Discuss Scratch
- Discussion Forums
- » Advanced Topics
- » JavaScript help ...
- Chiroyce
-
1000+ posts
JavaScript help ...
So I want my website to make a GET request to https://server.chiroyce.repl.co/views , and return the value of the key “views”.
I used fetch, it returned some jargon that didn't even contain the JSON.
I tried using ajax and it said that ‘$’ is not defined, maybe I used some wrong version of jQuery?
So what do I do now? Source code is available here (replace ‘%40’ with ‘@’)
edit! I used
and now it shows
in the console, how do i make it print only ‘2’?
I used fetch, it returned some jargon that didn't even contain the JSON.
I tried using ajax and it said that ‘$’ is not defined, maybe I used some wrong version of jQuery?
So what do I do now? Source code is available here (replace ‘%40’ with ‘@’)
edit! I used
fetch('https://server.chiroyce.repl.co/views') .then(res => res.json()) .then((out) => { console.log('Output: ', out); }).catch(err => console.error(err));
Object { views: "2" }
Last edited by Chiroyce (May 27, 2021 09:01:02)
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence
- kccuber
-
1000+ posts
JavaScript help ...
You have to parse the JSON first with JSON.parse like this:
then call
Also, the fact that you used jquery is going to trigger all of the ATers except me
Edit:
(are you using a book? dont use the jquery they showed in the book)
This is much newer.
var obj = JSON.parse('{ views: "2" }');
obj.views
Also, the fact that you used jquery is going to trigger all of the ATers except me
Edit:
Yeah. You used 3.2.1. maybe I used some wrong version of jQuery?
(are you using a book? dont use the jquery they showed in the book)
This is much newer.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
Last edited by kccuber (May 27, 2021 12:19:59)
- Chiroyce
-
1000+ posts
JavaScript help ...
ummm …this is my code
so which variable should I parse??
fetch('https://server.chiroyce.repl.co/views') .then(res => res.json()) .then((out) => { console.log('Output: ', out); }).catch(err => console.error(err));
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence
- kccuber
-
1000+ posts
JavaScript help ...
I don't even know because every time I try running that code, it gives me a CORS error ummm …this is my codeso which variable should I parse??fetch('https://server.chiroyce.repl.co/views') .then(res => res.json()) .then((out) => { console.log('Output: ', out); }).catch(err => console.error(err));
- Maximouse
-
1000+ posts
JavaScript help ...
None – ummm …this is my coderes.json() parses it. Just change the console.log toso which variable should I parse??fetch('https://server.chiroyce.repl.co/views') .then(res => res.json()) .then((out) => { console.log('Output: ', out); }).catch(err => console.error(err));
console.log('Output: ', out.views);
- Chiroyce
-
1000+ posts
JavaScript help ...
Alright thanks, now how do I replace the contents of h4 with “This page has {views} views” ?
I added an ID for the h4 tag, like this
and I added this JS code
But it doesn't seem to work …
I added an ID for the h4 tag, like this
<h4 id="views">Loading view count . . .</h4>
document.views.innerHTML = out.views;
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence
- Maximouse
-
1000+ posts
JavaScript help ...
Alright thanks, now how do I replace the contents of h4 with “This page has {views} views” ?
I added an ID for the h4 tag, like thisand I added this JS code<h4 id="views">Loading view count . . .</h4>But it doesn't seem to work …document.views.innerHTML = out.views;
document.getElementById("views").innerText = out.views;
- Chiroyce
-
1000+ posts
JavaScript help ...
Thanks a ton!!!
April Fools' topics:
— New Buildings in Scratch's headquarters
— Give every Scratcher an M1 MacBook Air
— Scratch should let users edit other Scratchers' projects
— Make a statue for Jeffalo
— Scratch Tech Tips™
— Make a Chiroyce statue emoji
<img src=“x” onerror=“alert('XSS vulnerability discovered')”>
this is a test sentence
- kccuber
-
1000+ posts
JavaScript help ...
Thanks! I need to parse JSON in NoteFinder as well.Alright thanks, now how do I replace the contents of h4 with “This page has {views} views” ?
I added an ID for the h4 tag, like thisand I added this JS code<h4 id="views">Loading view count . . .</h4>But it doesn't seem to work …document.views.innerHTML = out.views;Only use innerHTML when you actually need the text to be parsed as HTML.document.getElementById("views").innerText = out.views;
Also, chiroyce, you use inner html? i dont use that lol
Last edited by kccuber (May 27, 2021 15:24:47)
- Raihan142857
-
1000+ posts
JavaScript help ...
inner* is bad in general. Use textContent.Alright thanks, now how do I replace the contents of h4 with “This page has {views} views” ?
I added an ID for the h4 tag, like thisand I added this JS code<h4 id="views">Loading view count . . .</h4>But it doesn't seem to work …document.views.innerHTML = out.views;Only use innerHTML when you actually need the text to be parsed as HTML.document.getElementById("views").innerText = out.views;
document.getElementById("views").textContent = out.views;
I use scratch.
GF: I'll dump you. BF: hex dump or binary dump?
