Discuss Scratch
- Discussion Forums
- » Things I'm Making and Creating
- » Python programming language
- k0d3rrr
-
1000+ posts
Python programming language
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.
import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
- kkidslogin
-
1000+ posts
Python programming language
(#981)How long does it take to finish lol
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
Also, these might look nicer:
cool += cool
cool *= 2
- gilbert_given_189
-
500+ posts
Python programming language
I had a feeling there’s an easier way to do this but I implemented this
class Scope: def __enter__(self): self.glb_keys = set(globals().keys()) def __exit__(self, exc_type, exc_value, traceback): glb_keys = set(globals().keys()) for diff in glb_keys - self.glb_keys: del globals()[diff] foo = 1 bar = 2 with Scope(): baz = 3 assert "baz" not in globals()
If you see a line above this text, it means that below this text is my signature.
This place is just a memory to me, I may return occasionally but I'm busy.
I guess I'm an ATer now.
I think I may have seasoned my posts a bit too much.
Also, my posts are getting lengthy lately. Whoops.
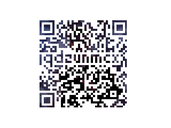
Colored Pencil is supposed to color the siggy, but Scratch says it's too big.
There is nothing here…
don don pan pan
dondo pan pan
- banana3333333333
-
500+ posts
Python programming language
>be me(#981)How long does it take to finish lol
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
Also, these might look nicer:orcool += coolcool *= 2
>be a python mastermind
>see these two posts
>holdup lemme rewrite this rq
import math num = 0.01 while True: num *= 2 if math.isinf(num): print("Number is no longer cool") break print(f"Number {num} is a cool number")
>i have made no contributions to society
>post this
siggy change for the first time since 2023!! real
select anything and shift+up/down to scroll or you can just use (THE EXTENSION THAT SHALL NOT BE NAMED)
im a developer. i primarily code on scratch because the limitations are fun to try to get around. i also sometimes code in python, and i like to dabble in web design. i have a github site, here. im poor so i cant afford any web servers or domains so i have to stick with static sites
i am also a music creator. i am in the band stock and am working on a solo project. im an aspiring synthist
lis of langags i kno (cuz im a devleoper)
- pyton
- jav
- html
- xml
- javscip
- css
- some c/c++
- c#
- english
- scrtc
will clean this up later
publically controlled image (i do not necessarily condone this image or approve of it)
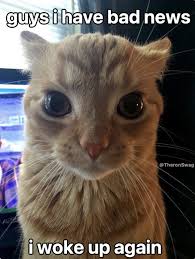
- banana3333333333
-
500+ posts
Python programming language
what does this do I had a feeling there’s an easier way to do this but I implemented thisclass Scope: def __enter__(self): self.glb_keys = set(globals().keys()) def __exit__(self, exc_type, exc_value, traceback): glb_keys = set(globals().keys()) for diff in glb_keys - self.glb_keys: del globals()[diff] foo = 1 bar = 2 with Scope(): baz = 3 assert "baz" not in globals()
siggy change for the first time since 2023!! real
select anything and shift+up/down to scroll or you can just use (THE EXTENSION THAT SHALL NOT BE NAMED)
im a developer. i primarily code on scratch because the limitations are fun to try to get around. i also sometimes code in python, and i like to dabble in web design. i have a github site, here. im poor so i cant afford any web servers or domains so i have to stick with static sites
i am also a music creator. i am in the band stock and am working on a solo project. im an aspiring synthist
lis of langags i kno (cuz im a devleoper)
- pyton
- jav
- html
- xml
- javscip
- css
- some c/c++
- c#
- english
- scrtc
will clean this up later
publically controlled image (i do not necessarily condone this image or approve of it)
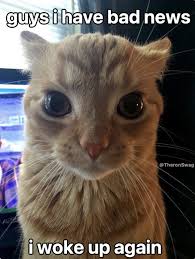
- kkidslogin
-
1000+ posts
Python programming language
(#985)Nobody knows.what does this do I had a feeling there’s an easier way to do this but I implemented thisclass Scope: def __enter__(self): self.glb_keys = set(globals().keys()) def __exit__(self, exc_type, exc_value, traceback): glb_keys = set(globals().keys()) for diff in glb_keys - self.glb_keys: del globals()[diff] foo = 1 bar = 2 with Scope(): baz = 3 assert "baz" not in globals()
- divtrev2013
-
26 posts
Python programming language
Try to use `pip install pygame` in your Termail or Command Prompt If it doesn't work, check if your Python version is 3.4+. If it is, chack if pip is installed. If not, install it. If it is installed, check if Python is added to environment variables. If it's not, make it. Then try `pip install pygame` again. I am making a 3d zombie survival game called swarm in python.
also does anyone know if pygame is working for me? Everytime I add a script like “import pygame” it gives me errors with the note Invalid syntax. It shows a red line on the first > in the script.
