Discuss Scratch
- Discussion Forums
- » Developing Scratch Extensions
- » I made a gamepad extension (SB2/SBX only)
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
Garamol56's Gamepad Extension
About:
So, recently I was making a clone of Super Mario Bros for the NES, and I wanted to be able to control the game with my wired xbox 360 controller. I looked on this forum and found a gamepad extension, but that only gave 2 state values for L stick and R stick positions, and things were named confusingly. So I decided to create a new one.
Current Version: v1.4
Blocks:
Menus for dropdowns:
Buttons: Y, B, A, X, LB, RB, LT, RB, SELECT, START, LEFT STICK, RIGHT STICK
Left/Right: Left, Right
HVB: Horizontal, Vertical, Both
HV: Horizontal, Vertical
Direction: Up, Down, Left, Right, Up Left, Up Right, Down Left, Down Right
AEFE: Angle, Force
Planned Blocks:
Code:
Minified:
Commented:
Credit to Alyssa Rosenzweig for the force/angle function and deadzone
About:
So, recently I was making a clone of Super Mario Bros for the NES, and I wanted to be able to control the game with my wired xbox 360 controller. I looked on this forum and found a gamepad extension, but that only gave 2 state values for L stick and R stick positions, and things were named confusingly. So I decided to create a new one.
Current Version: v1.4
Blocks:
<[(Buttons) v] is pressed?::extension> // Returns true if the selected button is pressed.
When [(Buttons) v] is pressed::hat extension // Run code when a button is pressed.
([(Left/Right) v] stick [(HV) v] position::extension) // Return the amount that the specified stick is pushed in a direction (-100% = -1, 100% = 1)
([(Left/Right) v] stick [(HVB) v] direction::extension) // Return a plaintext value containing the direction (Up/Down/Left/Right/Up Right etc..)
<[(Left/Right) v] stick is facing [(Direction) v]::extension> // Return true if the specified stick is facing a certain direction
When [(Left/Right) v] stick is facing [(Direction) v]::hat extension // Run code when a specified stick is facing a certain direction
([(Left/Right) v] stick [(AEFE) v]::extension) // Return the angle or force of a specified stick
Menus for dropdowns:
Buttons: Y, B, A, X, LB, RB, LT, RB, SELECT, START, LEFT STICK, RIGHT STICK
Left/Right: Left, Right
HVB: Horizontal, Vertical, Both
HV: Horizontal, Vertical
Direction: Up, Down, Left, Right, Up Left, Up Right, Down Left, Down Right
AEFE: Angle, Force
Planned Blocks:
([(Button) v] press time::extension) // Return the amount of time a specified button has been pressed for
Code:
Minified:
!function(e){e._shutdown=function(){};const t="ongamepadconnected"in window,s={};function a(e){s[e.index]=e}window.addEventListener("gamepadconnected",function(e){a(e.gamepad)}),window.addEventListener("gamepaddisconnected",function(e){!function(e){delete s[e.index]}(e.gamepad)}),t||setInterval(function(){for(var e=navigator.getGamepads?navigator.getGamepads():navigator.webkitGetGamepads?navigator.webkitGetGamepads():[],t=0;t<e.length;t++)e[t]&&(e[t].index in s?s[e[t].index]=e[t]:a(e[t]))},1),e._getStatus=(()=>({status:2,msg:"Ready"})),e.aefe=function(e,t){x=s[0].axes["Left"===e?0:2],y=-s[0].axes["Left"===e?1:5];const a=8e3/32767;return-a<x&&x<a&&(x=0),-a<y&&y<a&&(y=0),"Angle"===t?value=180*Math.atan2(x,y)/Math.PI:Math.sqrt(x*x+y*y)},e.ispressed=(e=>s[0].buttons[["A","B","X","Y","LB","RB","LT","RT","SELECT","START","LEFT STICK","RIGHT STICK"].indexOf(e)].pressed),e.stickpos=((e,t)=>s[0].axes[["LeftHorizontal","LeftVertical","RightHorizontal","","","RightVertical"].indexOf(e+t)]),e.stickfacing=function(e,t){let a="";const n="Vertical"===t||"Both"===t,i="Horizontal"===t||"Both"===t;return"Left"===e?