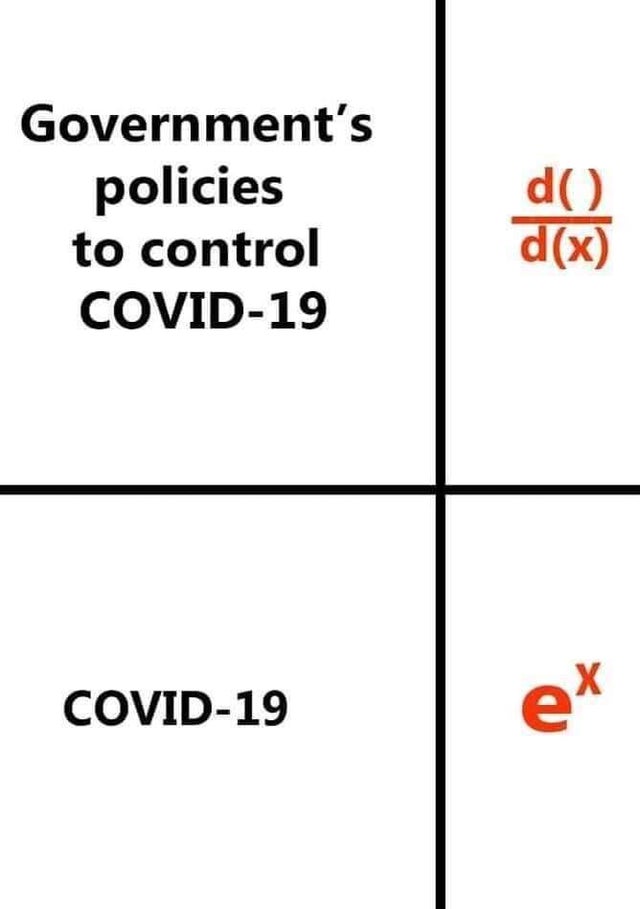
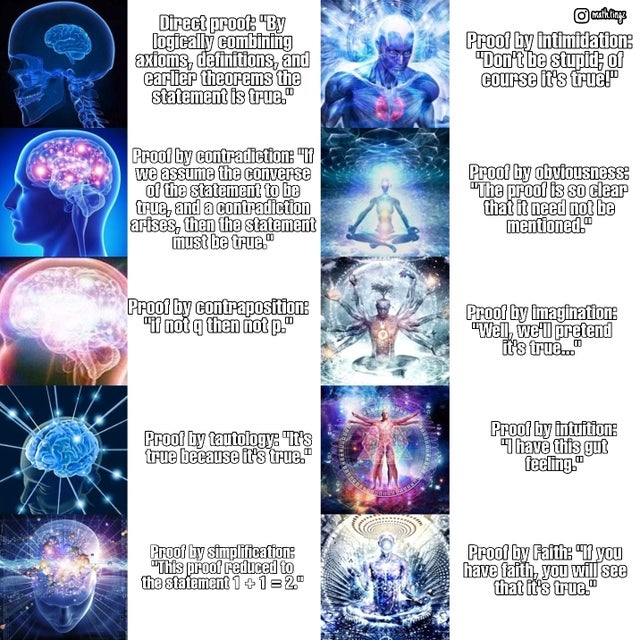
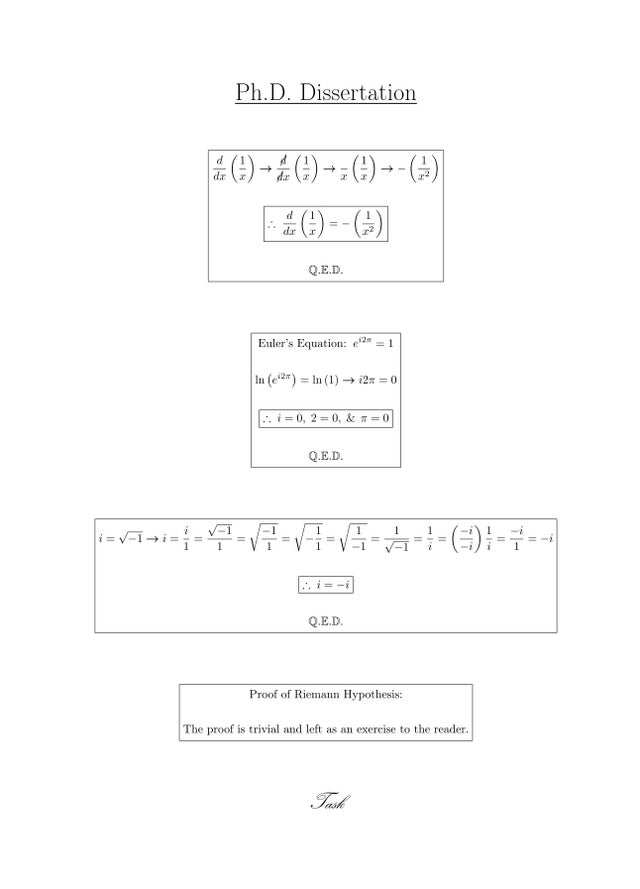
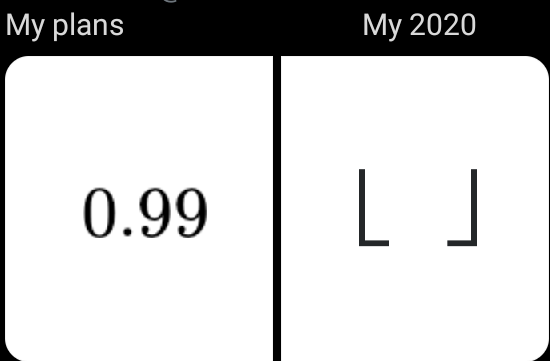
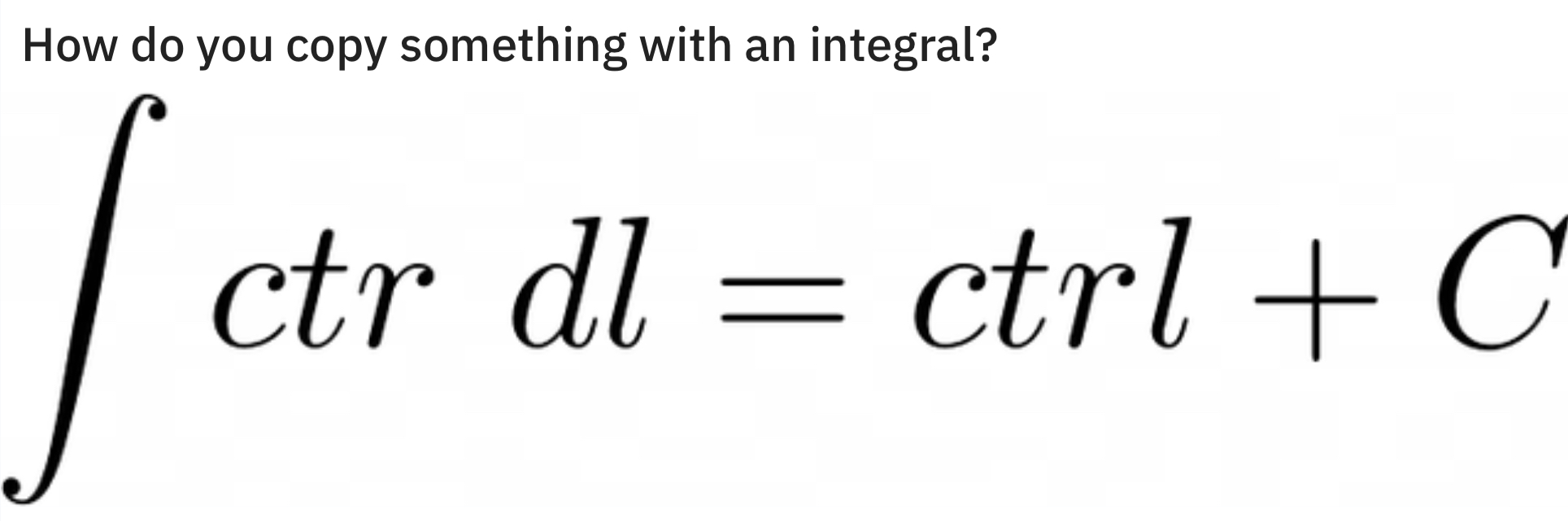
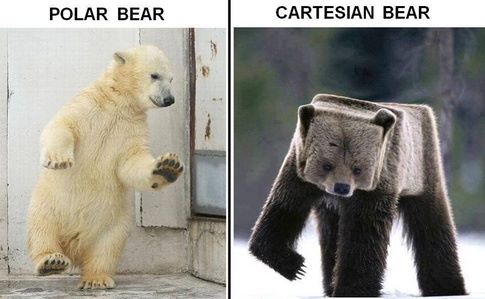
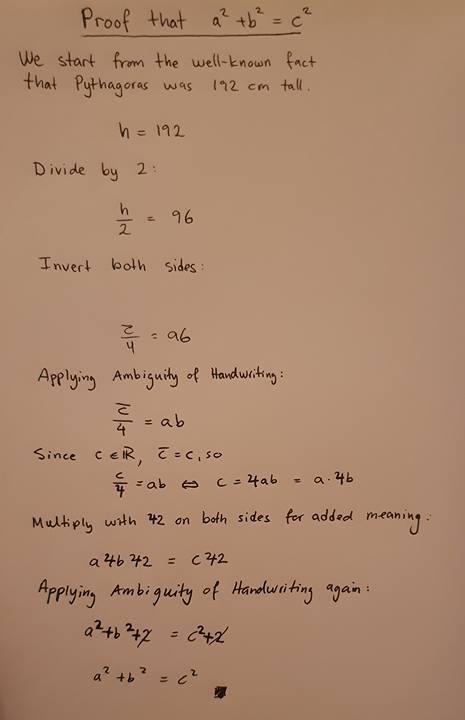