- gilbert_given_189
-
500+ posts
Python programming language
what does this do I had a feeling there’s an easier way to do this but I implemented thisclass Scope: def __enter__(self): self.glb_keys = set(globals().keys()) def __exit__(self, exc_type, exc_value, traceback): glb_keys = set(globals().keys()) for diff in glb_keys - self.glb_keys: del globals()[diff] foo = 1 bar = 2 with Scope(): baz = 3 assert "baz" not in globals()
It comes in a lot of names, but the main idea is that it creates a new scope, in which new variables could be made temporarily within that scope. It is closely equivalent to this C program:
int main() { int foo = 1; int bar = 2; { int baz = 3; } // there shouldn't be baz here... }
If you see a line above this text, it means that below this text is my signature.
This place is just a memory to me, I may return occasionally but I'm busy.
I guess I'm an ATer now.
I think I may have seasoned my posts a bit too much.
Also, my posts are getting lengthy lately. Whoops.
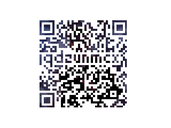
Colored Pencil is supposed to color the siggy, but Scratch says it's too big.
There is nothing here…
don don pan pan
dondo pan pan
- mumu245
-
1000+ posts
Python programming language
(#981)Python ints have infinite precision, so it will never reach infinity.
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
- zaid1442011
-
500+ posts
Python programming language
(#989)Nothing has infinite precision in computers. The maximum integer for 64-bit programs is 9,223,372,036,854,775,807 before it would overflow.(#981)Python ints have infinite precision, so it will never reach infinity.
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
I am a guy who codes weird stuff. Don’t check my GitHub only if you want stupid HTML pages (or something to host them). Check my platformer if you want to see something to see.
Profiles: GitHub.
You won't understand stuff in my signature because I talk about stuff in the ATs (Advanced Topics).
Check out this song for @griffpatch https://scratch.mit.edu/projects/887382049/
- Maximouse
-
1000+ posts
Python programming language
From the Python documentation:Nothing has infinite precision in computers. The maximum integer for 64-bit programs is 9,223,372,036,854,775,807 before it would overflow. Python ints have infinite precision, so it will never reach infinity.
Integers (int)
These represent numbers in an unlimited range, subject to available (virtual) memory only.
(source)
- kkidslogin
-
1000+ posts
Python programming language
(#991)Lesson: run this program and see how long your computer can copeFrom the Python documentation:Nothing has infinite precision in computers. The maximum integer for 64-bit programs is 9,223,372,036,854,775,807 before it would overflow. Python ints have infinite precision, so it will never reach infinity.Integers (int)
These represent numbers in an unlimited range, subject to available (virtual) memory only.
(source)
- imfh
-
1000+ posts
Python programming language
The variable is a float, so it will max out around 10^308 ish. I’m not sure if it will turn into infinity at that point or give an overflow error. I suspect the latter, but I’m not sure.(#981)Python ints have infinite precision, so it will never reach infinity.
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
Scratch to Pygame converter: https://scratch.mit.edu/discuss/topic/600562/
- yadayadayadagoodbye
-
1000+ posts
Python programming language
python my beloved
turns out i was wrong, it returns the overflow error
I remember it turns into infinity, i could test rnThe variable is a float, so it will max out around 10^308 ish. I’m not sure if it will turn into infinity at that point or give an overflow error. I suspect the latter, but I’m not sure.(#981)Python ints have infinite precision, so it will never reach infinity.
I gave up on trying to learn JavaScript, so instead I made a “game” in Python that detects whether or not the ‘cool’ variable equals infinity.import math cool = 0.01 while True: cool = cool + cool if math.isinf(cool): print(cool, 'is not a cool number.') break print(cool, 'is a cool number.')
turns out i was wrong, it returns the overflow error
Last edited by yadayadayadagoodbye (Feb. 5, 2024 21:50:46)
- zaid1442011
-
500+ posts
Python programming language
(#991)Bolded the important part.From the Python documentation:Nothing has infinite precision in computers. The maximum integer for 64-bit programs is 9,223,372,036,854,775,807 before it would overflow. Python ints have infinite precision, so it will never reach infinity.Integers (int)
These represent numbers in an unlimited range, subject to available (virtual) memory only.
(source)
I am a guy who codes weird stuff. Don’t check my GitHub only if you want stupid HTML pages (or something to host them). Check my platformer if you want to see something to see.
Profiles: GitHub.
You won't understand stuff in my signature because I talk about stuff in the ATs (Advanced Topics).
