> ${args.THREE} </${args.ONE}>`);
}
encodeToDataURL(args) {
return(`data:text/html, ${args.ONE}`);
}
openHTMLcode(args) {
let codepage = window.open(`data:text/html,<!DOCTYPE html> <html> <head> </head> <body> </body> </html>`);
codepage.document.body.innerHTML = args.ONE;
}
RunJS(args) {
eval(args.ONE);
}
RunJSwithReturn(args) {
return(eval("{ let funct = function() {" + args.ONE + "}; funct(); }"));
}
}
Scratch.extensions.register(new HTMLblocks());
})(Scratch);
——————————————————————————————————
How to use
1. Copy the code (in the box above) with CTRL + C or COMMAND + C
2. Open TurboWarp and click the extensions button.
3. Click “Custom Extension” from the menu.
4. Click “Text” and paste the code you copied in step 1.
5. Select “Run extension without sandbox” so it is allowed to run JavaScript.
6. Click the button that says Load, and the blocks should appear!
7. You are finished, and now you can start coding!
——————————————————————————————————
Known Bugs
- The “open HTML” block opens a blank page instead of a page with your code. - Trying to fix, having trouble
——————————————————————————————————
Last edited by IronBill05 (May 20, 2024 23:02:46)
- Jensvp2
-
100+ posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
well uhhm
How do i use this?
How do i use this?
- caftingdead261
-
96 posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
this is because it allows people to run malicious code The TurboWarp docs say that eval() is not allowed, but it works
- IronBill05
-
89 posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
Copy the code in the box by highlighting all of the code, and pressing ctrl+c. Go to turbowarp, click the extensions button, and select custom extension from the menu. Click “text” or “code” and paste in the HTML blocks code. Select run without sandbox (so it can run JavaScript) and click Ok or click the “Add extension” button. Then all of the new blocks will appear, and you can use them. well uhhm
How do i use this?
- Mahdir2111
-
100+ posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
I tried to add it but it doesn't work Hey everyone! I just remade the extension with an IIFE (immediately invoked function expression) in mind, so it works a bit better!
Stay on the lookout for future updates!
- IronBill05
-
89 posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
I tried to add it but it doesn't work Hey everyone! I just remade the extension with an IIFE (immediately invoked function expression) in mind, so it works a bit better!
Stay on the lookout for future updates!
Maybe try downloading it as a .js file? You can do it by pasting it in notepad or whatever text editor you have, then saving is as a javascript file (add .js to the end of the file name) and then load the new file into turbowarp as an extension.
- CST1229
-
1000+ posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
(#1)This is true, it only applies for the acceptance criteria for the gallery. Extensions loaded from file/text can use eval all they want.
*The TurboWarp docs say that eval() is not allowed, but it works, and i am assuming it is just if you are sharing your extension to the gallery. TurboWarp - CONTRIBUTING.md
This is a signature. It's a piece of text that appears below every post I write. Click here to learn more, including how to make your own.
RIP assets image hosting. 2013?-2023

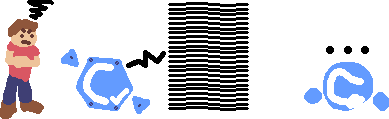
RIP assets image hosting. 2013?-2023

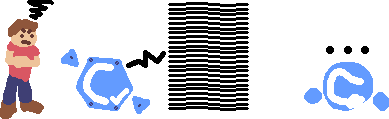
- IronAlt
-
2 posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
(some random unnecessary post that I made)
Last edited by IronAlt (May 12, 2024 14:11:46)
- BigNate469
-
500+ posts
HTML Blocks - TurboWarp Extension - Run JavaScript code!!!
They were, (relatively) briefly, for the ST to fix the 2.0 page bug (better known as the “My Stuff” bug, where 2.0 pages weren't loading properly or at all) Hmm, I thought the forums were going to be down…
Highlight any part of this signature and press ctrl+shift+down arrow to see the rest of it
foreverPlease read the list of Officially Rejected Suggestions before posting a suggestion for Scratch! 100th post
if <person asks [what's a signature] :: sensing> then
Redirect to [https://en.scratch-wiki.info/wiki/Signature] :: motion
end
end
This signature is designed to be as helpful as possible.
View all of the topics you've posted in:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=topics
View all of your posts:
https://scratch.mit.edu/discuss/search/?action=show_user&show_as=posts
Forum tips:
Don't post in topics where the latest post is over ~2 months old, unless you have something critical to add. Especially in topics that are several years old- it isn't helpful, and is known as necroposting.
Don't post unrelated things in topics, including questions of your own. Make a new topic for your questions.
You can use the
[color=color name or hexadecimal value here] and [/color]
Lesser-known Scratch URLs:
scratch.pizza (redirects to main page)
https://scratch.mit.edu/projects/PROJECT ID HERE/remixtree (replace “PROJECT ID HERE” with project id number. Shows all the remixes of the project, and the remixes of those projects, and the remixes of those projects, and so on, as a chart. Link currently redirects to one of my projects)
View a larger list at: https://scratch.mit.edu/discuss/topic/542480/
Why @Paddle2See's responses are so often identical: https://scratch.mit.edu/discuss/topic/762351/
- Discussion Forums
- » Developing Scratch Extensions
-
» HTML Blocks - TurboWarp Extension - Run JavaScript code!!!