(n&&(s[0].axes[1]<-.5?a+="Up ":s[0].axes[1]>.5&&(a+="Down ")),i&&(s[0].axes[0]<-.5?a+="Left":s[0].axes[0]>.5&&(a+="Right"))):(n&&(s[0].axes[5]<-.5?a+="Up ":s[0].axes[5]>.5&&(a+="Down ")),i&&(s[0].axes[2]<-.5?a+="Left":s[0].axes[2]>.5&&(a+="Right"))),a},e.stickis=function(e,t){if("Left"===e)switch(t){case"Up":return s[0].axes[1]<-.5;case"Down":return s[0].axes[1]>.5;case"Left":return s[0].axes[0]<-.5;case"Right":return s[0].axes[0]>.5;case"Up Left":return s[0].axes[1]<-.5&&s[0].axes[0]<-.5;case"Up Right":return s[0].axes[1]<-.5&&s[0].axes[0]>.5;case"Down Left":return s[0].axes[1]>.5&&s[0].axes[0]<-.5;case"Down Right":return s[0].axes[1]>.5&&s[0].axes[0]>.5}else switch(t){case"Up":return s[0].axes[5]<-.5;case"Down":return s[0].axes[5]>.5;case"Left":return s[0].axes[2]<-.5;case"Right":return s[0].axes[2]>.5;case"Up Left":return s[0].axes[5]<-.5&&s[0].axes[2]<-.5;case"Up Right":return s[0].axes[5]<-.5&&s[0].axes[2]>.5;case"Down Left":return s[0].axes[5]>.5&&s[0].axes[2]<-.5;case"Down Right":return s[0].axes[5]>.5&&s[0].axes[2]>.5}},ScratchExtensions.register("Gamepad",{blocks:[["b","%m.buttons is pressed?","ispressed","A"],["h","When %m.buttons is pressed","ispressed","A"],["r","%m.lr stick %m.hv position","stickpos","Left","Horizontal"],["r","%m.lr stick %m.hvb direction","stickfacing","Left","Both"],["b","%m.lr stick is facing %m.dir?","stickis","Left","Up"],["h","When %m.lr stick is facing %m.dir","stickis","Left","Up"],["r","%m.lr stick %m.aefe","aefe","Left","Angle"]],menus:{buttons:["Y","B","A","X","LB","RB","LT","RT","SELECT","START","LEFT STICK","RIGHT STICK"],lr:["Left","Right"],hv:["Horizontal","Vertical"],hvb:["Horizontal","Vertical","Both"],dir:["Up","Down","Left","Right","Up Left","Up Right","Down Left","Down Right"],aefe:["Angle","Force"]}},e)}({});
Commented:
(function(ext) { ext._shutdown = function() {}; const haveEvents = "ongamepadconnected" in window, controllers = {}; function connecthandler(e) { addgamepad(e.gamepad); // Add the controller to the controllers object } function addgamepad(e) { controllers[e.index] = e; // Add the controller to the controllers array } function disconnecthandler(e) { removegamepad(e.gamepad); // Handle Disconnects } function removegamepad(e) { delete controllers[e.index]; // Remove controllers when they are disconnected } function scangamepads() { for (var e = navigator.getGamepads ? navigator.getGamepads() : navigator.webkitGetGamepads ? navigator.webkitGetGamepads() : [], n = 0; n < e.length; n++) e[n] && (e[n].index in controllers ? (controllers[e[n].index] = e[n]) : addgamepad(e[n])); } window.addEventListener("gamepadconnected", connecthandler); window.addEventListener("gamepaddisconnected", disconnecthandler); haveEvents || setInterval(scangamepads, 1); // When the controller is detected, enable the extension ext._getStatus = () => ({ status: 2, msg: "Ready" }); ext.aefe = function(stick, af) { // Return the force or angle of a specified stick x = controllers[0].axes[stick === "Left" ? 0 : 2]; y = -controllers[0].axes[stick === "Left" ? 1 : 5]; const ni = 8000 / 32767; // Deadzone if (-ni < x && x < ni) x = 0; if (-ni < y && y < ni) y = 0; return af === "Angle" ? (value = 180 * Math.atan2(x, y) / Math.PI) : Math.sqrt(x * x + y * y); }; // Return if the user is pressing the button given to the function ext.ispressed = button => controllers[0].buttons[["A", "B", "X", "Y", "LB", "RB", "LT", "RT", "SELECT", "START", "LEFT STICK", "RIGHT STICK"].indexOf(button)].pressed; // Return the value of the axes for the stick and the direction specified ext.stickpos = (stick, axis) => controllers[0].axes[["LeftHorizontal", "LeftVertical", "RightHorizontal", "", "", "RightVertical"].indexOf(stick + axis)]; ext.stickfacing = function(stick, axis) { // Return a string representation of the direction of the given control stick let output = ""; const veritcal = axis === "Vertical" || axis === "Both", horizontal = axis === "Horizontal" || axis === "Both"; if (stick === "Left") { if (veritcal) { if (controllers[0].axes[1] < -0.5) output += "Up "; else if (controllers[0].axes[1] > 0.5) output += "Down "; } if (horizontal) { if (controllers[0].axes[0] < -0.5) output += "Left"; else if (controllers[0].axes[0] > 0.5) output += "Right"; } } else { if (veritcal) { if (controllers[0].axes[5] < -0.5) output += "Up "; else if (controllers[0].axes[5] > 0.5) output += "Down "; } if (horizontal) { if (controllers[0].axes[2] < -0.5) output += "Left"; else if (controllers[0].axes[2] > 0.5) output += "Right"; } } return output; }; ext.stickis = function(stick, direction) { // Return true or false depending on if the specified stick is facing a direction if (stick === "Left") { switch (direction) { case "Up": return controllers[0].axes[1] < -0.5; case "Down": return controllers[0].axes[1] > 0.5; case "Left": return controllers[0].axes[0] < -0.5; case "Right": return controllers[0].axes[0] > 0.5; case "Up Left": return controllers[0].axes[1] < -0.5 && controllers[0].axes[0] < -0.5; case "Up Right": return controllers[0].axes[1] < -0.5 && controllers[0].axes[0] > 0.5; case "Down Left": return controllers[0].axes[1] > 0.5 && controllers[0].axes[0] < -0.5; case "Down Right": return controllers[0].axes[1] > 0.5 && controllers[0].axes[0] > 0.5; } } else { switch (direction) { case "Up": return controllers[0].axes[5] < -0.5; case "Down": return controllers[0].axes[5] > 0.5; case "Left": return controllers[0].axes[2] < -0.5; case "Right": return controllers[0].axes[2] > 0.5; case "Up Left": return controllers[0].axes[5] < -0.5 && controllers[0].axes[2] < -0.5; case "Up Right": return controllers[0].axes[5] < -0.5 && controllers[0].axes[2] > 0.5; case "Down Left": return controllers[0].axes[5] > 0.5 && controllers[0].axes[2] < -0.5; case "Down Right": return controllers[0].axes[5] > 0.5 && controllers[0].axes[2] > 0.5; } } }; ScratchExtensions.register("Gamepad", { blocks: [ ["b", "%m.buttons is pressed?", "ispressed", "A"], ["h", "When %m.buttons is pressed", "ispressed", "A"], ["r", "%m.lr stick %m.hv position", "stickpos", "Left", "Horizontal"], ["r", "%m.lr stick %m.hvb direction", "stickfacing", "Left", "Both"], ["b", "%m.lr stick is facing %m.dir?", "stickis", "Left", "Up"], ["h", "When %m.lr stick is facing %m.dir", "stickis", "Left", "Up"], ["r", "%m.lr stick %m.aefe", "aefe", "Left", "Angle"] ], menus: { buttons: ["Y", "B", "A", "X", "LB", "RB", "LT", "RT", "SELECT", "START", "LEFT STICK", "RIGHT STICK"], lr: ["Left", "Right"], hv: ["Horizontal", "Vertical"], hvb: ["Horizontal", "Vertical", "Both"], dir: ["Up", "Down", "Left", "Right", "Up Left", "Up Right", "Down Left", "Down Right"], aefe: ["Angle", "Force"] } }, ext); // Register the extension so scratch can use it })({});
Credit to Alyssa Rosenzweig for the force/angle function and deadzone
Last edited by Garamol56 (May 4, 2021 17:16:35)
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
bump
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
Does anyone have any suggestions for version 1.4?
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- cat1554
-
18 posts
I made a gamepad extension (SB2/SBX only)
I honestley expected Wii U Gamepad for this, so maybe also implement that? Does anyone have any suggestions for version 1.4?
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
I don't actually own a WIi U, so it'd be quite hard to make.I honestley expected Wii U Gamepad for this, so maybe also implement that? Does anyone have any suggestions for version 1.4?
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- dragon0307
-
79 posts
I made a gamepad extension (SB2/SBX only)
Try WiinUPro. It is Windows exclusive, but you can use it to map Wii U Pro Controller buttons to Xbox, keyboard, mouse and joystick inputs. However, if you want to use Xbox controllers with it, you need to download the SCP Driver, as otherwise you can't trick Windows into thinking your Wii U controller is an Xbox controller. There is also a lighter version of it which comes with the SCP driver but only supports mapping to Xbox controls called WiinUSoft.I don't actually own a WIi U, so it'd be quite hard to make.I honestley expected Wii U Gamepad for this, so maybe also implement that? Does anyone have any suggestions for version 1.4?
The more you nag for it, the less likely it is to happen.
