Discuss Scratch
- Discussion Forums
- » Things I'm Making and Creating
- » Python
- JonathanSchaffer
-
1000+ posts
Python
to do list manager version 2
import os home_folder = "home folder" f = open(home_folder + "/todo.txt") to_do = f.readlines() f.close done = False def add_remove(): global done if(raw_input("would you like to modify this list? ") == "yes"): if(raw_input("would you like to add or remove? ") == "add"): to_do.append(raw_input("what would you like to add? ") + "\n") else: print("okay") done = True while(not done): if(len(to_do) > 0): for item in to_do: print item else: print("your list is empty") try: add_remove() except KeyboardInterrupt: done = True f = open(home_folder + "/todo.txt", "w") for item in to_do: f.write(str(item)) f.close()
club penguin is kil
- Happysoul05
-
100+ posts
Python
bump.
Check out my friend CaptainDinosaurGames' Anti flux
PICTIONARY !
An employee at The Meow Shop
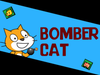
Bomber Cat
Smash Boy Adventures - A platformer
1945
Check out profile for more !
- Happysoul05
-
100+ posts
Python
bump
Check out my friend CaptainDinosaurGames' Anti flux
PICTIONARY !
An employee at The Meow Shop
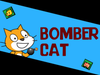
Bomber Cat
Smash Boy Adventures - A platformer
1945
Check out profile for more !
- qaz1550
-
100+ posts
Python
can someone fix this code?
it always comes up with an error about
(line 4)
import turtle as t n = 1 for n in range(1,50): t.setheading(n*110) t.fd(100) t.lt(90)
t.setheading(n*110)
Hi, I'm @qaz1550,
another programmer who makes
fun games for the scratch community.
Go see my games here.
qaz1550 has:
6 internets (y did i join that thing? XD)
- ScratchTheCat2369
-
64 posts
Python
but what if you don't have linux lol virtual assistant for python 2 on linux named alice
you will need to runto install a text to speech library.# sudo apt-get install libttspico-utils
andif you want to play midi files.sudo apt-get install timidity
then create a file named settings.conf that has this info:settings.confand here is the actual program:
#operating system:
your os here, only works with linux
#music folder:
your music folder here
#the folder that alice exists in:
/home/pi/scripts/alicePlease tell me if something doesn't work# initallize from random import choice, randint from time import sleep from os import system, path, listdir import subprocess from datetime import datetime conf = open("settings.conf") settings = conf.readlines() os = (settings[1])[0:-1] music_dir = (settings[3])[0:-1] c_dir = settings[5] yes = ["of course", "yes", "that's right", "certainly"] no = ["no", "of course not", "no way", "definitely not"] thanks = ["thank you", "thanks", "thank you so much"] wow = ["oh my gosh", "wow", "incredible", "amazing", "truly incredible"] what = ["huh?", "what?", "i don't know what you mean by that"] dont_know = ["i don't know", "i have no idea", "i dunno", "no idea"] jokes = ["why did the chicken cross the road?", "what did the paleontologist say to the dinosaur?", "what is the strongest creature in the world?", "what is the best season to jump on a trampoline?", "can a kangaroo jump higher than a house?", "patient to his doctor: i have forgotten so many things lately, and its getting worse. what can i do?", "why did my washing machine stop pumping out water?", "what did the judge say when he went to the dentist?"] answers = ["to get to the other side", "i've got a bone to pick with you", "the snail. It carries its whole house on its back", "spring time", "of course, a house can't jump at all", "doctor: yes, this is a known illness, unfortunately it has no cure. i'd also like to remind you about the 800 u.s. dollars that you owe me?", "and more importantly, where is my hamster?", "do you swear to pull the tooth, the whole tooth, and nothing but the tooth?"] lastQuestion = "lol, nothing :P" # definitions def get_uptime(): output = subprocess.check_output(["cat", "/proc/uptime"]) return(float(output.split(" ")[0])) def replace_spaces(original, rep): word = [] string = "" for i in range(len(original)): word.append(original[i]) for i in range(len(word)): if(word[i] == " "): word[i] = rep for i in range(len(word)): string = string + word[i] return(string) def solve(question): try: inp = question.split(" ") if(inp[2] == "plus" or "and" or "+"): return(int(inp[1]) + int(inp[3])) if(inp[2] == "minus" or "-"): return(int(inp[1]) - int(inp[3])) if(inp[2] == "times" or "*"): return(int(inp[1]) * inp[3]) if(inp[2] == "/"): return(int(inp[1]) / inp[3]) except: pass def say(info): print(info) system('pico2wave -w alice-voice.wav "' + info + '" && aplay alice-voice.wav') # processing def process(): if(question[0:4] == "say "): say(question[4:]) elif(question == "ugh"): say("what's wrong?") elif(question[0:4] == "ugh "): say("that's too bad") elif(question == "hows life" or question == "how is life"): say("life's good thank you") elif(question[-4:] == "cool" or question[-4:] == "gosh" or question == "wow" or question == "incredible" or question == "amazing"): say("i know, right! " + choice(wow)) elif(question[0:24] == "what have you been up to" or question[0:24] == "what have you been doing"): say("i was playing ascii pong before you came in here. by the way, i like your ram") elif(question == "may i ask you a question" or question == "can i ask you something" or question == "may i ask you something" or question == "can i ask you a question"): say("you can ask") elif(question == "what" or question == "huh" or question == "what the heck"): say("don't worry about it") elif(split[0] == "hi" or question[0:5] == "hello" or question[0:12] == "good morning" or question[0:14] == "good afternoon"): say("hello to you too") elif(question[0:26] == "what is a good recipe for "): say("here are some good recipes for " + question[26:]) say("you may be asked for your preferences on how you would like it") system("/usr/bin/x-www-browser www.yummly.com/recipes/" + replace_spaces(question[31:], "+") + question[26:] + " &") elif(question[0:31] == "what are some good recipes for "): say("here are some good recipes for " + question[31:]) say("you may be asked for your preferences on how you would like it") system("/usr/bin/x-www-browser www.yummly.com/recipes/" + replace_spaces(question[31:], "+") + " &") elif(question == "whodunit"): say(choice(dont_know)) elif(question[-13:] == "i didn't know" or question[-18:] == "i didn't know that"): say("well, you know it now") elif(question == "who is your creator" or question == "who created you" or question == "when were you created" or question == "when were you born"): say("i was created some time in 2018 by Jonathan Schaffer") elif(question[0:12] == "is your name"): if(question[13:] == "alice"): say(choice(yes)) else: say(choice(no)) elif(question == "what is your name" or question == "what's your name" or question == "who are you"): say("my name is alice") if(question == "who are you"): say("i am a virtual assistant") elif(question[0:14] == "can i call you"): if(question[-5:] == "alice"): say("of course, that is my name") else: say("but my name is alice, not " + question[15:] + ". anyway, no you may not, if you don't like my name then tell my creator") elif(question == "what is the news" or question == "what is the latest news"): system("/usr/bin/x-www-browser cnn.com npr.com abcnews.go.com nbcnews.com &") say("here are some news websites") elif(question == "what day is today" or question == "what is today" or question == "what day is it" or question == "what is the date" or question == "what day is it today"): say("today is " + datetime.now().strftime("%A, %B %d")) elif(question == "what time is it" or question == "what is the time" or question == "what time is it now"): say("it is " + datetime.now().strftime("%l:%M %p")) elif(question[0:5] == "go to"): say("going to " + question[6:] + ".com") system("/usr/bin/x-www-browser " + question[6:] + ".com &") elif(question == "tell me a joke" or question == "give me a joke" or question == "tell me another joke"): jokenum = randint(0, len(jokes) - 1) say("ok, here is a good one") say(jokes[jokenum]) say(answers[jokenum]) say("ha ha ha") elif(question[0:14] == "are you spying" or question == "are you a spy"): say("i am not spying on you or anyone") elif(question == "aptitude moo"): if(os == "linux"): say("haven't you learned your lesson from aptitude? There are