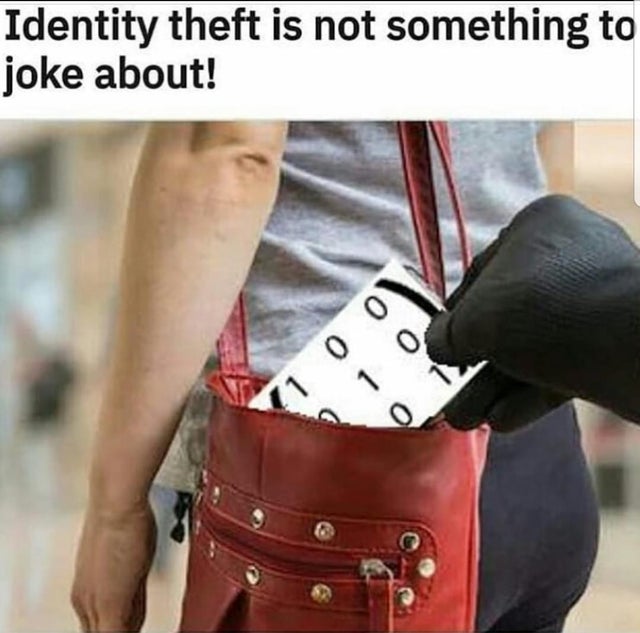
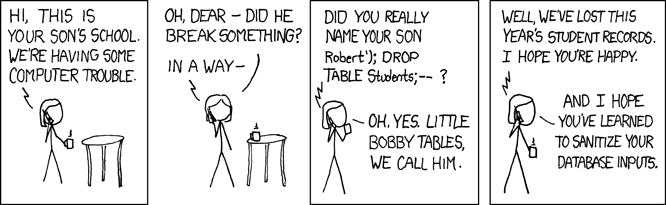
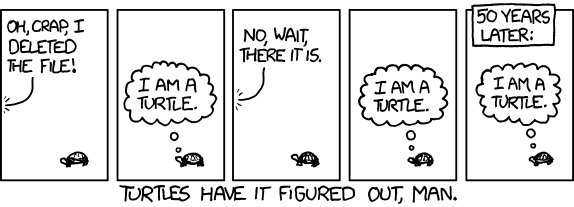
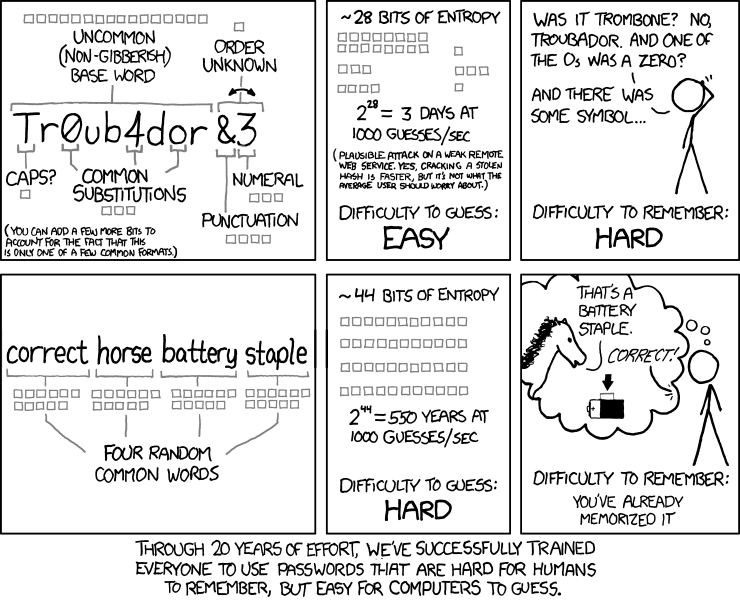
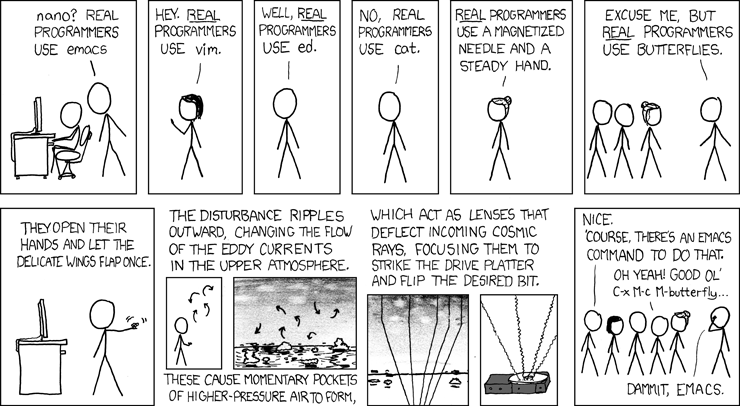
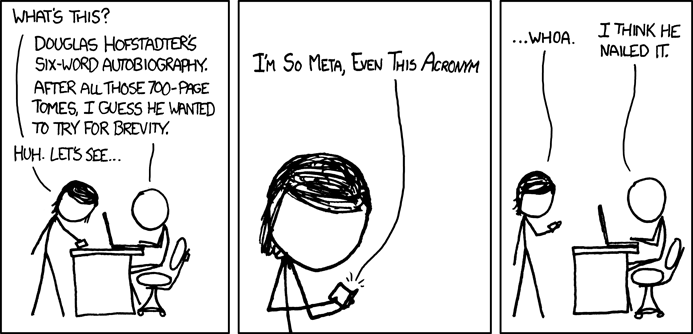
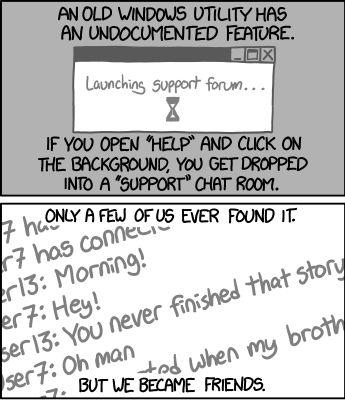
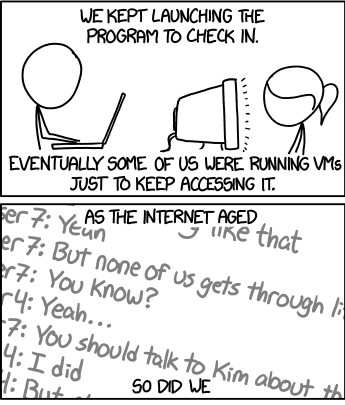
