Discuss Scratch
- Discussion Forums
- » Help with Scripts
- » Identifying Clone-to-Clone Collisions
- EgraltSe
-
16 posts
Identifying Clone-to-Clone Collisions
Say we have a sprite with multiple clones. When one clone collides with another, we need some way of identifying the clones that were involved in the collision. We can detect a collision like this:
For example, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. Keep in mind that more than one collision may be happening at once, and we want to identify which clones are involved in each collision. A complex system of broadcasts and hiding could work, but it is slow and inefficient. Is there a better way?
<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. Keep in mind that more than one collision may be happening at once, and we want to identify which clones are involved in each collision. A complex system of broadcasts and hiding could work, but it is slow and inefficient. Is there a better way?
Last edited by EgraltSe (March 26, 2024 14:21:19)
Follow @EgraltSe for awesome projects!
- MineTurte
-
500+ posts
Identifying Clone-to-Clone Collisions
Pretty much what you said. Clone ID's. Maybe try something like so: Say we have a sprite with multiple clones. When one clone collides with another, or a separate sprite collides with one of the clones, we need some way of identifying the clone that was collided with. We can detect a collision like this:<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a physics simulation with moving clones. We can give each clone an “ID” variable, and use a list to keep track of the motion of each corresponding clone. When one clone collides with another, we can use the movement data to transfer force to each clone. What we need is a way to get the ID of the clone that we collided with.
Or, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. I would like to use clones of the same sprite, and allow for different shapes and colors in each clone. How can I achieve this?
when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ whatever] ?> then
set [ touchingWhichClone?] to [personal_ID]
end
end
This is very simplified but hopefully it helps you!
Last edited by MineTurte (March 26, 2024 14:11:41)
(pronouns are she/her). Advanced Alpha Game Studios Manager. Our website: https://aags2.w3spaces.com
This is JuniperActias. AKA Moth mommy. If I helped you out consider following Juni-Bug (my new account)!
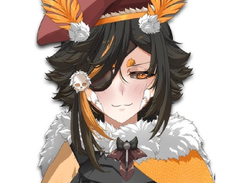
If you found this secret, say uwu
Signature gradients here
- EgraltSe
-
16 posts
Identifying Clone-to-Clone Collisions
This solves the problem of colliding with a separate sprite, but what about clone-to-clone collisions? I've edited the question so that the issue is clearer.Pretty much what you said. Clone ID's. Maybe try something like so: Say we have a sprite with multiple clones. When one clone collides with another, or a separate sprite collides with one of the clones, we need some way of identifying the clone that was collided with. We can detect a collision like this:<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a physics simulation with moving clones. We can give each clone an “ID” variable, and use a list to keep track of the motion of each corresponding clone. When one clone collides with another, we can use the movement data to transfer force to each clone. What we need is a way to get the ID of the clone that we collided with.
Or, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. I would like to use clones of the same sprite, and allow for different shapes and colors in each clone. How can I achieve this?when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ whatever] ?> then
set [ touchingWhichClone?] to [personal_ID]
end
end
This is very simplified but hopefully it helps you!
Last edited by EgraltSe (March 26, 2024 14:22:10)
Follow @EgraltSe for awesome projects!
- MineTurte
-
500+ posts
Identifying Clone-to-Clone Collisions
1. Make a random other sprite.This solves the problem of colliding with a separate sprite, but what about clone-to-clone collisions?Pretty much what you said. Clone ID's. Maybe try something like so: Say we have a sprite with multiple clones. When one clone collides with another, or a separate sprite collides with one of the clones, we need some way of identifying the clone that was collided with. We can detect a collision like this:<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a physics simulation with moving clones. We can give each clone an “ID” variable, and use a list to keep track of the motion of each corresponding clone. When one clone collides with another, we can use the movement data to transfer force to each clone. What we need is a way to get the ID of the clone that we collided with.
Or, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. I would like to use clones of the same sprite, and allow for different shapes and colors in each clone. How can I achieve this?when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ whatever] ?> then
set [ touchingWhichClone?] to [personal_ID]
end
end
This is very simplified but hopefully it helps you!