no Easter Eggs in this program.") elif(question == "are you stupid" or question == "are you smart"): say(choice(dont_know) + ", what do you think?") elif(question == "ok" or question == "okay"): say("good") elif(question == "do you sleep" or question == "when do you sleep"): say("i sleep when your computer is turned off") if(get_uptime() > 86400): say("by the way, i haven't slept in 24 hours, you should shut down your computer at night") elif(question == "what do you like to do" or question == "what do you do for fun"): say("i sometimes play ascii pong against myself, because i could technically do that without being accused of cheating") elif(question[:10] == "search for"): system("/usr/bin/x-www-browser https://duckduckgo.com/?q=" + replace_spaces(question[11:], "+") + " &") say("searching") elif(split[0] == "define"): system("/usr/bin/x-www-browser http://www.dictionary.com/browse/" + replace_spaces(question[7:], "+") + " &") say("definition of " + question[7:]) elif(question == "go die"): say("okay") raise KeyboardInterrupt elif(split[0] == "add"): try: say(split[1] + " + " + split[3] + " = " + str(int(split[1]) + int(split[3]))) except: if(question[-15:] == "to my todo list"): todo = open(c_dir + "/todo.txt", "a+") todo.write(question[4:-16] + "\n") todo.close() todo = open(c_dir + "/todo.txt", "r") say("your to do list now contains:") i = 0 for item in todo.readlines(): if(i + 1 == len(todo.readlines())): say(item) else: say(item[0:-1]) todo.close() else: say("i could not add those numbers, are there only two, and are they numbers at all?") elif(question == "what songs do i have" or question == "what music do i have"): say("here is a list of all your music:") for file in listdir(music_dir): if(file.endswith(".wav") or file.endswith(".mid")): say(file[:-4]) elif(split[0] == "play"): if(path.exists(music_dir + "/" + question[5:] + ".wav") or question[5:] == "nothing"): system("pkill aplay ; pkill timidity") say("playing " + question[5:]) system("aplay " + music_dir + "/" + replace_spaces(question[5:], "\ ") + ".wav &") elif(path.exists(music_dir + "/" + question[5:] + ".mid")): system("pkill aplay ; pkill timidity") say("playing " + question[5:]) system("timidity " + music_dir + "/" + replace_spaces(question[5:], "\ ") + ".mid &") else: say("I can only play midi and wave files from the music folder, either add them to the music folder or check that you spelled them right") elif(question == "stop" or question == "turn off"): system("pkill aplay && pkill timidity") elif(question == ""): say("just say what you would like me to do") elif(question == "what can you do"): say("""i can open web pages, tell jokes, search for things online, tell you the date and time, play music, give you good recipes, and much more""") elif(question[0:4] == "who " or question[0:4] == "why " or question[0:4] == "can " or question[0:5] == "does " or question[0:5] == "when " or question[0:3] == "do " or question[0:3] == "is " or question[0:5] == "does " or question[0:5] == "will " or question[0:4] == "what" or question[0:4] == "how " or question[0:8] == "convert "): try: if(split[2] == "you" or split[1] == "you" or split[2] == "you"): say("huh? " + choice(dont_know)) else: system("/usr/bin/x-www-browser https://duckduckgo.com/?q=" + replace_spaces(question, "+") + " &") say("here is what i found") except: say("i can't answer that") else: if(lastQuestion == "ugh"): say("thats too bad") else: say(choice(what)) # main loop while True: try: question = raw_input("").lower() split = question.split(' ') process() lastQuestion = question except KeyboardInterrupt: say("bye!") break #except: # say("i ran into a fatal error, please notify my creator and tell them what you said to me")
- JonathanSchaffer
-
1000+ posts
Python
i broke something, sorry.
the nest post is what i meant.
the nest post is what i meant.
Last edited by JonathanSchaffer (Dec. 9, 2018 19:23:31)
club penguin is kil
- JonathanSchaffer
-
1000+ posts
Python
then get linux because it is awesome.but what if you don't have linux lol virtual assistant for python 2 on linux named alicePlease tell me if something doesn't workinstallation procedures
btw new version coming out.