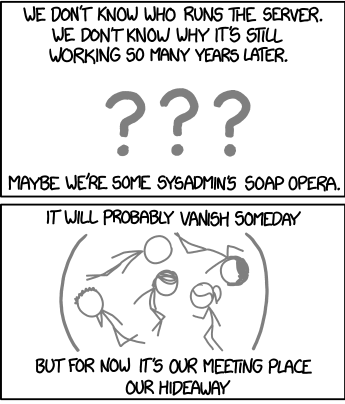
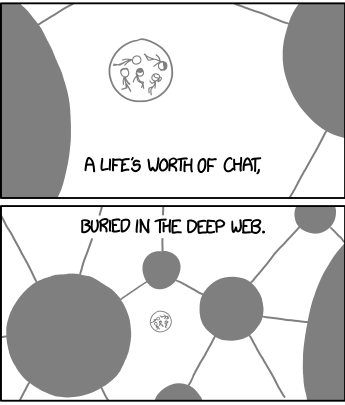
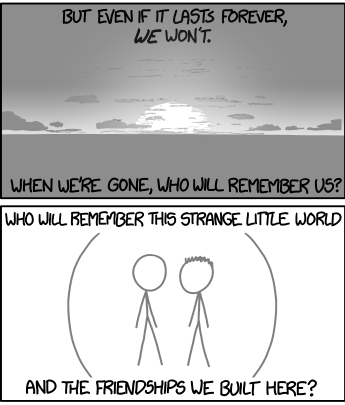
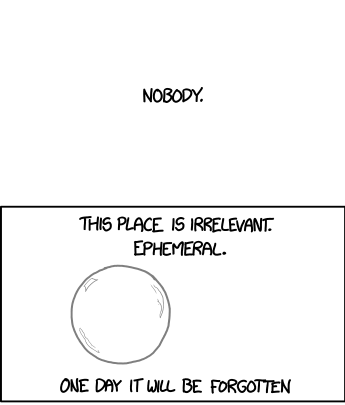
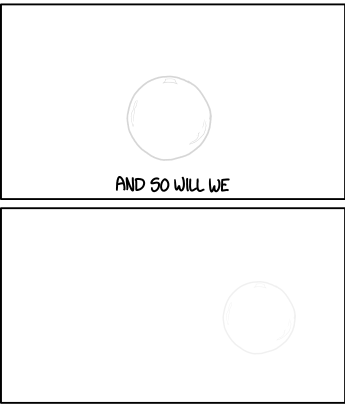
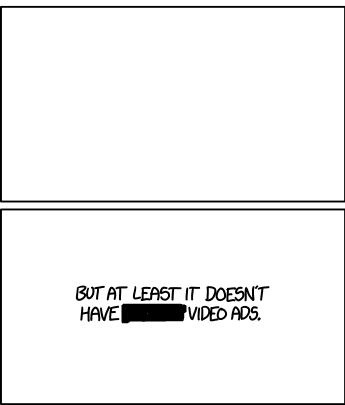
- Raihan142857
-
1000+ posts
JavaScript help ...
It doesn't do the exact same thing in some cases, and it's bad practice. If you use innerText to do one thing people will think it's fine to use it to get the text of an element, or who knows what. It's the same reason why eval is bad practice no matter what.bad in general. Use textContent.Setting innerText or textContent does the same thing. innerText only has performance issues when using it to get the text of an element. inner* isdocument.getElementById("views").textContent = out.views;
I use scratch.
GF: I'll dump you. BF: hex dump or binary dump?
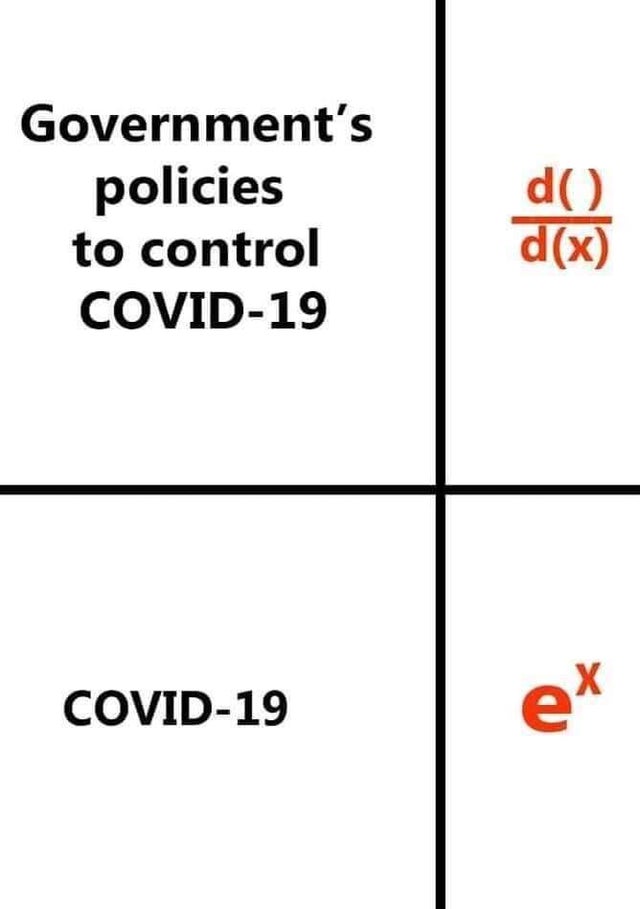
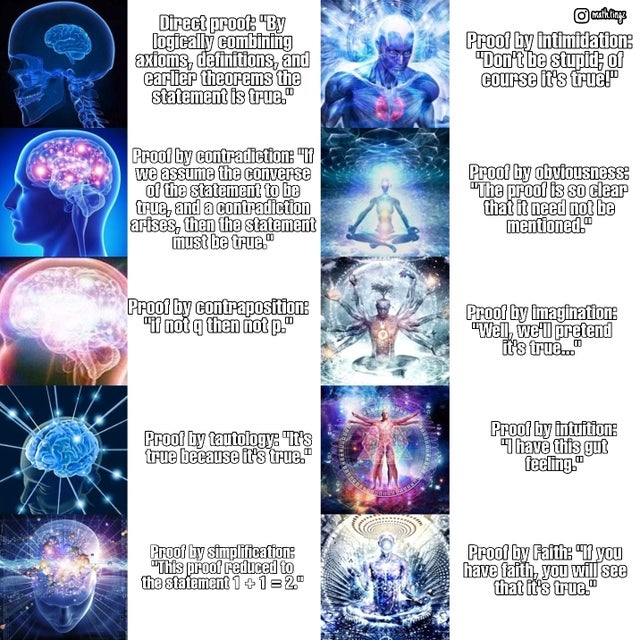
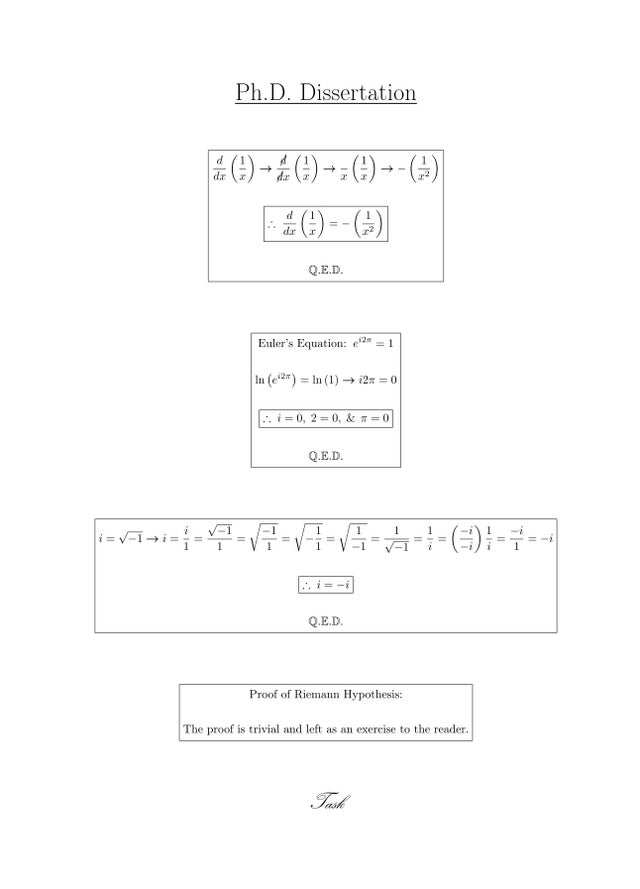
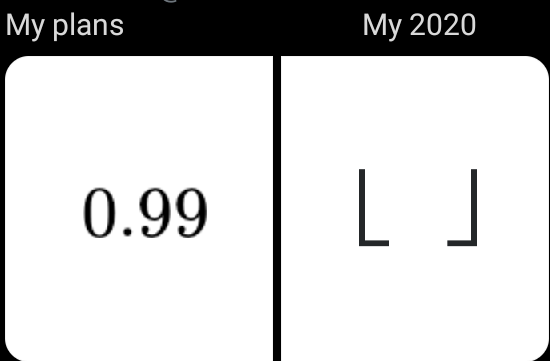
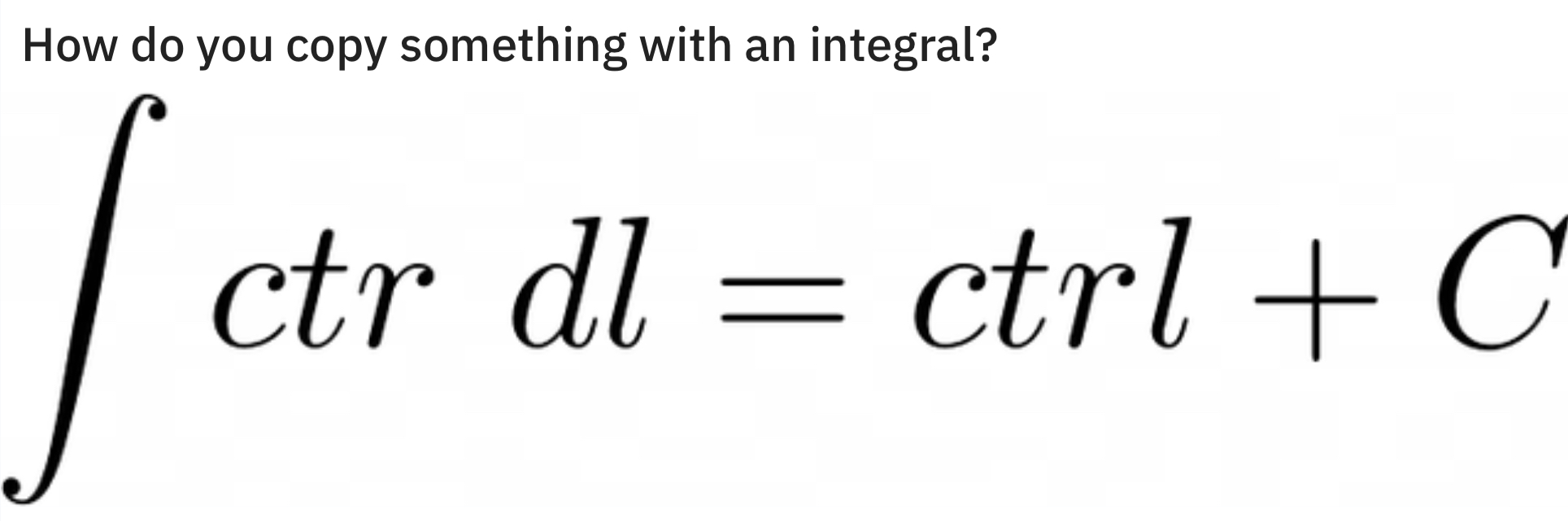
