Discuss Scratch
- Discussion Forums
- » Advanced Topics
- » js or python
- Sheep_maker
-
1000+ posts
js or python
@Greg8128 In JS there's a do expression proposal (stage 1) that aims to let you use statements as expressions; for example:
which makes statements return whatever they would if they were eval'd (or run in the console)
Of course, it's not as elegant as in other programming languages, unfortunately.
JavaScript is very asynchronous, so you might find yourself writing code with callbacks like
Had `let` instead been `var`, this would log “10” ten times, and back in the day, we'd have to use an IIFE to keep the value of `i` in each iteration of the loop. Now that `let` is blocked scope, this isn't a problem
Python is less likely to encounter these situations where an inner function references a variable from an outer function that is later changed because Python's “inner functions,” lambda expressions, are much more restricted. While you could instead define a function inside the loop, it's a lot harder to encounter situations in Python where you'd need callbacks like these. Even with Python's async/await, generally everything is written as if it should be executed in series, and executing something in parallel requires a bit more code (because running something async in parallel is usually a bug that often occurs in JS)
However, because Python's lambda expressions (and expressions in general) are very limited—probably because of their indentation-sensitive statement syntax—it's usually more convenient to take advantage of Python's function-scoped variables, like
In JS, you could just use an IIFE, though it's not very elegant; I agree with @Greg8128 that languages that unify statements and expressions are ideal
const val = do { if (condition) { const wow = a && b || c && d if (wow) { !a } else { b } } else { false } }
Of course, it's not as elegant as in other programming languages, unfortunately.
I think both languages' scoping are a good fit for themselves.OTOH, doesn't this make it a bit more convenient to use temporary variables in Javascript (and literally any other language with block scoping)? Also, does Python have IIFE's that would provide a construct for temporary variables? I'm just wondering Also, because Python variables are function scoped, it's not as inconvenient to store a value from an if-else or match statement as it is in JS with its block scoped let/const variables
I guess del could be used for temporary variables
JavaScript is very asynchronous, so you might find yourself writing code with callbacks like
for (let i = 0; i < 10; i++) { setTimeout(() => { console.log(i) }, i * 100) }
Python is less likely to encounter these situations where an inner function references a variable from an outer function that is later changed because Python's “inner functions,” lambda expressions, are much more restricted. While you could instead define a function inside the loop, it's a lot harder to encounter situations in Python where you'd need callbacks like these. Even with Python's async/await, generally everything is written as if it should be executed in series, and executing something in parallel requires a bit more code (because running something async in parallel is usually a bug that often occurs in JS)
However, because Python's lambda expressions (and expressions in general) are very limited—probably because of their indentation-sensitive statement syntax—it's usually more convenient to take advantage of Python's function-scoped variables, like
if condition: temp_var = wow() value = temp_var * 2 else: value = 'b' print(value)
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- PkmnQ
-
1000+ posts
js or python
I might try out Scala.In most languages, statements (such as if/else and switch) are used to select which code to run. But in newer and cooler languages, they can be used to return a value. One such language is Scala:Please elaborate on the “statements as expressions” part, I don't quite understand These languages didn't get in on the “statements as expressions” idea, and as a result JavaScript doesn't have it, either.
In Scala, the if/else statement can be used as an expression, so a separate ternary operator is not needed.But there are also cooler applications. For example, the “match” expression is like a cooler version of the “switch” statement. Just like the if/else expression, it can be used to return a value.val b = true val i = if (b) 3 else 2This is pretty neat because it prevents you from needing to write “j = ” a bunch of times, and from needing to declare the variable j separately from assigning it.val i = 2 val j = i match { case 1 => "one" case 2 => "two" case _ => "many" }
Another thing you can do is use blocks as expressions:Think of it as a very simple closure that has no arguments and returns the last expression.val f = 3; val i = {val j = 2; j * f + 3} // We only use j to calculate i
Overall, the point of being able to use statements as expressions is to be able to write in a more expressive style, with less boilerplate and a clearer relationship between the different calculations involved.