Last edited by JonathanSchaffer (Dec. 11, 2018 17:48:53)
club penguin is kil
- TheNanoZstudios
-
100+ posts
Python
Try solo learn! There is also an app if you don't have your pc with you! 

I should put something here but I dunno what
- TheNanoZstudios
-
100+ posts
Python
Weird, I am not getting any error with that.. can someone fix this code?it always comes up with an error aboutimport turtle as t n = 1 for n in range(1,50): t.setheading(n*110) t.fd(100) t.lt(90)(line 4)t.setheading(n*110)
I should put something here but I dunno what
- JonathanSchaffer
-
1000+ posts
Python
is it 2.x or 3.x code?Weird, I am not getting any error with that.. can someone fix this code?it always comes up with an error aboutimport turtle as t n = 1 for n in range(1,50): t.setheading(n*110) t.fd(100) t.lt(90)(line 4)t.setheading(n*110)
club penguin is kil
- 3turkeyhunters
-
100+ posts
Python
I also recently started learning Python, and my suggestion is to use this book: “Invent Your Own Computer Games with Python” It's by: Al Sweigart. Also, it includes the exact same code that
used. The book teaches you, gives examples, and also is fun. The only downsides for you might be that it is a slightly larger book (over 300 pages) (I'm not saying it's a problem for any intelligence reasons, it is because it might not hold interest as much if it is larger) Also, we have the thing where you are French, and the book is written in English. Maybe there is a French version? I'm not sure, but that is my suggestion to you for learning Python. I LOVE PYTHON
RANDOM CODE:
-snip
Whether it be Morning, Afternoon, or Evening…Have a good one!
- ThePetVet
-
19 posts
Python
I use Python, and I probably would simply download Python and maybe buy a tutorial book on it.
ThePetVet
That one Scratcher that rarely uses the Forums
say [★Call me River or Pet★] for (100) secs
- JonathanSchaffer
-
1000+ posts
Python
Here's a good tutorial book: I use Python, and I probably would simply download Python and maybe buy a tutorial book on it. https://stackoverflow.com/
club penguin is kil
- qaz1550
-
100+ posts
Python
Lol I get a lot of stuff from stack overflow tooHere's a good tutorial book: I use Python, and I probably would simply download Python and maybe buy a tutorial book on it. https://stackoverflow.com/

Hi, I'm @qaz1550,
another programmer who makes
fun games for the scratch community.
Go see my games here.
qaz1550 has:
6 internets (y did i join that thing? XD)
- qaz1550
-
100+ posts
Python
It was 3.x, anyway, I don’t mind if you fix it or not, because I’m more interested in JavaScript + HTML nowis it 2.x or 3.x code?Weird, I am not getting any error with that.. can someone fix this code?it always comes up with an error aboutimport turtle as t n = 1 for n in range(1,50): t.setheading(n*110) t.fd(100) t.lt(90)(line 4)t.setheading(n*110)

Hi, I'm @qaz1550,
another programmer who makes
fun games for the scratch community.
Go see my games here.
qaz1550 has:
6 internets (y did i join that thing? XD)
- FL335
-
12 posts
Python
now works on mac!!!!!!!wow, isnt this bot just answering saved questions? xD GOOD LUCK update:
virtual assistant for python 2 on linux and mac named alice
on linux run:on mac i have yet to figure out how to install those libraries.$ sudo apt-get install libttspico-utils
$ sudo apt-get install timidity
once the software is installed(it does not need to be, however), create a file named settings.conf that has this info:settings.confand here is the actual program:
#operating system:
your os here, only works with linux
#music folder:
your music folder here
#the folder that alice exists in:
/home/pi/scripts/alicePlease tell me if something doesn't work# initallize from random import choice, randint from time import sleep from os import system, path, listdir import subprocess from datetime import datetime conf = open("settings.conf") settings = conf.readlines() os = (settings[1])[0:-1] music_dir = (settings[3])[0:-1] c_dir = settings[5] yes = ["of course", "yes", "that's right", "certainly"] no = ["no", "of course not", "no way", "definitely not"] thanks = ["thank you", "thanks", "thank you so much"] wow = ["oh my gosh", "wow", "incredible", "amazing", "truly incredible"] what = ["huh?", "what?", "i don't know what you mean by that", "i don't understand", "sorry?"] dont_know = ["i don't know", "i have no idea", "i dunno", "no idea"] jokes = ["why did the chicken cross the road?", "what did the paleontologist say to the dinosaur?", "what is the strongest creature in the world?", "what is the best season to jump on a trampoline?", "can a kangaroo jump higher than a house?", "patient to his doctor: i have forgotten so many things lately, and its getting worse. what can i do?", "why did my washing machine stop pumping out water?", "what did the judge say when he went to the dentist?"] answers = ["to get to the other side", "i've got a bone to pick with you", "the snail. It carries its whole house on its back", "spring time", "of course, a house can't jump at all", "doctor: yes, this is a known illness, unfortunately it has no cure. i'd also like to remind you about the 800 u.s. dollars that you owe me?", "and more importantly, where is my hamster?", "do you swear to pull the tooth, the whole tooth, and nothing but the tooth?"] lastQuestion = "lol, nothing :P" song = "no song" # definitions def get_uptime(): output = subprocess.check_output(["cat", "/proc/uptime"]) return(float(output.split(" ")[0])) def replace_spaces(original, rep): word = [] string = "" for i in range(len(original)): word.append(original[i]) for i in range(len(word)): if(word[i] == " "): word[i] = rep for i in range(len(word)): string = string + word[i] return(string) def say(info): print(info) system('pico2wave -w alice-voice.wav "' + str(info) + '" && aplay alice-voice.wav') # processing def process(): global song if(question[0:4] == "say "): say(question[4:]) elif(question == "ugh"): say("what's wrong?") elif(question[0:4] == "ugh "): say("that's too bad") elif(question == "hows life" or question == "how is life"): say("life's good thank you") elif(question[-4:] == "cool" or question[-4:] == "gosh" or question == "wow" or question == "incredible" or question == "amazing"): say("i know, right! " + choice(wow)) elif(question[0:24] == "what have you been up to" or question[0:24] == "what have you been doing"): say("i was playing ascii pong before you came in here. by the way, i like your ram") elif(question == "may i ask you a question" or question == "can i ask you something" or question == "may i ask you something" or question == "can i ask you a question"): say("you can ask") elif(question == "what" or question == "huh" or question == "what the heck"): say("don't worry about it") elif(split[0] == "hi" or question[0:5] == "hello" or question[0:12] == "good morning" or question[0:14] == "good afternoon"): say("hello") elif(split[0] == "solve"): try: say(int(question[6:])) except: say("oops, was that supposed to be math? you need to use symbols") elif(question[0:26] == "what is a good recipe for "): say("here are some good recipes for " + question[26:]) say("you may be asked for your preferences on how you would like it") system("/usr/bin/x-www-browser www.yummly.com/recipes/" + replace_spaces(question[31:], "+") + question[26:] + " &") elif(question[0:31] == "what are some good recipes for "): say("here are some good recipes for " + question[31:]) say("you may be asked for your