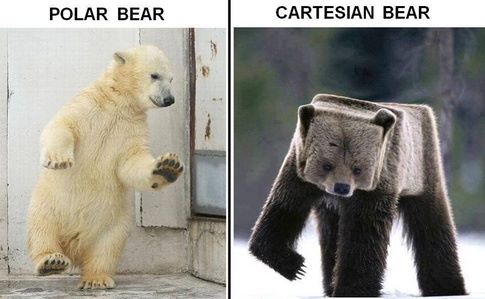
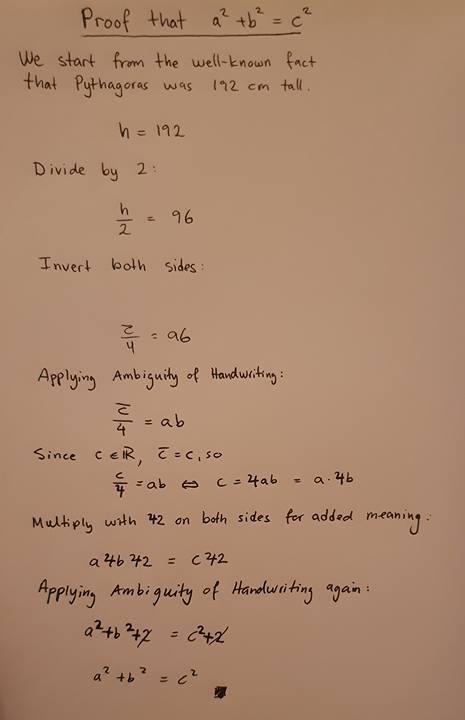
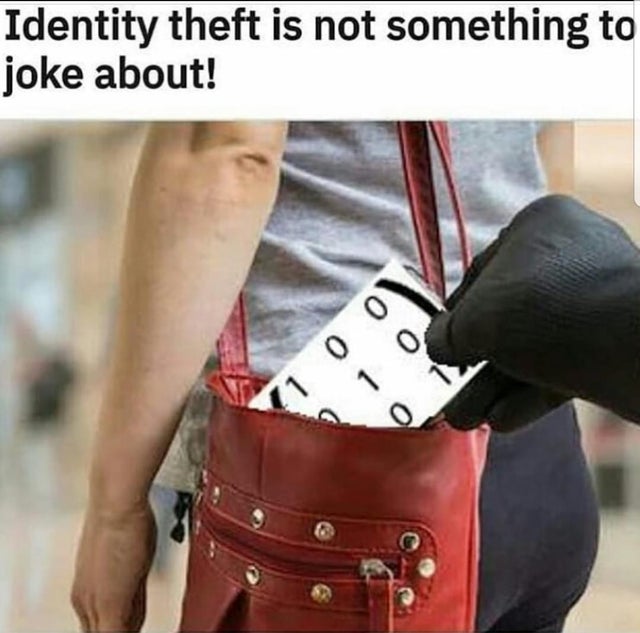
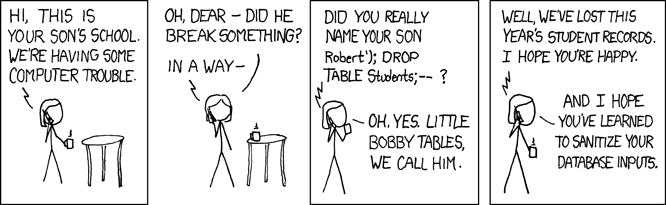
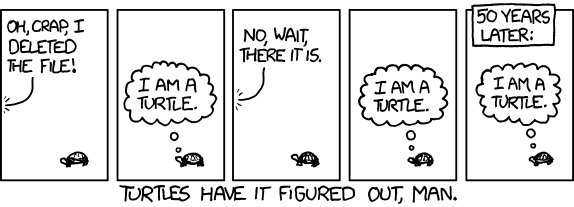
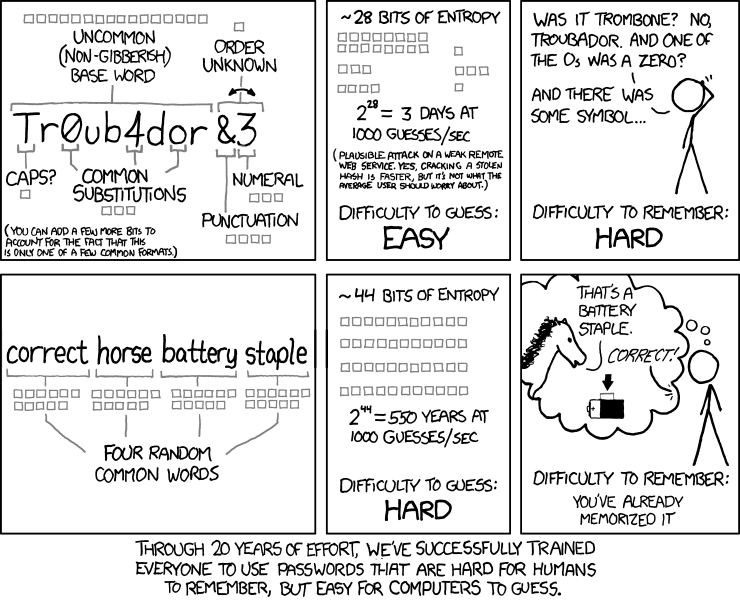
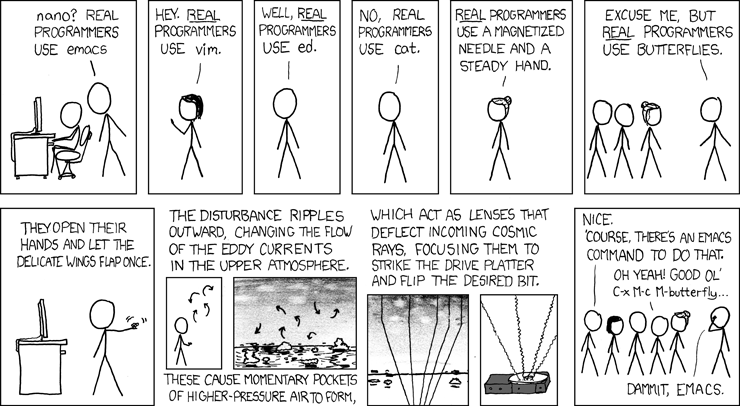
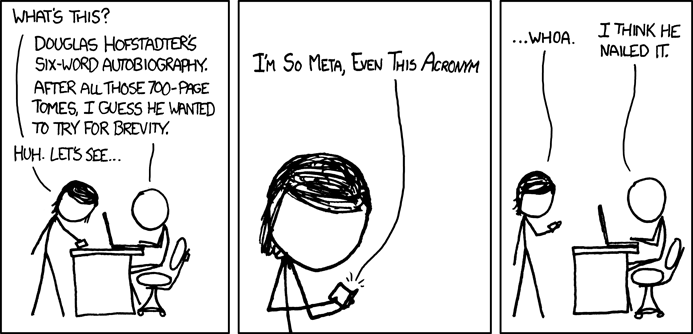
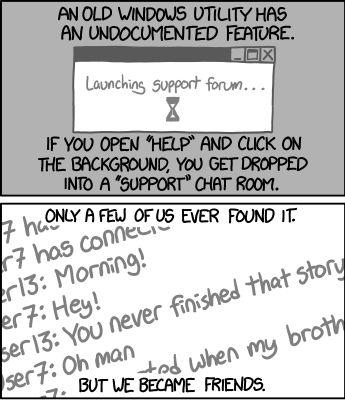
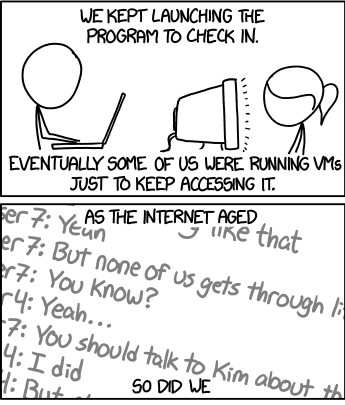
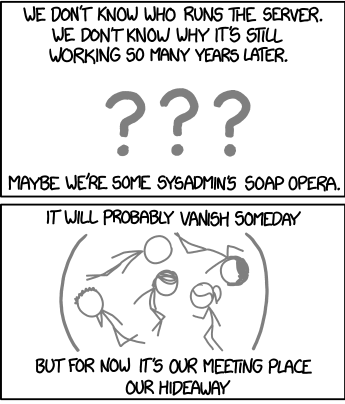
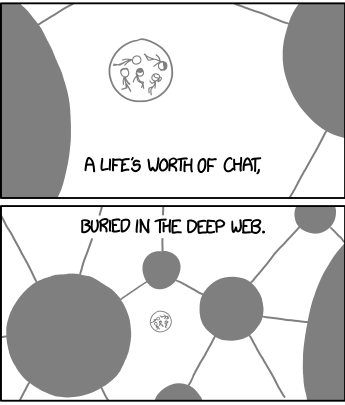
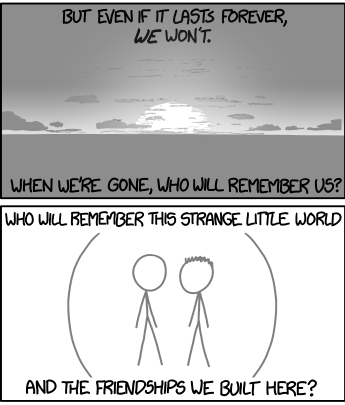
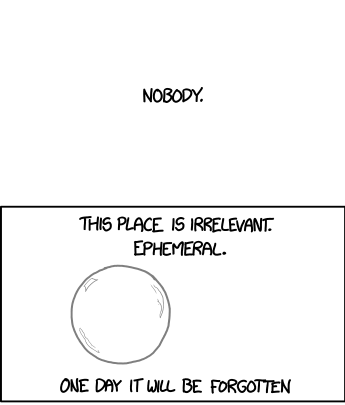
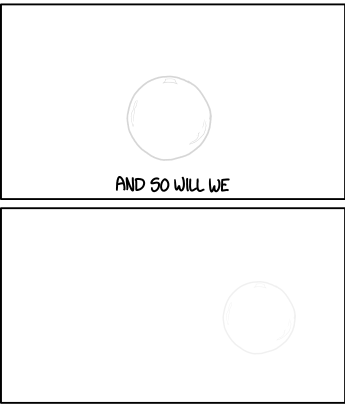
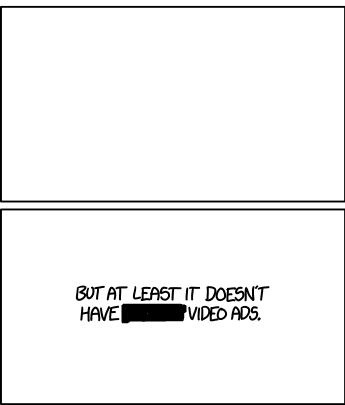
- Sheep_maker
-
1000+ posts
JavaScript help ...