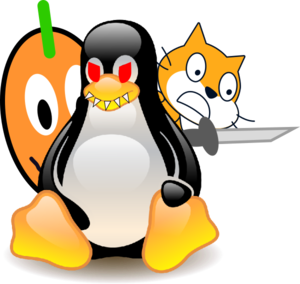
- coolyoshi7
-
24 posts
I made a gamepad extension (SB2/SBX only)
I Tried Wiin U Pro It Wouldnt Work For MeTry WiinUPro. It is Windows exclusive, but you can use it to map Wii U Pro Controller buttons to Xbox, keyboard, mouse and joystick inputs. However, if you want to use Xbox controllers with it, you need to download the SCP Driver, as otherwise you can't trick Windows into thinking your Wii U controller is an Xbox controller. There is also a lighter version of it which comes with the SCP driver but only supports mapping to Xbox controls called WiinUSoft.I don't actually own a WIi U, so it'd be quite hard to make.I honestley expected Wii U Gamepad for this, so maybe also implement that? Does anyone have any suggestions for version 1.4?
- coolyoshi7
-
24 posts
I made a gamepad extension (SB2/SBX only)
Have You Tryed It Before If You Put It In A Game So I Can Test ItTry WiinUPro. It is Windows exclusive, but you can use it to map Wii U Pro Controller buttons to Xbox, keyboard, mouse and joystick inputs. However, if you want to use Xbox controllers with it, you need to download the SCP Driver, as otherwise you can't trick Windows into thinking your Wii U controller is an Xbox controller. There is also a lighter version of it which comes with the SCP driver but only supports mapping to Xbox controls called WiinUSoft.I don't actually own a WIi U, so it'd be quite hard to make.I honestley expected Wii U Gamepad for this, so maybe also implement that? Does anyone have any suggestions for version 1.4?
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
I'm not going to be adding support for Wii U. If you need it, contact someone else
Last edited by Garamol56 (April 17, 2019 16:54:59)
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
bump
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
bump
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- lktornado360
-
36 posts
I made a gamepad extension (SB2/SBX only)
so…how to use code?
“Heluim is inert, it does not react to anything. This makes it perfect to help create a safe atmosphere for welding.” & “Atomic Number: 2; Formula: He; Atomic Weight: 4.002602; State at 20° C: gas; Boiling point: -268.928° C; Melting point: unknown; % in the Universe: 23; % in the Earth's crust: 0.00000055; % in Earths's oceans: 0.00000000072; % in humans: none.”
–The Periodic Table by Sean Callery and Miranda Smith
- Burgame-Productions
-
59 posts
I made a gamepad extension (SB2/SBX only)
Garamol56's Gamepad Extension
About:
So, recently I was making a clone of Super Mario Bros for the NES, and I wanted to be able to control the game with my wired xbox 360 controller. I looked on this forum and found a gamepad extension, but that only gave 2 state values for L stick and R stick positions, and things were named confusingly. So I decided to create a new one.
Current Version: v1.3
Blocks:<[(Buttons) v] is pressed?::extension> // Returns true if the selected button is pressed.
When [(Buttons) v] is pressed::hat extension // Run code when a button is pressed.
([(Left/Right) v] stick [(HV) v] position::extension) // Return the amount that the specified stick is pushed in a direction (-100% = -1, 100% = 1)
([(Left/Right) v] stick [(HVB) v] direction::extension) // Return a plaintext value containing the direction (Up/Down/Left/Right/Up Right etc..)
<[(Left/Right) v] stick is facing [(Direction) v]::extension> // Return true if the specified stick is facing a certain direction
When [(Left/Right) v] stick is facing [(Direction) v]::hat extension // Run code when a specified stick is facing a certain direction
([(Left/Right) v] stick [(AEFE) v]::extension) // Return the angle or force of a specified stick
Menus for dropdowns:
Buttons: Y, B, A, X, LB, RB, LT, RB, SELECT, START, LEFT STICK, RIGHT STICK
Left/Right: Left, Right
HVB: Horizontal, Vertical, Both
HV: Horizontal, Vertical
Direction: Up, Down, Left, Right, Up Left, Up Right, Down Left, Down Right
AEFE: Angle, Force
Planned Blocks:([(Button) v] press time::extension) // Return the amount of time a specified button has been pressed for
Code:
Minified:!function(e){e._shutdown=function(){};var t="ongamepadconnected"in window,i={};function n(e){i[e.index]=e}function a(){for(var e=navigator.getGamepads?navigator.getGamepads():navigator.webkitGetGamepads?navigator.webkitGetGamepads():[],t=0;t<e.length;t++)e[t]&&(e[t].index in i?i[e[t].index]=e[t]:n(e[t]))}window.addEventListener("gamepadconnected",function(e){n(e.gamepad)}),window.addEventListener("gamepaddisconnected",function(e){!function(e){delete i[e.index]}(e.gamepad)}),t||setInterval(a,1),e._getStatus=function(){return{status:2,msg:"Ready"}};var s=8e3/32767;e.aefe=function(e,t){switch(e){case"Left":x=i[0].axes[0],y=-i[0].axes[1];break;case"Right":x=i[0].axes[2],y=-i[0].axes[5]}switch(-s<x&&x<s&&(x=0),-s<y&&y<s&&(y=0),t){case"Angle":return value=180*Math.atan2(x,y)/Math.PI;case"Force":return Math.sqrt(x*x+y*y)}},e.ispressed=function(e){return i[0].buttons[["Y","B","A","X","LB","RB","LT","RT","SELECT","START","LEFT STICK","RIGHT STICK"].indexOf(e)].pressed},e.stickpos=function(e,t){return i[0].axes[["LeftHorizontal","LeftVertical","RightHorizontal","","","RightVertical"].indexOf(e+t)]},e.stickfacing=function(e,t){let n="";return"Left"==e&&("Both"!=t&&"Vertical"!=t||(i[0].axes[1]<-.5?n+="Up ":i[0].axes[1]>.5&&(n+="Down ")),"Both"!=t&&"Horizontal"!=t||(i[0].axes[0]<-.5?n+="Left":i[0].axes[0]>.5&&(n+="Right"))),"Right"==e&&("Both"!=t&&"Vertical"!=t||(i[0].axes[5]<-.5?n+="Up ":i[0].axes[5]>.5&&(n+="Down ")),"Both"!=t&&"Horizontal"!=t||(i[0].axes[2]<-.5?n+="Left":i[0].axes[2]>.5&&(n+="Right"))),n},e.stickis=function(e,t){if("Left"==e){if("Up"==t)return i[0].axes[1]<-.5;if("Down"==t)return i[0].axes[1]>.5;if("Left"==t)return i[0].axes[0]<-.5;if("Right"==t)return i[0].axes[0]>.5;if("Up Left"==t)return i[0].axes[1]<-.5&&i[0].axes[0]<-.5;if("Up Right"==t)return i[0].axes[1]<-.5&&i[0].axes[0]>.5;if("Down Left"==t)return i[0].axes[1]>.5&&i[0].axes[0]<-.5;if("Down Right"==t)return i[0].axes[1]>.5&&i[0].axes[0]>.5}if("Left"==e){if("Up"==t)return i[0].axes[5]<-.5;if("Down"==t)return i[0].axes[5]>.5;if("Left"==t)return i[0].axes[2]<-.5;if("Right"==t)return i[0].axes[2]>.5;if("Up Left"==t)return i[0].axes[5]<-.5&&i[0].axes[2]<-.5;if("Up Right"==t)return i[0].axes[5]<-.5&&i[0].axes[2]>.5;if("Down Left"==t)return i[0].axes[5]>.5&&i[0].axes[2]<-.5;if("Down Right"==t)return i[0].axes[5]>.5&&i[0].axes[2]>.5}};ScratchExtensions.register("Gamepad",{blocks:[["b","%m.buttons is pressed?","ispressed","A"],["h","When %m.buttons is pressed","ispressed","A"],["r","%m.lr stick %m.hv position","stickpos","Left","Horizontal"],["r","%m.lr stick %m.hvb direction","stickfacing","Left","Both"],["b","%m.lr stick is facing %m.dir?","stickis","Left","Up"],["h","When %m.lr stick is facing %m.dir","stickis","Left","Up"],["r","%m.lr stick %m.aefe","aefe","Left","Angle"]],menus:{buttons:["Y","B","A","X","LB","RB","LT","RT","SELECT","START","LEFT STICK","RIGHT STICK"],lr:["Left","Right"],hv:["Horizontal","Vertical"],hvb:["Horizontal","Vertical","Both"],dir:["Up","Down","Left","Right","Up Left","Up Right","Down Left","Down Right"],aefe:["Angle","Force"]}},e)}({});
Commented:(function(ext) {
ext._shutdown = function() {};
var haveEvents = "ongamepadconnected" in window,
controllers = {};
function connecthandler(e) {
addgamepad(e.gamepad) // Add the controller to the "controllers" object
}
function addgamepad(e) {
controllers[e.index] = e // Add the controller to the "controllers" object
}
function disconnecthandler(e) {
removegamepad(e.gamepad) // Handle Disconnects
}
function removegamepad(e) {
delete controllers[e.index] // Remove controllers when disconnected
}
function updateStatus() {
haveEvents || scangamepads(), requestAnimationFrame(updateStatus) // Update the controller values
}
function scangamepads() {
for (var e = navigator.getGamepads ? navigator.getGamepads() : navigator.webkitGetGamepads ? navigator.webkitGetGamepads() : [], n = 0; n < e.length; n++) e[n] && (e[n].index in controllers ? controllers[e[n].index] = e[n] : addgamepad(e[n]))
}
window.addEventListener("gamepadconnected", connecthandler), window.addEventListener("gamepaddisconnected", disconnecthandler), haveEvents || setInterval(scangamepads, 1); // When the controller is detected, enable the extension
ext._getStatus = function() {
return {
status: 2,
msg: 'Ready'
};
};
var ni = 8000 / 32767; // Deadzone
ext.aefe = function(s,af) { // Return the force or angle of a specified stick
var xp, yp;
switch (s) {
case "Left":
x = controllers[0].axes[0];
y = -controllers[0].axes[1];
break;
case "Right":
x = controllers[0].axes[2];
y = -controllers[0].axes[5];
break;
}
if (-ni < x && x < ni) x = 0;
if (-ni < y && y < ni) y = 0;
switch(af) {
case "Angle":
return(value = 180 * Math.atan2(x, y) / Math.PI);
break;
case "Force":
return Math.sqrt(x*x + y*y);
break;
}
};
ext.ispressed = function(b) {
return (controllers[0].buttons[["Y", "B", "A", "X", "LB", "RB", "LT", "RT", "SELECT", "START", "LEFT STICK", "RIGHT STICK"].indexOf(b)].pressed); // Return if the user is pressing the button given to the function
};
ext.stickpos = function(s, hv) {
return (controllers[0].axes[(["LeftHorizontal", "LeftVertical", "RightHorizontal", "", "", "RightVertical"].indexOf(s + hv))]); // Return the value of the axes for the stick and the direction specified
};
ext.stickfacing = function(s, hvb) { // Return a plaintext direction for a control stick
let output = "";
if (s == "Left") {
if (hvb == "Both" || hvb == "Vertical") {
if (controllers[0].axes[1] < -.5) {
output += "Up "
} else if (controllers[0].axes[1] > .5) {
output += "Down "
}
}
if (hvb == "Both" || hvb == "Horizontal") {
if (controllers[0].axes[0] < -.5) {
output += "Left"
} else if (controllers[0].axes[0] > .5) {
output += "Right"
}
}
};
if (s == "Right") {
if (hvb == "Both" || hvb == "Vertical") {
if (controllers[0].axes[5] < -.5) {
output += "Up "
} else if (controllers[0].axes[5] > .5) {
output += "Down "
}
}
if (hvb == "Both" || hvb == "Horizontal") {
if (controllers[0].axes[2] < -.5) {
output += "Left"
} else if (controllers[0].axes[2] > .5) {
output += "Right"
}
}
};
return (output);
};
ext.stickis = function(s, dir) { // Return true or false depending on if the specified stick is facing a direction
if (s == "Left") {
if (dir == "Up") {
return (controllers[0].axes[1] < -.5)
}
if (dir == "Down") {
return (controllers[0].axes[1] > .5)
}
if (dir == "Left") {
return (controllers[0].axes[0] < -.5)
}
if (dir == "Right") {
return (controllers[0].axes[0] > .5)
}
if (dir == "Up Left") {
return (controllers[0].axes[1] < -.5 && (controllers[0].axes[0] < -.5))
}
if (dir == "Up Right") {
return (controllers[0].axes[1] < -.5 && (controllers[0].axes[0] > .5))
}
if (dir == "Down Left") {
return (controllers[0].axes[1] > .5 && (controllers[0].axes[0] < -.5))
}
if (dir == "Down Right") {
return (controllers[0].axes[1] > .5 && (controllers[0].axes[0] > .5))
}
}
if (s == "Left") {
if (dir == "Up") {
return (controllers[0].axes[5] < -.5)
}
if (dir == "Down") {
return (controllers[0].axes[5] > .5)
}
if (dir == "Left") {
return (controllers[0].axes[2] < -.5)
}
if (dir == "Right") {
return (controllers[0].axes[2] > .5)
}
if (dir == "Up Left") {
return (controllers[0].axes[5] < -.5 && (controllers[0].axes[2] < -.5))
}
if (dir == "Up Right") {
return (controllers[0].axes[5] < -.5 && (controllers[0].axes[2] > .5))
}
if (dir == "Down Left") {
return (controllers[0].axes[5] > .5 && (controllers[0].axes[2] < -.5))
}
if (dir == "Down Right") {
return (controllers[0].axes[5] > .5 && (controllers[0].axes[2] > .5))
}
}
};
var descriptor = {
blocks: [
['b', '%m.buttons is pressed?', 'ispressed', "A"],
['h', 'When %m.buttons is pressed', 'ispressed', "A"],
['r', '%m.lr stick %m.hv position', 'stickpos', "Left", "Horizontal"],
['r', '%m.lr stick %m.hvb direction', 'stickfacing', "Left", "Both"],
['b', '%m.lr stick is facing %m.dir?', 'stickis', "Left", "Up"],
['h', 'When %m.lr stick is facing %m.dir', 'stickis', "Left", "Up"],
['r', '%m.lr stick %m.aefe', 'aefe', 'Left', "Angle"],
],
menus: {
buttons: ["Y", "B", "A", "X", "LB", "RB", "LT", "RT", "SELECT", "START", "LEFT STICK", "RIGHT STICK"],
lr: ["Left", "Right"],
hv: ["Horizontal", "Vertical"],
hvb: ["Horizontal", "Vertical", "Both"],
dir: ["Up", "Down", "Left", "Right", "Up Left", "Up Right", "Down Left", "Down Right"],
aefe: ["Angle", "Force"]
}
};
ScratchExtensions.register('Gamepad', descriptor, ext); // Register the extension so scratch can use it
})({});
Credit to Alyssa Rosenzweig for the force/angle function and deadzone
That would be awesome! Sadly I don’t know what ST will think of it
Swiftz, your one stop shop for all your art, programming, advertising and more needs!