Check out this song for @griffpatch https://scratch.mit.edu/projects/887382049/
- banana3333333333
-
500+ posts
Python programming language
who else here uses flask on a daily basis
i use it to run my html files on a server that other computers in my house can access (ip stuff idk i don't quite understand it) so i can show people like my dad or my sister what i do in my free time
i also use ngrok (port forwarding service) to put them online but nobody i send the urls to clicks on them (maybe because they look like this: v5ut5e8uj8gusjivjv8tkiyrejb.ngrok.free)
also i made a sort of web hosting site with it where you can post one-file html pages (with no size limit; so my computer's hard drive cried whenever i ran it) and shared it with all my friends
i can post the source if anybody wants to but whatever
tl;dr flask is super flipin kewl
i use it to run my html files on a server that other computers in my house can access (ip stuff idk i don't quite understand it) so i can show people like my dad or my sister what i do in my free time
i also use ngrok (port forwarding service) to put them online but nobody i send the urls to clicks on them (maybe because they look like this: v5ut5e8uj8gusjivjv8tkiyrejb.ngrok.free)
also i made a sort of web hosting site with it where you can post one-file html pages (with no size limit; so my computer's hard drive cried whenever i ran it) and shared it with all my friends
i can post the source if anybody wants to but whatever

tl;dr flask is super flipin kewl
Last edited by banana3333333333 (Feb. 6, 2024 18:06:34)
siggy change for the first time since 2023!! real
select anything and shift+up/down to scroll or you can just use (THE EXTENSION THAT SHALL NOT BE NAMED)
im a developer. i primarily code on scratch because the limitations are fun to try to get around. i also sometimes code in python, and i like to dabble in web design. i have a github site, here. im poor so i cant afford any web servers or domains so i have to stick with static sites
i am also a music creator. i am in the band stock and am working on a solo project. im an aspiring synthist
lis of langags i kno (cuz im a devleoper)
- pyton
- jav
- html
- xml
- javscip
- css
- some c/c++
- c#
- english
- scrtc
will clean this up later
publically controlled image (i do not necessarily condone this image or approve of it)
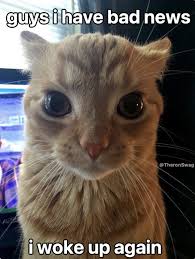
- kkidslogin
-
1000+ posts
Python programming language
(#996)I once used flask to make a forum in a week.
who else here uses flask on a daily basis
i use it to run my html files on a server that other computers in my house can access (ip stuff idk i don't quite understand it) so i can show people like my dad or my sister what i do in my free time
i also use ngrok (port forwarding service) to put them online but nobody i send the urls to clicks on them (maybe because they look like this: v5ut5e8uj8gusjivjv8tkiyrejb.ngrok.free)
also i made a sort of web hosting site with it where you can post one-file html pages (with no size limit; so my computer's hard drive cried whenever i ran it) and shared it with all my friends
i can post the source if anybody wants to but whatever
tl;dr flask is super flipin kewl
- Chiroyce
-
1000+ posts
Python programming language
ive used it extensively, was my primary backend for a long time, i use it now for REST APIs, for web apps i use a framework with server functions like SvelteKit or Next.js. its very powerful if you want a basic full stack site with templating + vanilla css who else here uses flask on a daily basis
maybe try cloudflare tunnels? i think that has shorter domain names, and ofc you can always get a domain and configure it. i havent used it but know a few people who use it to self host i also use ngrok (port forwarding service) to put them online but nobody i send the urls to clicks on them (maybe because they look like this: v5ut5e8uj8gusjivjv8tkiyrejb.ngrok.free)
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence
- Quantum1993
-
500+ posts
Python programming language
I'm just starting to learn Python. Any tips?
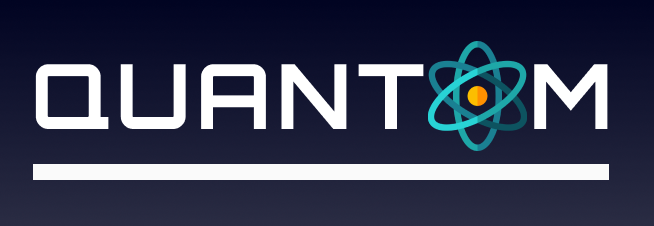
If you are seeing this, you've found my old signature because my banner isn't loading for some reason. It is most likely due to your system admin blocking cubeupload, or because it simply hasn't loaded yet. Comment ‘null5’ on my profile to show you found the secret.
Manager of Quantum Games and Quantum Software
Are you an experienced Scratcher? Join the Jurassic Park: Security Shift Development Team at https://scratch.mit.edu/studios/34523787
- Chiroyce
-
1000+ posts
Python programming language
make stuff on your own, even if its dead simple like a calculator or a todo list or a calendar or a game or anything simple, DO NOT fall into tutorial hell and only make stuff you see in tutorials, use them for only learning the syntax and its features and how you structure your code. first thing to do after learning the basics is make your own scripts/projects that you can benefit from or show off to friends/family I'm just starting to learn Python. Any tips?
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence