Discuss Scratch
- Discussion Forums
- » Developing Scratch Extensions
- » Creating extensions for Scratch 3.0
- ManRa36
-
12 posts
Creating extensions for Scratch 3.0
Im Really Confused with this
Its Wierd


- ianiiaannn
-
1 post
Creating extensions for Scratch 3.0
Is it possible to make “Choose an extension” button with some javascript that can be paste in F12 console?
- MAS313
-
1 post
Creating extensions for Scratch 3.0
That is a modified version of Scratch made to load extensions from a URL. Hello,
How can I load an extension in scratch-gui by launching
my_web_site/scratch-gui/?url=https://…
For example,
https://sheeptester.github.io/scratch-gui/?url=https://nitrocipher.github.io/ext2to3/ext-test.js
website loads the dynamic extension from another web page.
But when I build scratch-gui and run it, and give my url as a parameter,
it does not load the extension from the webpage.
this does not change also when I run the both the extension site and scratch-gui under https or http
do I need to run scratch-gui with some different parameter in order to load extensions from URL by giving it as a parameter?
Best Regards,
Here are the changes that were made to make that possible:
- Changes in the GUI
- Changes in the VM
I have followed https://medium.com/@hiroyuki.osaki/how-to-develop-your-own-block-for-scratch-3-0-1b5892026421 to add an external extension to my clone version of scratch-vm. It is working fine.
but i have multiple users and wanted each user to load their own extension. so i wanted to apply modification by sheeptester to pass the block url. It is working fine when testing it on nitrocipher extension. However, it is not working for my extension. I think the error is stemming from:
var nets = require(“nets”)
Would appreciate it if you can have a look and let me know whats wrong.
my working extension using scratch-vm: https://github.com/MariamQusai/del/blob/master/z1.js
my extension using scratch-gui (notworking): https://github.com/MariamQusai/del/blob/master/z5.js
Last edited by MAS313 (March 2, 2020 06:39:45)
- Sheep_maker
-
1000+ posts
Creating extensions for Scratch 3.0
@MAS313 Extensions loaded from a URL are run directly inside a web worker, so they do not have access to the `require` function. However, if you include it directly within scratch-vm by modifying the Scratch files, it works because webpack will substitute it with something that works on the web when compiling it.
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- ScratchMasterScott
-
72 posts
Creating extensions for Scratch 3.0
wow complicatedCreating extensions for Scratch 3.0There is now official documentation regarding extensions!, but feel free to use this post to get you started
You'll want to start off by creating your extension's class, and register the extension - In my case, this would be ‘NitroBlock’class NitroBlock { //In both instances, NitroBlock will be the name in both instances } Scratch.extensions.register(new NitroBlock());
Next, we will be constructing block and menu definitions - We will continue to use ‘NitroBlock’ through this tutorialgetInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [ ], "menus": { //we will get back to this in a later tutorial } }; }
We are going to take a look at how blocks are constructed
For those of you that are familiar with extensions for Scratch 2.0, we will start off with this: - If not, you can ignore this['r', 'letters %n through %n of %s', 'substringy', '2', '5', 'hello world'] //breakdown below: ['r' = block type, 'letters %n through %n of %s' = block text, 'substringy' = block ID/opcode]{ "opcode": "substringy", //This will be the ID code for the block "blockType": "reporter", //This can either be Boolean, reporter, command, or hat "text": "letters [num1] through [num2] of [string]", //This is the block text, and how it will display in the Scratch interface "arguments": { //Arguments are the input fields in the block. In the block text, place arguments in square brackets with the corresponding ID "num1": { //This is the ID for your argument "type": "number", //This can be either Boolean, number, or string "defaultValue": "2" //This is the default text that will appear in the input field, you can leave this blank if you wish }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } },
We will put this newly constructed code into the blocks object above - My code will now look like thisclass NitroBlock { getInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [{ "opcode": "substringy", "blockType": "reporter", "text": "letters [num1] through [num2] of [string]", "arguments": { "num1": { "type": "number", "defaultValue": "2" }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } }, }], "menus": { //we will get back to this in a later tutorial } }; } Scratch.extensions.register(new NitroBlock());
Next we come to the most important part, the code that actually runs the blocks! - I am keeping this short and simple for tutorial's sake.//Make sure you name this function with with the proper ID for the block you defined above substringy({num1, num2, string}) { //these names will match the argument names you used earlier, and will be used as the variables in your code //this code can be anything you want return string.substring(num1 - 1, num2); //for reporters and Boolean blocks the important thing is to use 'return' to get the value back into Scratch. }
Place this new code below your getInfo() functionclass NitroBlock { getInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [{ "opcode": "substringy", "blockType": "reporter", "text": "letters [num1] through [num2] of [string]", "arguments": { "num1": { "type": "number", "defaultValue": "2" }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } }, }], "menus": { //we will get back to this in a later tutorial } }; substringy({num1, num2, string}) { return string.substring(num1 - 1, num2); }; }
Save this code to your computer as a js file, in my case it is NitroBlock_3.js
Congratz, you now have your extension code created!!
You can use this tutorial to add it your own personal copy of scratch
Or, you can host the extension with gh-pages, and go here to test it (provide your own url, don't use mine)
Here is the archived copy of my original post
hi! this is my signature. i like destroying thinks, making things and playing video games.
yeah idk what to put here
NEW GAME I'M WORKING ON! PLEASE TRY IT OUT! create your own platformer https://scratch.mit.edu/projects/368825774 please try, i'm looking for feedback
- Not_Kaif
-
4 posts
Creating extensions for Scratch 3.0
You could make your own Scratch Extensions? Can you use the extensions in actual Scratch games and then publish a Scratch game with a custom extension to the Scratch website? 

move (69) steps
- 1800_m1necr4ft
-
21 posts
Creating extensions for Scratch 3.0
what about an extention that allows more blocks? https://scratch.mit.edu/discuss/topic/389419/?page=1#post-3868586
show list [ things that describe me]
// |
//\|/
1: Ten-year-old Minecraft lover
2: technology enthusiast
3: trying to figure out how to get garageband on the raspberry pi that i am using right now
- Not_Kaif
-
4 posts
Creating extensions for Scratch 3.0
These are what I would want as extensions!:
if on sprite[], bounce
randomblock<only if <>>
Last edited by Not_Kaif (March 12, 2020 18:58:32)
- Not_Kaif
-
4 posts
Creating extensions for Scratch 3.0
the only if block kinda relates to: These are what I would want as extensions!:if on sprite[], bouncerandomblock<only if <>>
if <> thenso yeah
end
- Boomer001
-
1000+ posts
Creating extensions for Scratch 3.0
I have this code, and when i go to https://sheeptester.github.io/scratch-gui/?url=https://boomerscratch.github.io/javascripts/PointerLockScratch3.js , the extension does not load! When i do it manually from the extension library, it gives me this error:
How do i fix this?
Uncaught (in promise) TypeError: Cannot read property 'yield' of null
at shared-dispatch.js:130
at new Promise (<anonymous>)
at WorkerDispatch._remoteTransferCall (shared-dispatch.js:126)
at WorkerDispatch.transferCall (shared-dispatch.js:82)
at WorkerDispatch.call (shared-dispatch.js:59)
at extension-worker.js:28
::::::::: :::::::: :::::::: :::: :::: :::::::::: ::::::::: ::::::: ::::::: ::: :+: :+: :+: :+: :+: :+: +:+:+: :+:+:+ :+: :+: :+: :+: :+: :+: :+: :+:+: +:+ +:+ +:+ +:+ +:+ +:+ +:+ +:+:+ +:+ +:+ +:+ +:+ +:+ :+:+ +:+ :+:+ +:+ +#++:++#+ +#+ +:+ +#+ +:+ +#+ +:+ +#+ +#++:++# +#++:++#: +#+ + +:+ +#+ + +:+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+# +#+ +#+# +#+ +#+ #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# ######### ######## ######## ### ### ########## ### ### ####### ####### #######

- Sheep_maker
-
1000+ posts
Creating extensions for Scratch 3.0
@Boomer001 My mod loads extensions from the URL as unofficial extensions, which are run in web workers, so they don't have access to `document`
- Sheep_maker This is a kumquat-free signature. :P
This is my signature. It appears below all my posts. Discuss it on my profile, not the forums. Here's how to make your own.
.postsignature { overflow: auto; } .scratchblocks { overflow-x: auto; overflow-y: hidden; }
- Boomer001
-
1000+ posts
Creating extensions for Scratch 3.0
removed
Last edited by Boomer001 (March 18, 2020 15:14:53)
::::::::: :::::::: :::::::: :::: :::: :::::::::: ::::::::: ::::::: ::::::: ::: :+: :+: :+: :+: :+: :+: +:+:+: :+:+:+ :+: :+: :+: :+: :+: :+: :+: :+:+: +:+ +:+ +:+ +:+ +:+ +:+ +:+ +:+:+ +:+ +:+ +:+ +:+ +:+ :+:+ +:+ :+:+ +:+ +#++:++#+ +#+ +:+ +#+ +:+ +#+ +:+ +#+ +#++:++# +#++:++#: +#+ + +:+ +#+ + +:+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+# +#+ +#+# +#+ +#+ #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# ######### ######## ######## ### ### ########## ### ### ####### ####### #######

- TurboCatboy
-
100+ posts
Creating extensions for Scratch 3.0
Hi NitroCipher,
I'm playing with scratch 3 and found this post. Can you tell me where I can find more information to make a Command button that in the arguments values come from a menu or array.
Samples:point towards [ v]
Thanks,
Allan Moreira
At the moment, not much is known (publically) about the extensions. I will post here as soon as I discover more.
sad::control cap
Last edited by TurboCatboy (March 18, 2020 12:38:10)
you can scroll
//this signature is yellow but whywhy is there no border for this one
//tell me! tell me!
//ah. another question.answer both NOW!answers:comments, out of scratchblocks, you can't, the comments are in scratchblocks
//third question: why are you not answering me :mad:
//fourth question: why do the emojis not work?
// fifth question: why did it take me this long to find the answers to the other questions
comment on my profile if that was funny
- Boomer001
-
1000+ posts
Creating extensions for Scratch 3.0
After a lot of struggling on how i needed to do everything, i finally managed to port my 3.0 extension to a copy of 3.0!
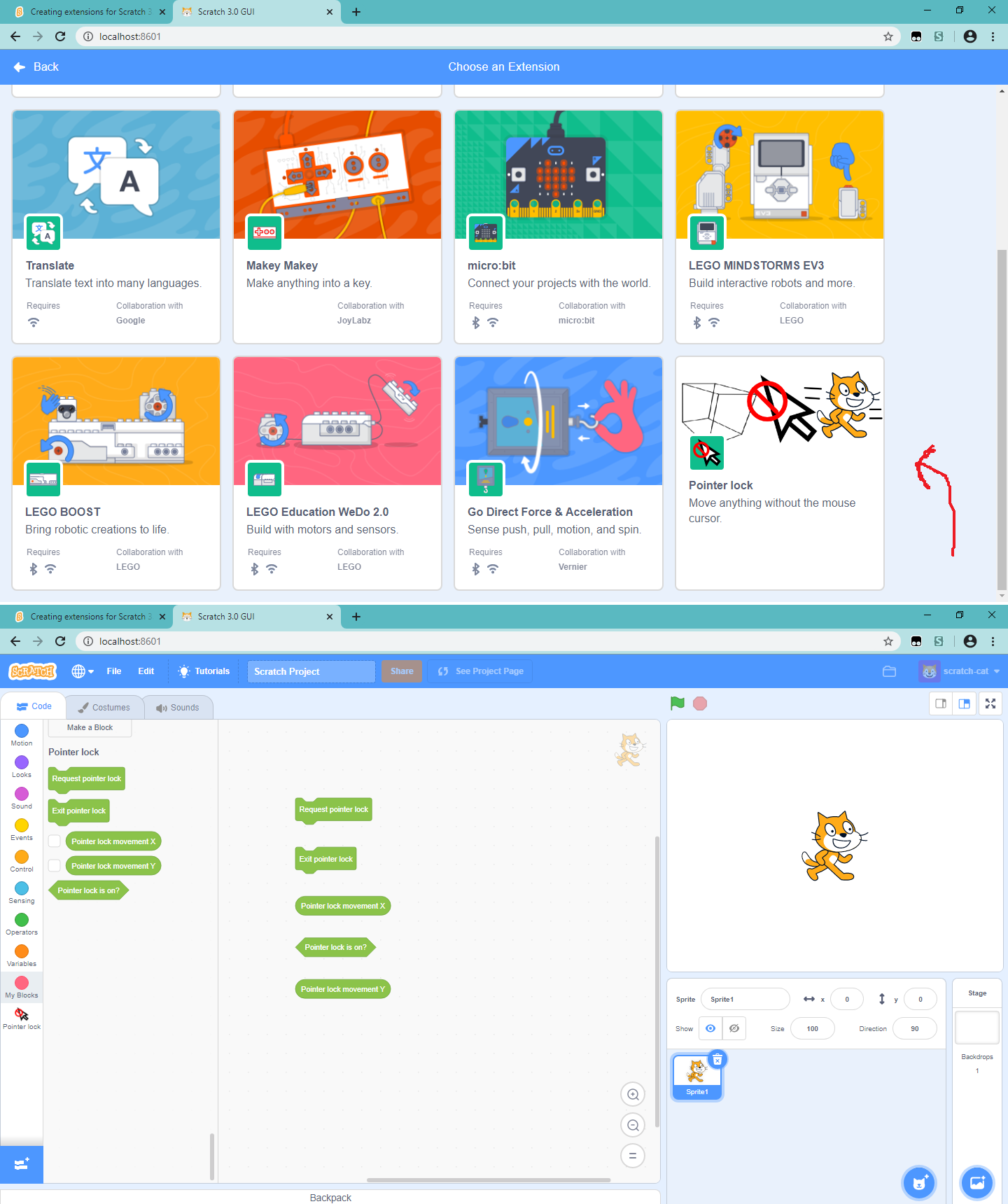
I readed this article with instructions on how to port a scratch 3 extension to a copy of scratch. My extension is pointer lock (for the people who don't know what that is: it disables your mouse cursor and tracks movement of it). You can find it in the extension library, and it has a icon, all just like a normal extension.
Edit: Published it to github pages.
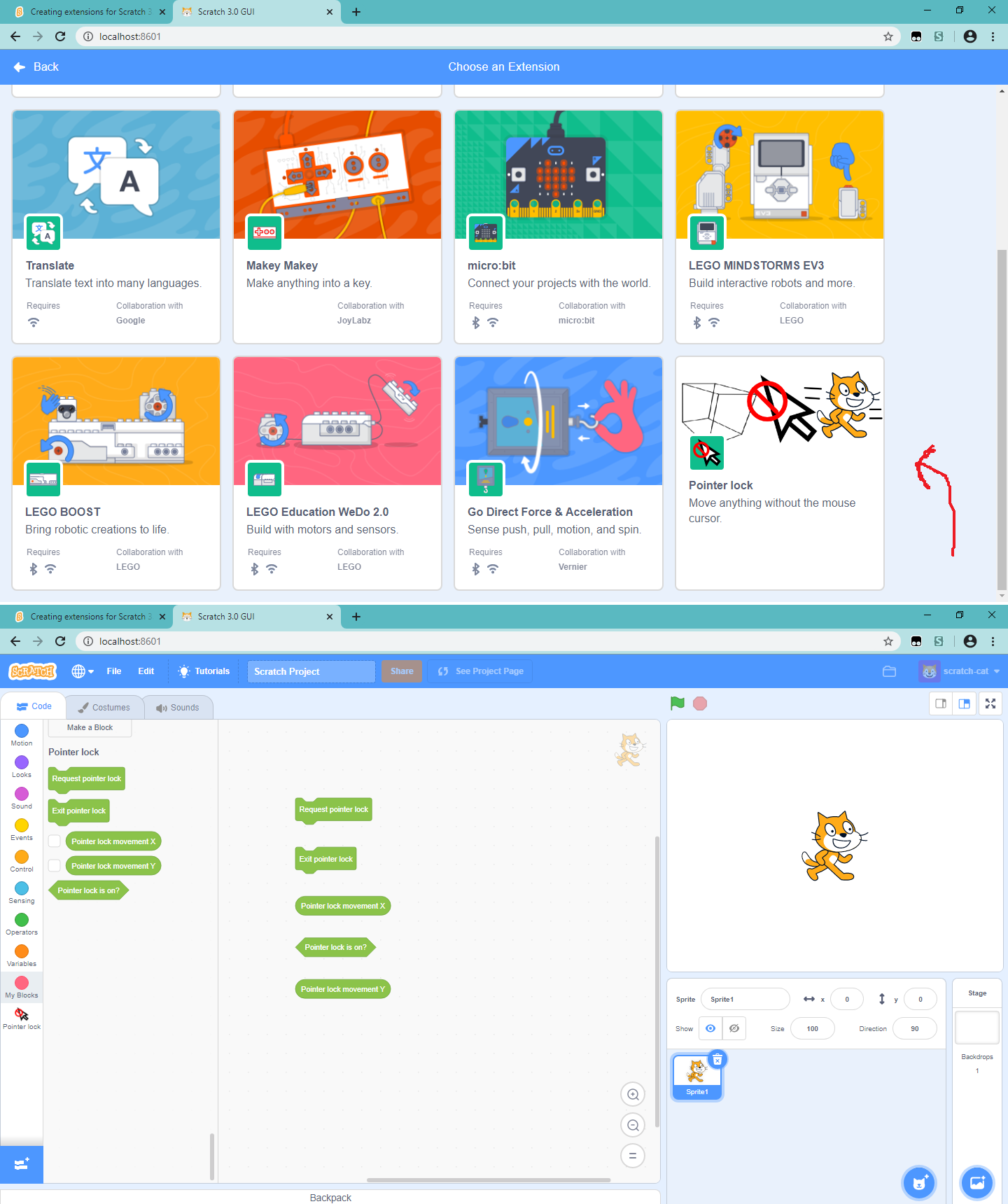
I readed this article with instructions on how to port a scratch 3 extension to a copy of scratch. My extension is pointer lock (for the people who don't know what that is: it disables your mouse cursor and tracks movement of it). You can find it in the extension library, and it has a icon, all just like a normal extension.
Edit: Published it to github pages.
Last edited by Boomer001 (March 21, 2020 15:17:22)
::::::::: :::::::: :::::::: :::: :::: :::::::::: ::::::::: ::::::: ::::::: ::: :+: :+: :+: :+: :+: :+: +:+:+: :+:+:+ :+: :+: :+: :+: :+: :+: :+: :+:+: +:+ +:+ +:+ +:+ +:+ +:+ +:+ +:+:+ +:+ +:+ +:+ +:+ +:+ :+:+ +:+ :+:+ +:+ +#++:++#+ +#+ +:+ +#+ +:+ +#+ +:+ +#+ +#++:++# +#++:++#: +#+ + +:+ +#+ + +:+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+# +#+ +#+# +#+ +#+ #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# ######### ######## ######## ### ### ########## ### ### ####### ####### #######

- joshua_tom
-
7 posts
Creating extensions for Scratch 3.0
See my extension for first an last words at https://scratch.mit.edu/projects/377678202/ do reply a nd remix

- 5e4
-
37 posts
Creating extensions for Scratch 3.0
i hope they except extensions sooner or later.
- Locness
-
100+ posts
Creating extensions for Scratch 3.0
After a lot of struggling on how i needed to do everything, i finally managed to port my 3.0 extension to a copy of 3.0!
Congrats! This extension seems amazing!
I don't do stuff on Scratch anymore, sorry

- Boomer001
-
1000+ posts
Creating extensions for Scratch 3.0
Thanks!After a lot of struggling on how i needed to do everything, i finally managed to port my 3.0 extension to a copy of 3.0!
Congrats! This extension seems amazing!