This is an account that exists.
Here, have a useful link:

- Raihan142857
-
1000+ posts
js or python
do expression proposal (stage 1) that aims to let you use statements as expressions; for example:Couldn't this conflict with do {} while() ? @Greg8128 In JS there's awhich makes statements return whatever they would if they were eval'd (or run in the console)const val = do { if (condition) { const wow = a && b || c && d if (wow) { !a } else { b } } else { false } }
I use scratch.
GF: I'll dump you. BF: hex dump or binary dump?
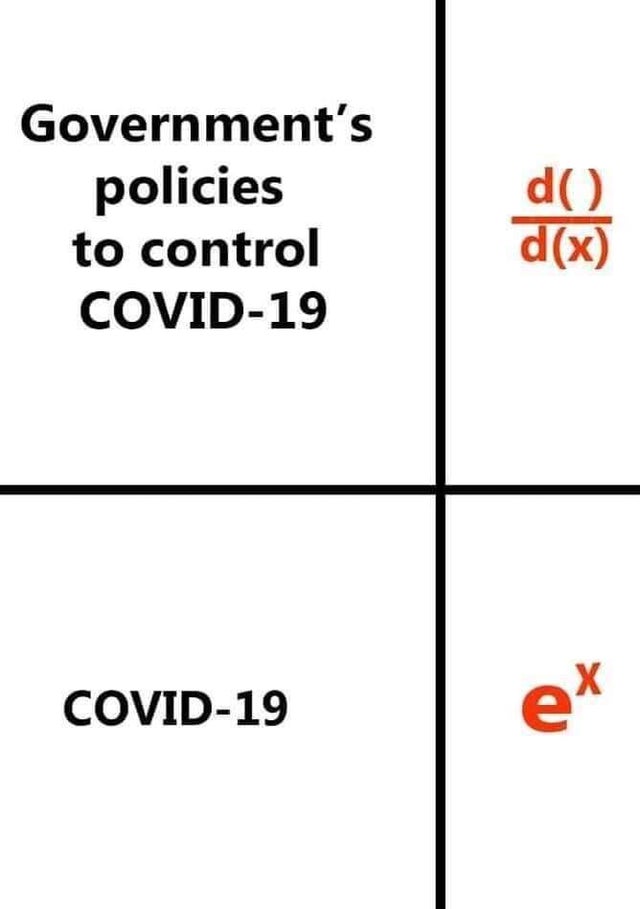
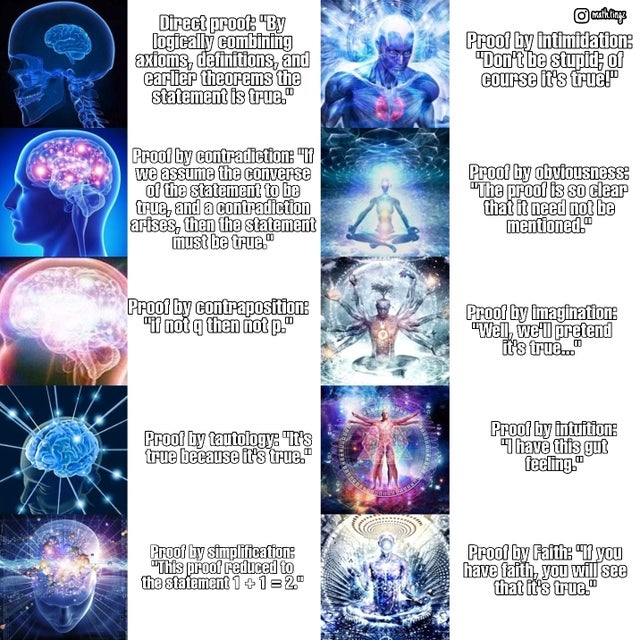
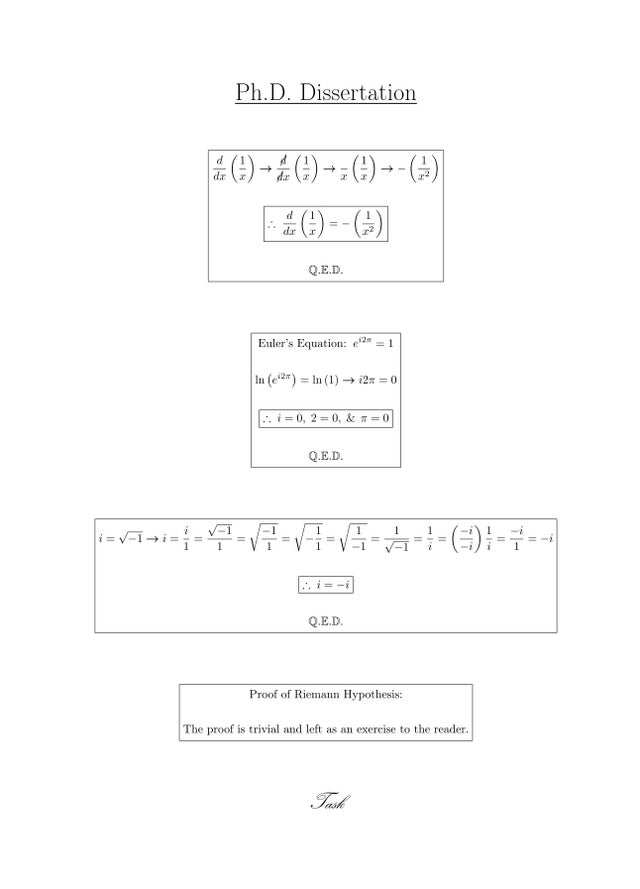
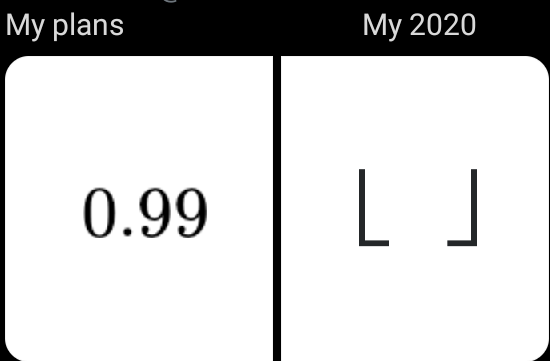
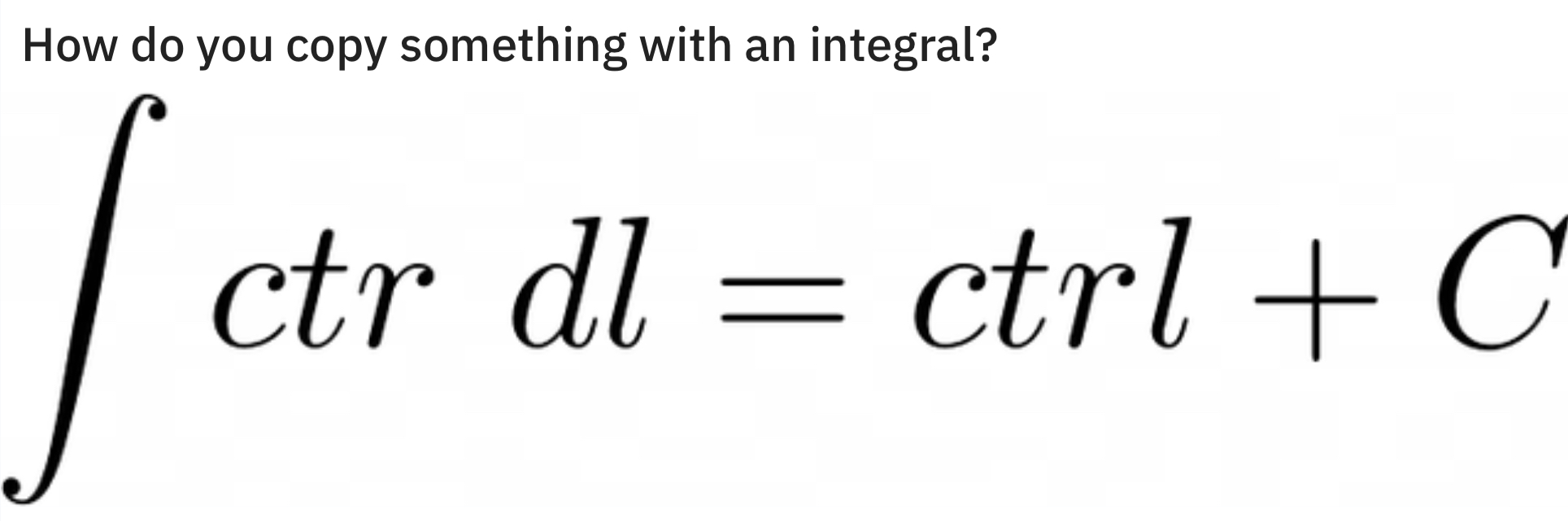
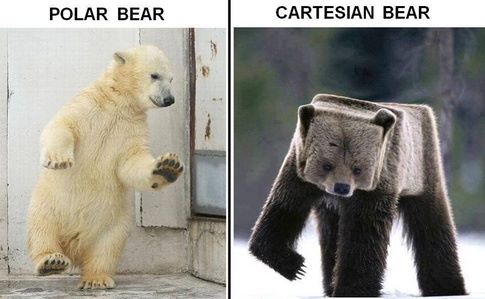
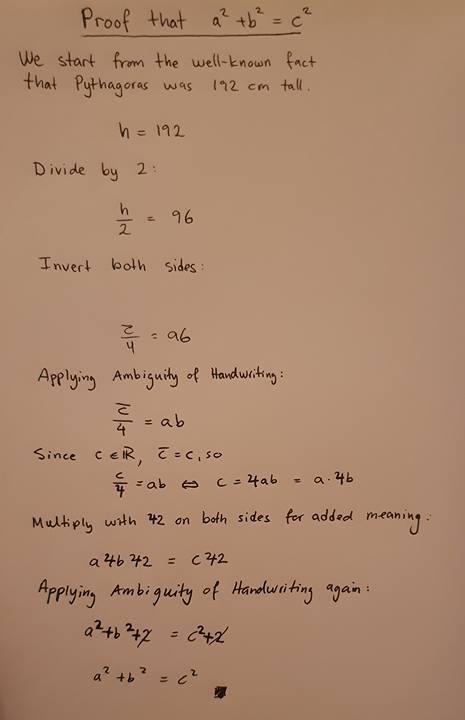