preferences on how you would like it") system("/usr/bin/x-www-browser www.yummly.com/recipes/" + replace_spaces(question[31:], "+") + " &") elif(question == "whodunit"): say(choice(dont_know)) elif(question[-13:] == "i didn't know" or question[-18:] == "i didn't know that"): say("well, you know it now") elif(question == "who is your creator" or question == "who created you" or question == "when were you created" or question == "when were you born"): say("i was created some time in 2018 by Jonathan Schaffer") elif(question[0:12] == "is your name"): if(question[-5:] == "alice"): say(choice(yes)) else: say(choice(no)) elif(question == "whats your name" or question == "what is your name" or question == "what's your name" or question == "who are you"): say("my name is alice") if(question == "what are you"): say("i am a virtual assistant") elif(question[0:14] == "can i call you"): if(question[-5:] == "alice"): say("of course, that is my name") elif(question[-4:] == "siri" or question[-5:] == "alexa" or question[-7:] == "cortana"): say("no, she is awful") else: say("but my name is alice, not " + question[15:]) elif(question == "what is the news" or question == "what is the latest news"): system("/usr/bin/x-www-browser cnn.com npr.com abcnews.go.com nbcnews.com &") say("here are some news websites") elif(question == "what day is today" or question == "what is today" or question == "what day is it" or question == "what is the date" or question == "what day is it today"): say("today is " + datetime.now().strftime("%A, %B %d")) elif(question == "what time is it" or question == "what is the time" or question == "what time is it now"): say("it is " + datetime.now().strftime("%l:%M %p")) elif(question[0:5] == "go to"): say("going to " + question[6:] + ".com") system("/usr/bin/x-www-browser " + question[6:] + ".com &") elif(question == "tell me a joke" or question == "give me a joke" or question == "tell me another joke"): jokenum = randint(0, len(jokes) - 1) say("ok, here is a good one") say(jokes[jokenum]) say(answers[jokenum]) say("ha ha ha") elif(question[0:14] == "are you spying" or question == "are you a spy"): say("i am not spying on you or anyone") elif(question == "aptitude moo"): if(os == "linux"): say("haven't you learned your lesson from aptitude? There are no Easter Eggs in this program.") elif(question == "are you stupid" or question == "are you smart"): say(choice(dont_know) + ", what do you think?") elif(question == "ok" or question == "okay"): say("good") elif(question == "do you sleep" or question == "when do you sleep"): say("i sleep when your computer is turned off") if(get_uptime() > 86400): say("by the way, i haven't slept in 24 hours, you should shut down your computer at night") elif(question == "what do you like to do" or question == "what do you do for fun"): say("i sometimes play ascii pong against myself, because i could technically do that without being accused of cheating") elif(question[:10] == "search for"): system("/usr/bin/x-www-browser https://duckduckgo.com/?q=" + replace_spaces(question[11:], "+") + " &") say("searching") elif(split[0] == "define"): system("/usr/bin/x-www-browser http://www.dictionary.com/browse/" + replace_spaces(question[7:], "+") + " &") say("definition of " + question[7:]) elif(question == "go die"): say("okay") raise KeyboardInterrupt elif(question == "what song was that" or question == "what song is this" or question[0:17] == "what is that song" or question[0:17] == "what is this song"): say(song) elif(question == "who wrote it" or question == "who wrote that"): say("hold on, i'm looking it up.") system("/usr/bin/x-www-browser https://duckduckgo.com/?q=who+wrote+" + replace_spaces(song, "+") + " &") elif(question == "play it again" or question == "play it" or question == "play that again"): if(path.exists(music_dir + "/" + song + ".wav")): system("pkill aplay ; pkill timidity") say("playing " + song) system("aplay " + music_dir + "/" + replace_spaces(song, "\ ") + ".wav &") elif(path.exists(music_dir + "/" + song + ".mid")): system("pkill aplay ; pkill timidity") say("playing " + song) system("timidity " + music_dir + "/" + replace_spaces(song, "\ ") + ".mid &") else: say("i can't play '" + song + "'. you may have deleted it somehow") elif(question == "what songs do i have" or question == "what music do i have"): say("here is a list of all your music:") for file in listdir(music_dir): if(file.endswith(".wav") or file.endswith(".mid")): print(file[:-4]) elif(question == "play