2. Grab the
<touching [ v] ?>block and set it to the sprite with the clones then drag it onto the sprite with the clones and drop it.
3. Go back into the clone sprite (you can delete the blank sprite you just made) and put the block into the clones like so:
when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ enemy] ?> then //in this case "enemy" is the sprite with all of the clones. Remember you get this block from the steps above here
set [ touchingWhichClone?] to [personal_ID]
end
end
Hope this helps!
Last edited by MineTurte (March 26, 2024 14:25:07)
(pronouns are she/her). Advanced Alpha Game Studios Manager. Our website: https://aags2.w3spaces.com
This is JuniperActias. AKA Moth mommy. If I helped you out consider following Juni-Bug (my new account)!
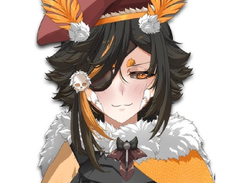
If you found this secret, say uwu
Signature gradients here
- EgraltSe
-
16 posts
Identifying Clone-to-Clone Collisions
Oh, that's clever. This certainly helps with two clones colliding, and will help a lot. What if we have four clones, colliding in pairs? How do we identify which clones were involved in each collision?1. Make a random other sprite.This solves the problem of colliding with a separate sprite, but what about clone-to-clone collisions?Pretty much what you said. Clone ID's. Maybe try something like so: Say we have a sprite with multiple clones. When one clone collides with another, or a separate sprite collides with one of the clones, we need some way of identifying the clone that was collided with. We can detect a collision like this:<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a physics simulation with moving clones. We can give each clone an “ID” variable, and use a list to keep track of the motion of each corresponding clone. When one clone collides with another, we can use the movement data to transfer force to each clone. What we need is a way to get the ID of the clone that we collided with.
Or, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. I would like to use clones of the same sprite, and allow for different shapes and colors in each clone. How can I achieve this?when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ whatever] ?> then
set [ touchingWhichClone?] to [personal_ID]
end
end
This is very simplified but hopefully it helps you!
2. Grab the<touching [ v] ?>block and set it to the sprite with the clones then drag it onto the sprite with the clones and drop it.
3. Go back into the clone sprite (you can delete the blank sprite you just made) and put the block into the clones like so:when green flag clicked
set [ cloneType] to [0]
repeat (3)
change [ cloneType] by (1)
create clone of [ myself]
end
when I start as a clone
set [ personal_ID] to (cloneType) //Set the variable personal_ID to a "This sprite only" variable when you make it.
forever
if <touching [ enemy] ?> then //in this case "enemy" is the sprite with all of the clones. Remember you get this block from the steps above here
set [ touchingWhichClone?] to [personal_ID]
end
end
Hope this helps!
I'm not sure that this is possible in Scratch. If it isn't, I could try to use different sprites. But I would like to avoid this because it can complicate things more. Thanks for your help!
Last edited by EgraltSe (March 26, 2024 14:32:58)
Follow @EgraltSe for awesome projects!
- grandpasp
-
36 posts
Identifying Clone-to-Clone Collisions
If you are still interested, I just shared my solution on my profile. Say we have a sprite with multiple clones. When one clone collides with another, we need some way of identifying the clones that were involved in the collision. We can detect a collision like this:<touching [ Cloned_Sprite] ?>However this gives us no way of knowing which specific clone is being collided with.
For example, say we have a game with different types of enemy clones. We need a way to identify what type of enemy we have collided with. Keep in mind that more than one collision may be happening at once, and we want to identify which clones are involved in each collision. A complex system of broadcasts and hiding could work, but it is slow and inefficient. Is there a better way?
- EgraltSe
-
16 posts
Identifying Clone-to-Clone Collisions
@grandpasp - Thanks!
Follow @EgraltSe for awesome projects!
- Discussion Forums
- » Help with Scripts
-
» Identifying Clone-to-Clone Collisions