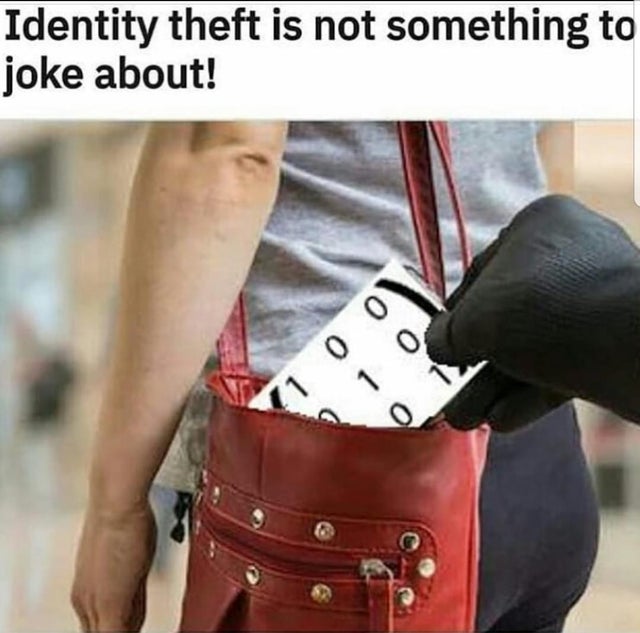
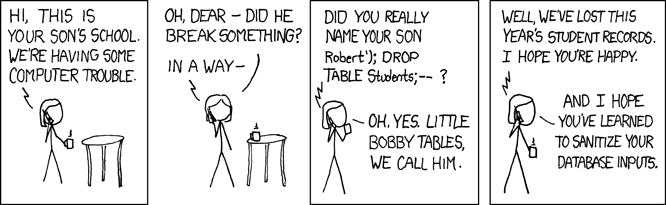
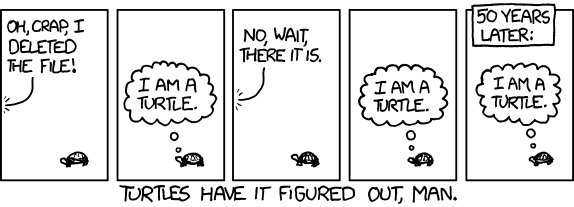
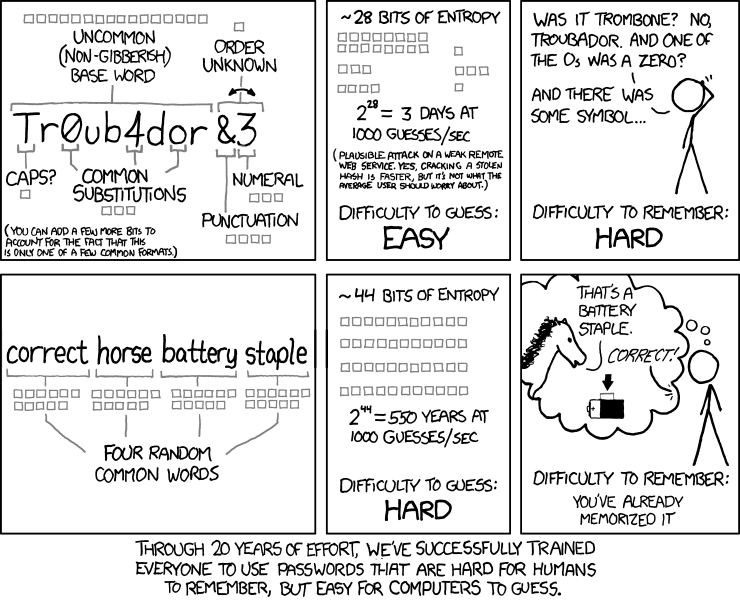
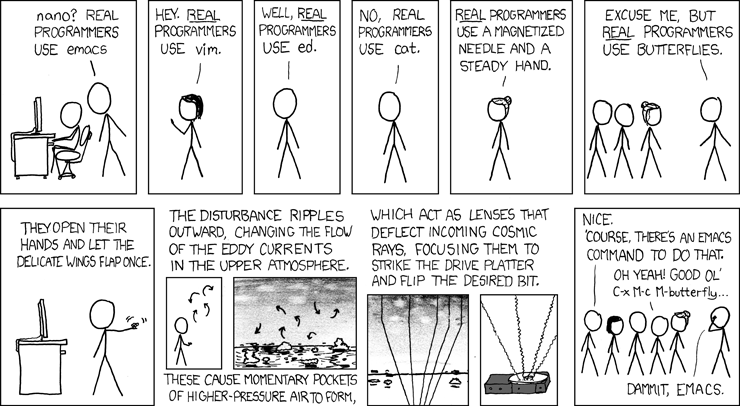
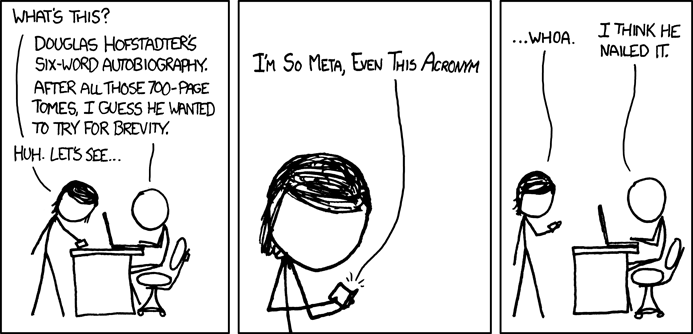
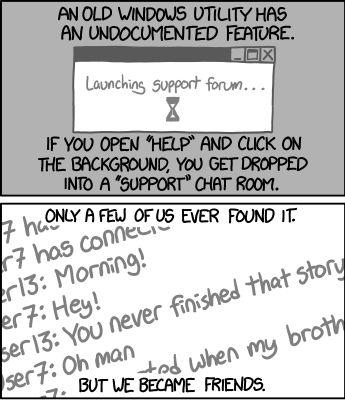
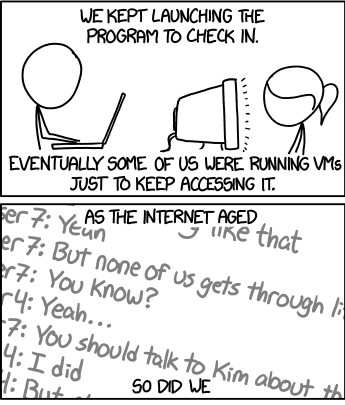
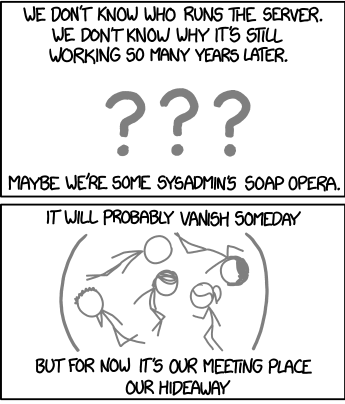
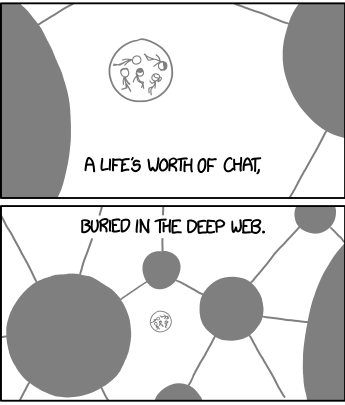
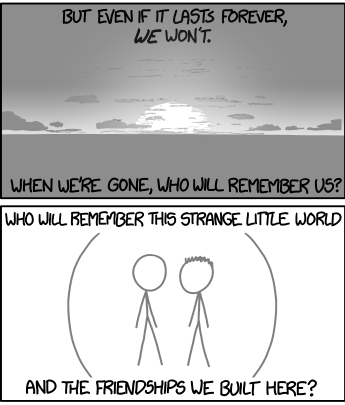
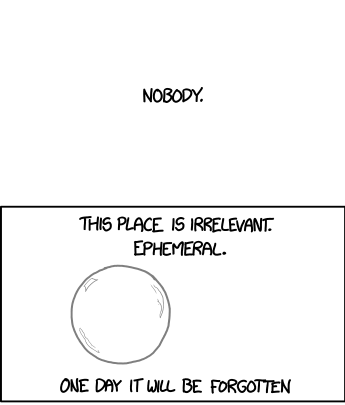
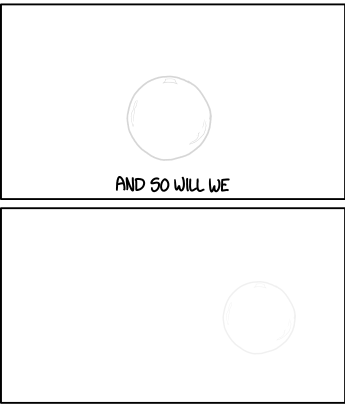
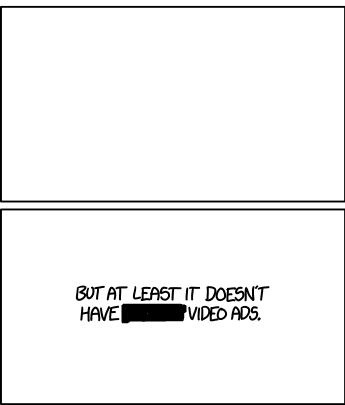
- Greg8128
-
500+ posts
js or python
You can't use a do{} as a statement (the only place where you could use a do-while) so it doesn't matter. But honestly I think that the proposal is pretty ugly; the way it is done in Rust, Scala, etc, is much nicer.do expression proposal (stage 1) that aims to let you use statements as expressions; for example:Couldn't this conflict with do {} while() ? @Greg8128 In JS there's awhich makes statements return whatever they would if they were eval'd (or run in the console)const val = do { if (condition) { const wow = a && b || c && d if (wow) { !a } else { b } } else { false } }
- Maximouse
-
1000+ posts
js or python
del could be used for temporary variablesIn Python you just don't use temporary variables. I guess
- gdpr5b78aa4361827f5c2a08d700
-
1000+ posts
js or python
Is that not extremely messy?del could be used for temporary variablesIn Python you just don't use temporary variables. I guess
- Maximouse
-
1000+ posts
js or python
Unless you write very long functions, no.Is that not extremely messy?del could be used for temporary variablesIn Python you just don't use temporary variables. I guess
- gosoccerboy5
-
1000+ posts
js or python
Wait really? That's weird imo.del could be used for temporary variablesIn Python you just don't use temporary variables. I guess

- Maximouse
-
1000+ posts
js or python
In Python this is valid:Wait really? That's weird imo.del could be used for temporary variablesIn Python you just don't use temporary variables. I guess
for i in x: do_something_with(i) print(i)
- gdpr5b78aa4361827f5c2a08d700
-
1000+ posts
js or python
surely that's quite inefficient, memory management wise?In Python this is valid:Wait really? That's weird imo.del could be used for temporary variablesIn Python you just don't use temporary variables. I guessIt doesn't cause problems but it's weird if you aren't used to it.for i in x: do_something_with(i) print(i)
- Maximouse