::::::::: :::::::: :::::::: :::: :::: :::::::::: ::::::::: ::::::: ::::::: ::: :+: :+: :+: :+: :+: :+: +:+:+: :+:+:+ :+: :+: :+: :+: :+: :+: :+: :+:+: +:+ +:+ +:+ +:+ +:+ +:+ +:+ +:+:+ +:+ +:+ +:+ +:+ +:+ :+:+ +:+ :+:+ +:+ +#++:++#+ +#+ +:+ +#+ +:+ +#+ +:+ +#+ +#++:++# +#++:++#: +#+ + +:+ +#+ + +:+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+ +#+# +#+ +#+# +#+ +#+ #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# #+# ######### ######## ######## ### ### ########## ### ### ####### ####### #######

- TurboCatboy
-
100+ posts
Creating extensions for Scratch 3.0
Creating extensions for Scratch 3.0There is now official documentation regarding extensions!, but feel free to use this post to get you started
You'll want to start off by creating your extension's class, and register the extension - In my case, this would be ‘NitroBlock’class NitroBlock { //In both instances, NitroBlock will be the name in both instances } Scratch.extensions.register(new NitroBlock());
Next, we will be constructing block and menu definitions - We will continue to use ‘NitroBlock’ through this tutorialgetInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [ ], "menus": { //we will get back to this in a later tutorial } }; }
We are going to take a look at how blocks are constructed
For those of you that are familiar with extensions for Scratch 2.0, we will start off with this: - If not, you can ignore this['r', 'letters %n through %n of %s', 'substringy', '2', '5', 'hello world'] //breakdown below: ['r' = block type, 'letters %n through %n of %s' = block text, 'substringy' = block ID/opcode]{ "opcode": "substringy", //This will be the ID code for the block "blockType": "reporter", //This can either be Boolean, reporter, command, or hat "text": "letters [num1] through [num2] of [string]", //This is the block text, and how it will display in the Scratch interface "arguments": { //Arguments are the input fields in the block. In the block text, place arguments in square brackets with the corresponding ID "num1": { //This is the ID for your argument "type": "number", //This can be either Boolean, number, or string "defaultValue": "2" //This is the default text that will appear in the input field, you can leave this blank if you wish }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } },
We will put this newly constructed code into the blocks object above - My code will now look like thisclass NitroBlock { getInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [{ "opcode": "substringy", "blockType": "reporter", "text": "letters [num1] through [num2] of [string]", "arguments": { "num1": { "type": "number", "defaultValue": "2" }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } }, }], "menus": { //we will get back to this in a later tutorial } }; } Scratch.extensions.register(new NitroBlock());
Next we come to the most important part, the code that actually runs the blocks! - I am keeping this short and simple for tutorial's sake.//Make sure you name this function with with the proper ID for the block you defined above substringy({num1, num2, string}) { //these names will match the argument names you used earlier, and will be used as the variables in your code //this code can be anything you want return string.substring(num1 - 1, num2); //for reporters and Boolean blocks the important thing is to use 'return' to get the value back into Scratch. }
Place this new code below your getInfo() functionclass NitroBlock { getInfo() { return { "id": "NitroBlock", "name": "NitroBlock", "blocks": [{ "opcode": "substringy", "blockType": "reporter", "text": "letters [num1] through [num2] of [string]", "arguments": { "num1": { "type": "number", "defaultValue": "2" }, "num2": { "type": "number", "defaultValue": "5" }, "string": { "type": "string", "defaultValue": "hello world" } } }, }], "menus": { //we will get back to this in a later tutorial } }; substringy({num1, num2, string}) { return string.substring(num1 - 1, num2); }; }
Save this code to your computer as a js file, in my case it is NitroBlock_3.js
Congratz, you now have your extension code created!!
You can use this tutorial to add it your own personal copy of scratch
Or, you can host the extension with gh-pages, and go here to test it (provide your own url, don't use mine)
Here is the archived copy of my original post
you can scroll
//this signature is yellow but whywhy is there no border for this one
//tell me! tell me!
//ah. another question.answer both NOW!answers:comments, out of scratchblocks, you can't, the comments are in scratchblocks
//third question: why are you not answering me :mad:
//fourth question: why do the emojis not work?
// fifth question: why did it take me this long to find the answers to the other questions
comment on my profile if that was funny