https://scratch.mit.edu/studios/8063592/]
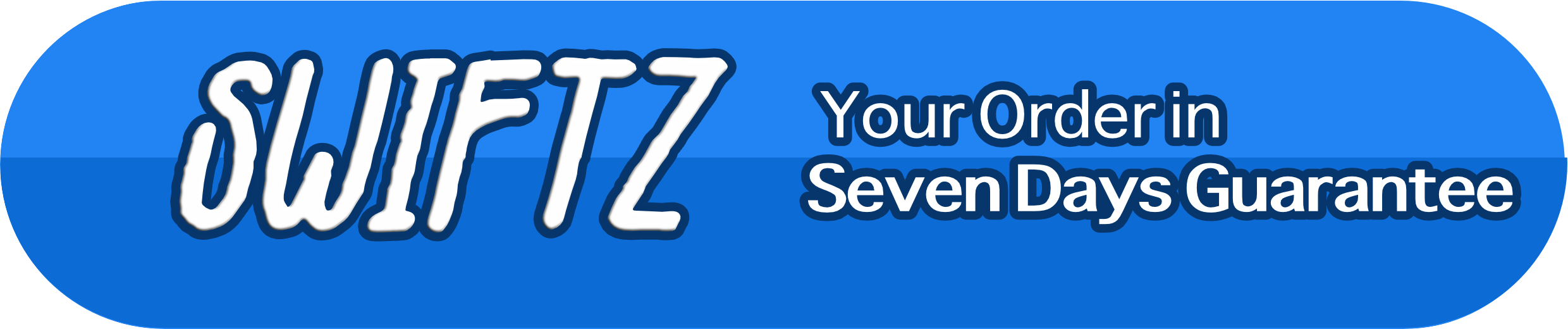
- Garamol56
-
100+ posts
I made a gamepad extension (SB2/SBX only)
There's essentially no reason to use this code, as you can't share projects using it and it only works on scratchX so…how to use code?
Current Posts: 266
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
Code to find my posts: Z2FyYW1vbDU2
Logic will get you from A to B. Imagination will take you everywhere - Albert Einstein
- lktornado360
-
36 posts
I made a gamepad extension (SB2/SBX only)
Well how do you use it on ScratchX?
“Heluim is inert, it does not react to anything. This makes it perfect to help create a safe atmosphere for welding.” & “Atomic Number: 2; Formula: He; Atomic Weight: 4.002602; State at 20° C: gas; Boiling point: -268.928° C; Melting point: unknown; % in the Universe: 23; % in the Earth's crust: 0.00000055; % in Earths's oceans: 0.00000000072; % in humans: none.”