Based on the spec for the setters for textContent and innerText, it seems one difference is their handling of line feed and carriage return characters:It doesn't do the exact same thing in some cases, and it's bad practice. If you use innerText to do one thing people will think it's fine to use it to get the text of an element, or who knows what. It's the same reason why eval is bad practice no matter what.Setting innerText or textContent does the same thing. innerText only has performance issues when using it to get the text of an element. …
document.body.innerText = 'hello\rweee' // Replaces \r with <br> document.body.textContent // === 'helloweee' // vs document.body.textContent = 'hello\rweee' document.body.textContent // === 'hello\rweee'
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- AmazingMech2418
-
1000+ posts
JavaScript help ...
This should be a complete script:
By the way, ignore the red boxes. XD It's a part of JS that I think is newer, but it's just easier to do it that way. LOL!
fetch('https://server.chiroyce.repl.co/views') .then(res => res.json()) .then((out) => { console.log('Output: ', out.views); document.getElementById("views").textContent = `This page has ${out.views} views.`; }).catch(err => console.error(err));
By the way, ignore the red boxes. XD It's a part of JS that I think is newer, but it's just easier to do it that way. LOL!
I'm a programmer, ethical hacker, and space nerd!
Last edited by Neil Armstrong (July 20, 1969 20:17:00)
sam
- Discussion Forums
- » Advanced Topics
-
» JavaScript help ...