-
1000+ posts
js or python
If you keep functions short it isn't really a problem. surely that's quite inefficient, memory management wise?
- gdpr5b78aa4361827f5c2a08d700
-
1000+ posts
js or python
import 2tb from download-more.ramIf you keep functions short it isn't really a problem. surely that's quite inefficient, memory management wise?
- Sikecon
-
1000+ posts
js or python
Never learned Python loololololol
so I vote JavaScript. It's simple to learn (at least in the beginning―had a hard time figuring out OOP/OOD)
so I vote JavaScript. It's simple to learn (at least in the beginning―had a hard time figuring out OOP/OOD)
hi i love Fnf like very much
growing artist
- gdpr5b78aa4361827f5c2a08d700
-
1000+ posts
js or python
Both are fun to play with and are decent for small-scale things, but don't use either of them for anything serious unless you have to.
- gosoccerboy5
-
1000+ posts
js or python
import 2tb from download-more.ramIf you keep functions short it isn't really a problem. surely that's quite inefficient, memory management wise?

Last edited by gosoccerboy5 (April 16, 2021 21:30:33)

- PkmnQ
-
1000+ posts
js or python
Ok then why does this existdel could be used for temporary variablesIn Python you just don't use temporary variables. I guess
with temp_var as "h" print(temp_var)
This is an account that exists.
Here, have a useful link:

- Maximouse
-
1000+ posts
js or python
For opening files and other things that need to be cleaned up after use. Ok then why does this existwith temp_var as "h" print(temp_var)
- ninjaMAR
-
1000+ posts
js or python
Can we just appreciate Javascript's lambda at least
stuff = lambda arg:stuff with arg