–The Periodic Table by Sean Callery and Miranda Smith
- superman32123
-
30 posts
I made a gamepad extension (SB2/SBX only)
i want to use it (does it work with xbox 1 controller)
- lktornado360
-
36 posts
I made a gamepad extension (SB2/SBX only)
If it has USB, yes it works. Just press those three dots at the top-right of the screen in ScratchX, press More Tools, press Developer Tools, press Console, and paste in i want to use it (does it work with xbox 1 controller)
!function(e){e._shutdown=function(){};var t=“ongamepadconnected”in window,i={};function n(e){i=e}function a(){for(var e=navigator.getGamepads?navigator.getGamepads():navigator.webkitGetGamepads?navigator.webkitGetGamepads():,t=0;t<e.length;t++)e&&(e.index in i?i[e.index]=e:n(e))}window.addEventListener(“gamepadconnected”,function(e){n(e.gamepad)}),window.addEventListener(“gamepaddisconnected”,function(e){!function(e){delete i}(e.gamepad)}),t||setInterval(a,1),e._getStatus=function(){return{status:2,msg:“Ready”}};var s=8e3/32767;e.aefe=function(e,t){switch(e){case“Left”:x=i.axes,y=-i.axes;break;case“Right”:x=i.axes,y=-i.axes}switch(-s<x&&x<s&&(x=0),-s<y&&y<s&&(y=0),t){case“Angle”:return value=180*Math.atan2(x,y)/Math.PI;case“Force”:return Math.sqrt(x*x+y*y)}},e.ispressed=function(e){return i.buttons[.indexOf(e)].pressed},e.stickpos=function(e,t){return i.axes[.indexOf(e+t)]},e.stickfacing=function(e,t){let n=“”;return“Left”==e&&(“Both”!=t&&“Vertical”!=t||(i.axes<-.5?n+=“Up ”:i.axes>.5&&(n+=“Down ”)),“Both”!=t&&“Horizontal”!=t||(i.axes<-.5?n+=“Left”:i.axes>.5&&(n+=“Right”))),“Right”==e&&(“Both”!=t&&“Vertical”!=t||(i.axes<-.5?n+=“Up ”:i.axes>.5&&(n+=“Down ”)),“Both”!=t&&“Horizontal”!=t||(i.axes<-.5?n+=“Left”:i.axes>.5&&(n+=“Right”))),n},e.stickis=function(e,t){if(“Left”==e){if(“Up”==t)return i.axes<-.5;if(“Down”==t)return i.axes>.5;if(“Left”==t)return i.axes<-.5;if(“Right”==t)return i.axes>.5;if(“Up Left”==t)return i.axes<-.5&&i.axes<-.5;if(“Up Right”==t)return i.axes<-.5&&i.axes>.5;if(“Down Left”==t)return i.axes>.5&&i.axes<-.5;if(“Down Right”==t)return i.axes>.5&&i.axes>.5}if(“Left”==e){if(“Up”==t)return i.axes<-.5;if(“Down”==t)return i.axes>.5;if(“Left”==t)return i.axes<-.5;if(“Right”==t)return i.axes>.5;if(“Up Left”==t)return i.axes<-.5&&i.axes<-.5;if(“Up Right”==t)return i.axes<-.5&&i.axes>.5;if(“Down Left”==t)return i.axes>.5&&i.axes<-.5;if(“Down Right”==t)return i.axes>.5&&i.axes>.5}};ScratchExtensions.register(“Gamepad”,{blocks:[,,,,,,],menus:{buttons:,lr:,hv:,hvb:,dir:,aefe:}},e)}({});
“Heluim is inert, it does not react to anything. This makes it perfect to help create a safe atmosphere for welding.” & “Atomic Number: 2; Formula: He; Atomic Weight: 4.002602; State at 20° C: gas; Boiling point: -268.928° C; Melting point: unknown; % in the Universe: 23; % in the Earth's crust: 0.00000055; % in Earths's oceans: 0.00000000072; % in humans: none.”
–The Periodic Table by Sean Callery and Miranda Smith
- lktornado360
-
36 posts
I made a gamepad extension (SB2/SBX only)
and press enter
“Heluim is inert, it does not react to anything. This makes it perfect to help create a safe atmosphere for welding.” & “Atomic Number: 2; Formula: He; Atomic Weight: 4.002602; State at 20° C: gas; Boiling point: -268.928° C; Melting point: unknown; % in the Universe: 23; % in the Earth's crust: 0.00000055; % in Earths's oceans: 0.00000000072; % in humans: none.”
–The Periodic Table by Sean Callery and Miranda Smith
- JackK211424
-
500+ posts
I made a gamepad extension (SB2/SBX only)
content moved
Last edited by JackK211424 (July 29, 2019 16:24:55)
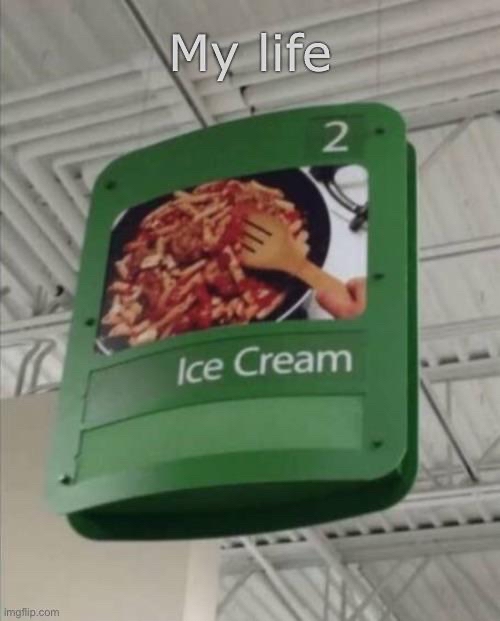
- Discussion Forums
- » Developing Scratch Extensions
-
» I made a gamepad extension (SB2/SBX only)