Discuss Scratch
- Discussion Forums
- » Things I'm Making and Creating
- » ***Python function changing local variables!
- Mr_Custom
-
100+ posts
***Python function changing local variables!
New Issue!
Here it is!
I am creating a python function that returns all the possible moves that a player can make in one turn in a list of tuples (which represent each board state).
I am running into the issue where the line:
somehow changes the variable ‘board’, which it shouldn't!
The full function is:
An example call is:
Here it is!
I am creating a python function that returns all the possible moves that a player can make in one turn in a list of tuples (which represent each board state).
I am running into the issue where the line:
possible[-1][count1] = turn
The full function is:
def getPossible(board, turn):
possible = []
temp2 = board
while "-" in temp2:
count1 = 0
while temp2[count1] != "-" and count1 < len(temp2)-1:
count1 += 1
possible.append(board)
possible[-1][count1] = turn # << It was that guy
print(board)
return possible
list3 = getPossible(["-", "-", "-", "-", "-", "-", "-", "-", "-"], "X")
Last edited by Mr_Custom (Jan. 4, 2020 14:20:32)
- Sheep_maker
-
1000+ posts
***Python function changing local variables!
I think `possible[-1]` points to the same list in memory as `board`, so modifying it by doing `possible[-1][count1] = turn` will also modify `board`. You can prevent it by cloning `board` before appending it to `possible` so that `possible[-1]` points to a fresh new separate list in memory.
Edit: BBCode absorbed my square brackets! I have fixed.
Edit: BBCode absorbed my square brackets! I have fixed.
Last edited by Sheep_maker (Dec. 26, 2019 20:52:50)
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- Mr_Custom
-
100+ posts
***Python function changing local variables!
I think `possible[-1]` points to the same list in memory as `board`, so modifying it by doing `possible[-1][count1] = turn` will also modify `board`. You can prevent it by cloning `board` before appending it to `possible` so that `possible[-1]` points to a fresh new separate list in memory.
I amended it to this (below) with the same results! Sorry if I didn't understand your answer properly!
def getPossible(board, turn):
possible = []
temp2 = board
while "-" in temp2:
count1 = 0
while temp2[count1] != "-" and count1 < len(temp2)-1:
count1 += 1
temp = board
possible.append(temp)
possible[-1][count1] = turn
print(board)
return possible
- Sheep_maker
-
1000+ posts
***Python function changing local variables!
temp, board, and possible[-1] all refer to the same list in memory, so changing the items in one will affect the others as well (for example, when you do possible[-1][count] = turn, board[count] will also be turn)
Fortunately, there's a way to prevent this. From the original version of your code, if you changed line 8 to
.copy() (as recommended by StackOverflow) stores an exact copy of the list elsewhere in memory so that board and possible[-1] refer to different lists and changing one won't affect the other
Fortunately, there's a way to prevent this. From the original version of your code, if you changed line 8 to
possible.append(board.copy())
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- HPD1155
-
100+ posts
***Python function changing local variables!
you know python tooI think `possible[-1]` points to the same list in memory as `board`, so modifying it by doing `possible[-1][count1] = turn` will also modify `board`. You can prevent it by cloning `board` before appending it to `possible` so that `possible[-1]` points to a fresh new separate list in memory.
I amended it to this (below) with the same results! Sorry if I didn't understand your answer properly!def getPossible(board, turn):
possible = []
temp2 = board
while "-" in temp2:
count1 = 0
while temp2[count1] != "-" and count1 < len(temp2)-1:
count1 += 1
temp = board
possible.append(temp)
possible[-1][count1] = turn
print(board)
return possible
here's an animation code:
import turtle
turtle.shape('turtle')
turtle.color('green')
turtle.pen('green')
turtle.forward(50)
turtle.left(30*30)
turtle.backward(10)
turtle.right(70)
turtle.forward(500*500)
I give you permission to copy the code.
Last edited by HPD1155 (Jan. 2, 2020 21:00:48)
i luv koenigseggs I is a hackr FYI
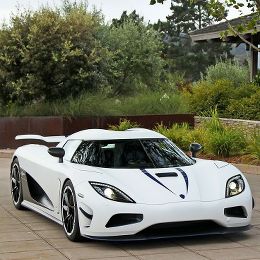
console.log(“hi); I’m an ethical hacker FYI
- Discussion Forums
- » Things I'm Making and Creating
-
» ***Python function changing local variables!