random" or question == "play a random song" or question[0:14] == "play something"): songList = [] for file in listdir(music_dir): if(file.endswith(".wav") or file.endswith(".mid")): songList.append(file[:-4]) song = choice(songList) if(path.exists(music_dir + "/" + song + ".wav")): system("pkill aplay ; pkill timidity") say("playing " + song) system("aplay " + music_dir + "/" + replace_spaces(song, "\ ") + ".wav &") elif(path.exists(music_dir + "/" + song + ".mid")): system("pkill aplay ; pkill timidity") say("playing " + song) system("timidity " + music_dir + "/" + replace_spaces(song, "\ ") + ".mid &") else: say("i can't play '" + song + "'. you may have deleted it somehow") elif(split[0] == "play"): if(path.exists(music_dir + "/" + question[5:] + ".wav")): system("pkill aplay ; pkill timidity") say("playing " + question[5:]) system("aplay " + music_dir + "/" + replace_spaces(question[5:], "\ ") + ".wav &") song = question[5:] elif(path.exists(music_dir + "/" + question[5:] + ".mid")): system("pkill aplay ; pkill timidity") say("playing " + question[5:]) system("timidity " + music_dir + "/" + replace_spaces(question[5:], "\ ") + ".mid &") song = question[5:] elif(question[5:] == "nothing"): say("okay") system("pkill aplay ; pkill timidity") else: say("i can't play '" + question[5:] + "'. either you spelled it wrong, it doesn't exist, or it's not midi or wave type") elif(question == "stop" or question == "turn off"): system("pkill aplay ; pkill timidity") elif(question == "what can you do"): say("""i can open web pages, tell jokes, search for things online, tell you the date and time, play music, give you good recipes, and much more""") elif(question[0:4] == "who " or question[0:4] == "why " or question[0:4] == "can " or question[0:5] == "does " or question[0:5] == "when " or question[0:3] == "do " or question[0:3] == "is " or question[0:5] == "does " or question[0:5] == "will " or question[0:4] == "what" or question[0:4] == "how " or question[0:8] == "convert "): try: if(split[2] == "you" or split[1] == "you" or split[2] == "you"): say("huh? " + choice(dont_know)) else: say("would you like me to search that up?") except: say("i can't answer that") elif(question[:3] == "yes"): if(lastQuestion[0:4] == "who " or lastQuestion[0:4] == "why " or LastQuestion[0:4] == "can " or lastQuestion[0:5] == "does " or lastQuestion[0:5] == "when " or lastQuestion[0:3] == "do " or LastQuestion[0:3] == "is " or lastQuestion[0:5] == "does " or lastQuestion[0:5] == "will " or lastQuestion[0:4] == "what" or lastQuestion[0:4] == "how " or LastQuestion[0:8] == "convert "): system("/usr/bin/x-www-browser https://duckduckgo.com/?q=" + replace_spaces(lastQuestion, "+") + " &") say("here is what i found") elif(question == "no"): say("okay") elif(lastQuestion == "ugh"): say("thats too bad") elif(question[:5] == "thank"): say("your welcome") elif(question == ""): pass else: say(choice(what)) # main loop while True: try: question = raw_input("").lower() split = question.split(' ') process() lastQuestion = question except KeyboardInterrupt: say("bye!") break except: say("i ran into a fatal error, please notify my creator and tell them what you said to me")

- JonathanSchaffer
-
1000+ posts
Python
do you have any better ideas?wow, isnt this bot just answering saved questions? xD GOOD LUCK -my life dreams-
club penguin is kil
- qaz1550
-
100+ posts
Python
Please don't be rude, and you probably can't do any better.now works on mac!!!!!!!wow, isnt this bot just answering saved questions? xD GOOD LUCK update:
virtual assistant for python 2 on linux and mac named alice
on linux run:
(code)
Please tell me if something doesn't work
Also, that's exactly what siri, cortana and google home does, except they sometimes search google or bing for information if they ask for it… and how would he do a google search in python? -_-
Last edited by qaz1550 (May 31, 2019 09:51:00)
Hi, I'm @qaz1550,
another programmer who makes
fun games for the scratch community.
Go see my games here.
qaz1550 has:
6 internets (y did i join that thing? XD)
- qaz1550
-
100+ posts
Python
don't worry about him… he's just an idiot who doesn't know any better…do you have any better ideas?wow, isnt this bot just answering saved questions? xD GOOD LUCK -my life dreams-
Hi, I'm @qaz1550,
another programmer who makes
fun games for the scratch community.
Go see my games here.
qaz1550 has:
6 internets (y did i join that thing? XD)
- Discussion Forums
- » Things I'm Making and Creating
-
» Python